https://github.com/phughesmcr/grid-neighbors-1d
Get the 8 directional neighbors of a cell in a 1d array
https://github.com/phughesmcr/grid-neighbors-1d
1d 2d array game-of-life grid javascript neighbors neighbours node
Last synced: about 1 month ago
JSON representation
Get the 8 directional neighbors of a cell in a 1d array
- Host: GitHub
- URL: https://github.com/phughesmcr/grid-neighbors-1d
- Owner: phughesmcr
- License: mit
- Created: 2019-04-02T15:21:00.000Z (about 6 years ago)
- Default Branch: master
- Last Pushed: 2023-11-01T07:11:11.000Z (over 1 year ago)
- Last Synced: 2025-03-26T23:51:15.959Z (about 2 months ago)
- Topics: 1d, 2d, array, game-of-life, grid, javascript, neighbors, neighbours, node
- Language: TypeScript
- Size: 201 KB
- Stars: 3
- Watchers: 1
- Forks: 1
- Open Issues: 5
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# grid-neighbors-1d
Get the 8 closest neighbors of a grid with edge wrapping from a 1d array
## Why?
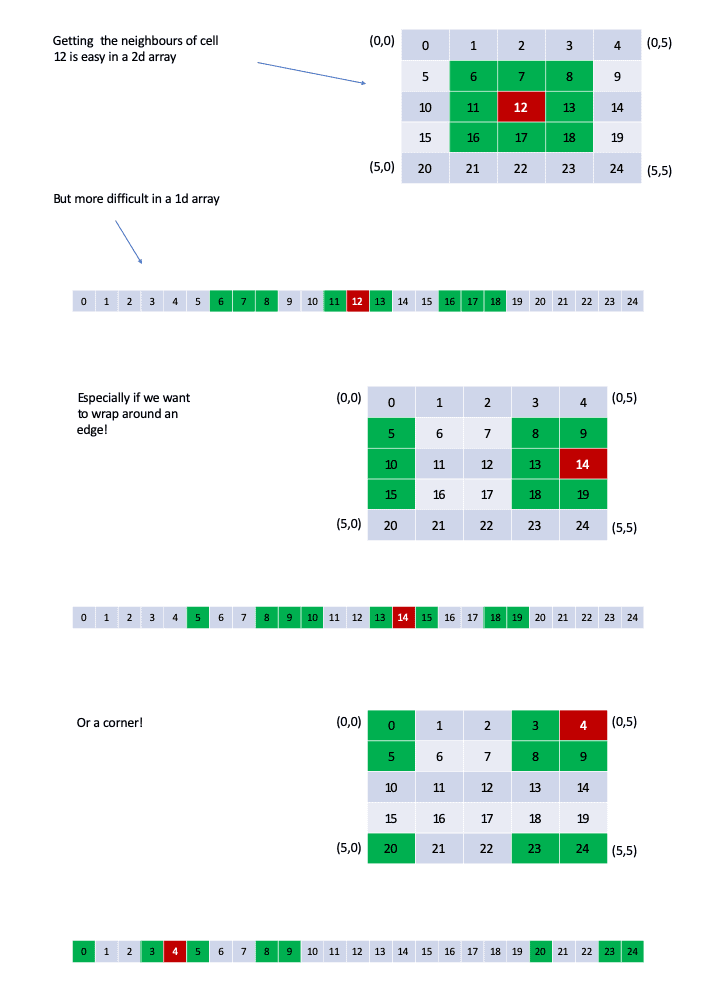## Usage
Get the neighbors of cell 12 in a 5x5 grid:
```javascript
import { getNeighbors } from "grid-neighbors-1d";
const neighbors = getNeighbors(12, 5, 5);
console.log(neighbors); // [7, 8, 13, 18, 17, 16, 11, 6] - clockwise from north
````getNeighbors` returns an array of indexes of the cell immediately to the north of the chosen cell, then clockwise around the remaining cells:
* neighbors[0] = north neighbor
* neighbors[1] = north-east neighbor
* neighbors[2] = east neighbor
* neighbors[3] = south-east neighbor
* neighbors[4] = south neighbor
* neighbors[5] = south-west neighbor
* neighbors[6] = west neighbor
* neighbors[7] = north-west neighborThis means you can use destructuring if your environment supports it:
```javascript
const [north, northEast, east, southEast, south, southWest, west, northWest] = getNeighbors(4, 10, 6);
```A `Direction` object is also available for convenience:
```javascript
import { Direction } from "grid-neighbors-1d";
const neighbors = getNeighbors(12, 5, 5);
console.log(neighbors[Direction.NORTH]); // 7
```If memory usage is not a concern and you are likely to call `getNeighbors` many times, you may want to precalculate the neighbors for a given grid size:
```javascript
import { generateNeighborLookup } from "grid-neighbors-1d";
const getNeighbors = generateNeighborLookup(5, 5);
const neighbors = getNeighbors(12);
console.log(neighbors); // [7, 8, 13, 18, 17, 16, 11, 6]
```For your convenience, TypeScript type declarations (index.d.ts), a declaration map (index.d.ts.map), and a sourcemap (index.js.map) are included.
## License
© 2019-23 [P. Hughes](https://www.phugh.es). All rights reserved.Shared under the [MIT](https://choosealicense.com/licenses/mit/) license.