https://github.com/raghavtilak/simpledragswiperv
Simple RecyclerViewAdapter library with Swipe to delete and Drag to Sort functionality. Supports all the three Layouts(Vertical, Horizontal, Grid).
https://github.com/raghavtilak/simpledragswiperv
android custom-recyclerview drag-swipe-recyclerview dragsort-recyclerview dragsortview hacktoberfest hacktoberfest-accepted hacktoberfest-android hacktoberfest2023 java recyclerview-adapter swipe-recyclerview
Last synced: about 1 month ago
JSON representation
Simple RecyclerViewAdapter library with Swipe to delete and Drag to Sort functionality. Supports all the three Layouts(Vertical, Horizontal, Grid).
- Host: GitHub
- URL: https://github.com/raghavtilak/simpledragswiperv
- Owner: raghavtilak
- Created: 2021-07-21T10:58:27.000Z (almost 4 years ago)
- Default Branch: master
- Last Pushed: 2023-11-11T17:54:49.000Z (over 1 year ago)
- Last Synced: 2025-03-25T15:49:10.623Z (about 2 months ago)
- Topics: android, custom-recyclerview, drag-swipe-recyclerview, dragsort-recyclerview, dragsortview, hacktoberfest, hacktoberfest-accepted, hacktoberfest-android, hacktoberfest2023, java, recyclerview-adapter, swipe-recyclerview
- Language: Kotlin
- Homepage:
- Size: 166 KB
- Stars: 3
- Watchers: 1
- Forks: 3
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# SimpleDragSwipeAdapter
Simple RecyclerView Adapter with Drag-Sort and Swipe Delete functionality.
[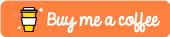](https://www.buymeacoffee.com/raghavtilak)[](https://shields.io/)
### Functionality #
1. Swipe UP/DOWN (Horizontal) Swipe RIGHT/LEFT (Vertical) to remove an item.
2. LongPress and Drag to sort items in RecyclerView.# Demo 📱 #
LinearLayout | HorizontalLayout | GridLayout
--- | --- | ---
||
|
# Usage 🛠️ #
## Dependency #
> Step 1. Add the JitPack repository to your build file
```
allprojects {
repositories {
...
maven { url 'https://jitpack.io' }
}
}
```>Step 2. Add the dependency
```
dependencies {
implementation 'com.github.raghavtilak:SimpleDragSwipeRV:1.0.0'
}
```
## Model Class #
```
public class UserModel {
private String name;
private String phone;
private String country;public UserModel(String name, String phone, String country) {
this.name = name;
this.phone = phone;
this.country = country;
}
....
}
```
## RecyclerView Adapter #
```
public class RVAdapter extends DragSwipeAdapter {public RVAdapter(@NonNull Context context, List datas, int itemLayoutId, RecyclerView recyclerView, boolean isCanDrag, boolean isCanSwipe, int displayMode, int itemSpacing, OnItemClick onItemClickListener) {
super(context, datas, itemLayoutId, recyclerView, isCanDrag, isCanSwipe, displayMode, itemSpacing, onItemClickListener);
}@Override
protected void bindView(UserModel item, DragSwipeAdapter.ViewHolder viewHolder) {
if(item!=null){
TextView name=(TextView)viewHolder.getView(R.id.textViewName);
TextView phone=(TextView)viewHolder.getView(R.id.textViewPhone);
TextView country=(TextView)viewHolder.getView(R.id.textViewCountry);
name.setText(item.getName());
phone.setText(item.getPhone());
country.setText(item.getCountry());
}
}@Override
public void onItemSwiped() {}
@Override
public void onItemMoved() {}
}
```
## OnItemClickListener #
```
public class MainActivity extends AppCompatActivity implements DragSwipeAdapter.OnItemClick {
...
@Override
public void onClick(View view, int position, UserModel item) {
...
}
...
}
```## Attach Adapter to RecyclerView
```
...
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);recyclerView=findViewById(R.id.recyclerView);
recyclerView.setLayoutManager(new LinearLayoutManager(this,LinearLayoutManager.VERTICAL,false));userList=new ArrayList<>();
loadData();
listAdapter = new RVAdapter(this,userList,R.layout.item_layout,
recyclerView,
true,false,RVAdapter.VERTICAL,
16,this);
}
...
```
# Constructor #
```
public DragSwipeAdapter(@NonNull Context context, List datas, int itemLayoutId,
RecyclerView recyclerView,
boolean isCanDrag, boolean isCanSwipe, int displayMode,
int itemSpacing, OnItemClick onItemClickListener)
```