https://github.com/revelcw/react-hooks-helper
A custom React Hooks library that gives you custom hooks for your code.
https://github.com/revelcw/react-hooks-helper
custom-hook form hooks react react-hooks step wizard wizard-steps
Last synced: 2 months ago
JSON representation
A custom React Hooks library that gives you custom hooks for your code.
- Host: GitHub
- URL: https://github.com/revelcw/react-hooks-helper
- Owner: revelcw
- License: mit
- Archived: true
- Created: 2018-12-21T14:42:13.000Z (over 6 years ago)
- Default Branch: develop
- Last Pushed: 2023-01-03T15:47:13.000Z (over 2 years ago)
- Last Synced: 2024-09-17T01:41:46.796Z (9 months ago)
- Topics: custom-hook, form, hooks, react, react-hooks, step, wizard, wizard-steps
- Language: JavaScript
- Homepage:
- Size: 1.68 MB
- Stars: 244
- Watchers: 7
- Forks: 17
- Open Issues: 22
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
- awesome - react-hooks-helper - A custom React Hooks library that gives you custom hooks for your code. (JavaScript)
README
# react-hooks-helper
[](#contributors)
A custom [React Hooks](https://reactjs.org/docs/hooks-overview.html) library that gives you custom
hooks for your code.[](https://badge.fury.io/js/react-hooks-helper)
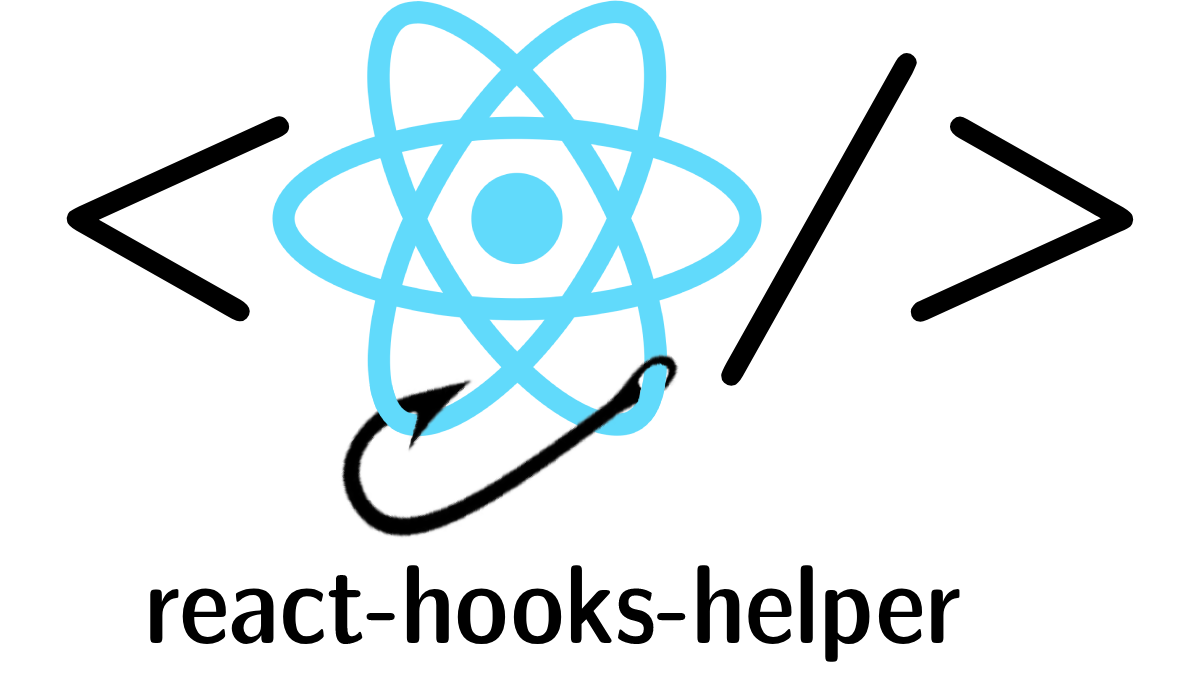
> π§β `useStep` is a multi-purpose step wizard. Build an image carousel!
>
> π `useForm` for dead simple form control with nested object support.
>
> π¦ `useTrafficLight` easily build a fun traffic light component.
>
> βΌ `useNot` to simplify toggling `true` / `false` without lambda functions.
>
> π Full 100% test coverage!
>
> π₯ Blazing fast!## Requirement β οΈ
To use `react-hooks-helper`, you must use `[email protected]`.
## Installation
```sh
$ npm i react-hooks-helper
```## Usage
```js
const { isPaused, index, step, navigation } = useStep(config);
const [{ foo, bar }, setForm] = useForm({ foo, bar });
const currentValue = useTrafficLight(initialIndex, durations);
const [bar, notBar] = useNot(bool);
```## Examples
### useStep
The new `useStep` Hook is the new `useTrafficLight` and is a more general step wizard.
You can use it to simplify many tasks, such as a multi-page input form, or an image carousel.It has an auto advance function, or control manually by calling `previous` and/or `next`.
#### Usage
```js
const { isPaused, index, step, navigation } = useStep(config);
```#### Config
You pass `useStep` a configuration object containing the following (\* = required).
| Key | Description |
| :-------------------- | :-------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| `steps`\* | Either an array containing the steps to process or an integer specifying the number of steps. |
| `initialStep` | The starting stepβeither a string id or an index. Default = 0. |
| `autoAdvanceDuration` | If you wish the steps to auto-advance, specify the number of milliseconds. You can also include an `autoAdvanceDuration` in each `step` in your `steps` array, if you wish to have different durations for each step. |#### Return object
| Key | Description |
| :-------------------- | :------------------------------------------------ |
| `index` | A number containing the current step index. |
| `step` | The current `step` object from the `steps` array. |
| `navigation` | A `navigation` object (see below). |
| `isPaused` | `true` if the `autoAdvanceDuration` is paused. |
| `autoAdvanceDuration` | Duration of the current auto-advance. |#### Navigation object
The `navigation` object returned from `useStep` contains control callback functions as follows.
| Key | Description |
| :--------- | :----------------------------------------------------------------------------------------------------- |
| `previous` | Call to navigate to the previous item index. Wraps from the first item to the last item. |
| `next` | Call to navigate to the next item index. Wraps from the last item to the first item. |
| `go` | Call to navigate to a specific step by `id` or by `index`. Example: `go(2)` or `go('billing-address')` |
| `pause` | Pause auto-advance navigation. |
| `play` | Play auto-advance navigation once it has been paused. |#### Example
There's a simple multi-step control with 3 "pages".
You use the "Previous" and "Next" buttons to navigate.```js
function App() {
const {
index,
navigation: { previous, next },
} = useStep({ steps: 3 });
return (
Hello CodeSandbox
{index === 0 &&
This is step 1}
{index === 1 &&This is step 2}
{index === 2 &&This is step 3}
Previous
Next
);
}
```#### Live demo
You can view/edit a photo carousel on CodeSandbox.
It automatically advances after 5 seconds. You can also click previous/next, or
navigate directly to a particular image.[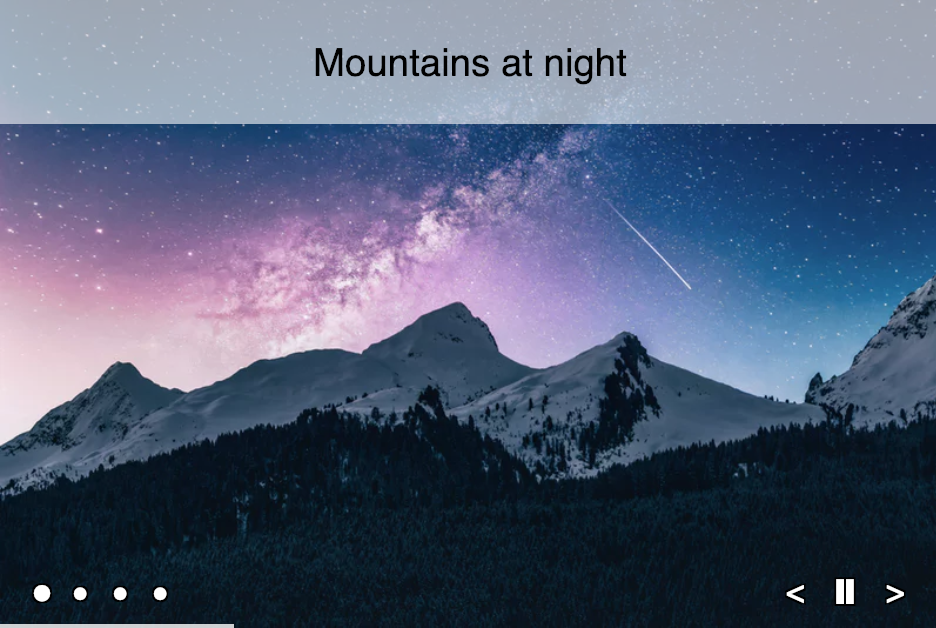](https://codesandbox.io/s/31228l0rnm)
[](https://codesandbox.io/s/31228l0rnm)
### useForm
`useForm` is for an advanced search, sign-up form, etc, something with a lot of text felds, because
you only need to use one hook. Wereas on the otherwise you would need many `useState` hooks.---
#### Before
Right here is some code for a sign-up form. As you can see it is using two `useState` hooks and we
need a lambda function to change it.```jsx
function App() {
const [firstName, setFirstName] = useState("");
const [lastName, setLastName] = useState("");
const [gender, setGender] = useState("Male");
const [isAccept, setAcceptToC] = useState(false);
return (
{
setFirstName(ev.target.value);
}}
/>
{firstName}
{
setLastName(ev.target.value);
}}
/>
{lastName}
{
setGender(ev.target.value);
}}
/>{" "}
Female
{
setGender(ev.target.value);
}}
/>{" "}
Male
Selected Gender: {gender}
{
setAcceptToC(ev.target.checked);
}}
/>{" "}
I accept and agree Terms & Conditions.
);
}
```#### After
```jsx
function App() {
const [{ firstName, lastName, gender, isAccept }, setValue] = useForm({
firstName: "",
lastName: "",
gender: "Male",
isAccept: false,
});
return (
{firstName}
{lastName}
{" "}
Female
{" "}
Male
Selected Gender: {gender}
{" "}
I accept and agree Terms & Conditions.
);
}
```You see `useForm` takes the name of your `input` and changes the object, so you only have to create
one `useForm`. You can have as many items in the object, and this allows many inputs, but with still
**_one_** `useForm`. And it eliminates the use of a lambda function.#### Nest objects
`useForm` also supports nested objects. This is useful for things like `billing.city` and `shipping.city`.
In your markup, you simply add the dots in the `name` field like this.
```html
```
#### Live demo
[](https://codesandbox.io/s/useform-rhh-demo-4jy0pxxxo0)
---
#### Before
```jsx
const lightDurations = [5000, 4000, 1000];const BeforeTrafficLight = ({ initialColor }) => {
const [colorIndex, setColorIndex] = useState(initialColor);useEffect(() => {
const timer = setTimeout(() => {
setColorIndex((colorIndex + 1) % 3);
}, lightDurations[colorIndex]);
return () => clearTimeout(timer);
}, [colorIndex]);return (
);
};
```#### After
```jsx
const AfterTrafficLight = ({ initialColor }) => {
const colorIndex = useTrafficLight(initialColor, [5000, 4000, 1000]);return (
);
};
```#### Live demo
[](https://codesandbox.io/s/zqo981j4ym)
### useNot
`useNot` is a toggle function for React components.
---
Here is a simple App that toggles a value to produce either a **blue** or a **red** square.
```jsx
function App() {
const [value, setValue] = useState(false);
return (
(
setValue( !value )
)}
style={{
width: 100,
height: 100,
backgroundColor: value ? 'red' : 'blue'
}}
/>
);
```#### After
```jsx
function App() {
const [value, notValue] = useNot(false);
return (
);
}
````value`, a boolean, is a variable. `notValue`function that nots the value from `true` to `false` and
vise versa. Notice the `notValue` is not a lambda function, like in the **[before](#useNotBefore)**#### Live demo
[](https://codesandbox.io/s/rwk86po7rn)
## My Coding Journey
On Dec 18, 2017, I did a talk at [ReactNYC](https://www.meetup.com/ReactNYC/) about the
`useTrafficLight` code above, but it was the "before" code and did not use a custom hook, and
_certainly_ not `react-hooks-helper` because it was not out yet!Here's my video.
[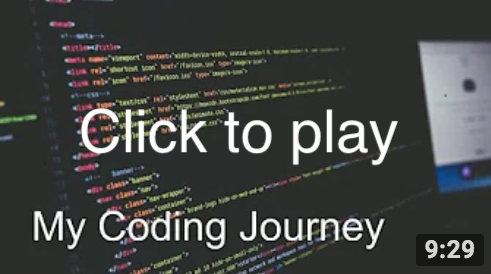](https://www.youtube.com/watch?v=mbiryVTIJ4Q&t=3s)
## License
**[MIT](LICENSE)** Licensed
## Code in the wild
Have you built an app (real or sample) using `react-hooks-helper`? Make a PR and add it to the list below.
- [Multi-step form demo](https://codesandbox.io/s/github/donavon/use-step-multi-step-form-demo)
## Contributors
Thanks goes to these wonderful people ([emoji key](https://github.com/all-contributors/all-contributors#emoji-key)):
Revel Carlberg West
π β οΈ π‘ π€ π§ π π§ π»
Donavon West
π» π€ β οΈ
Sunil Pai
π
Permadi Wibisono
π»
Jang Rush
π
This project follows the [all-contributors](https://github.com/all-contributors/all-contributors) specification. Contributions of any kind welcome!