Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/richytong/arche
HTML as JavaScript
https://github.com/richytong/arche
arche atom buildless component dom element es6 esm html javascript jsx-less react reactjs
Last synced: 3 months ago
JSON representation
HTML as JavaScript
- Host: GitHub
- URL: https://github.com/richytong/arche
- Owner: richytong
- License: mit
- Created: 2020-06-19T20:37:15.000Z (over 4 years ago)
- Default Branch: master
- Last Pushed: 2024-10-24T17:36:38.000Z (4 months ago)
- Last Synced: 2024-11-10T07:08:05.067Z (3 months ago)
- Topics: arche, atom, buildless, component, dom, element, es6, esm, html, javascript, jsx-less, react, reactjs
- Language: JavaScript
- Homepage:
- Size: 80.1 KB
- Stars: 6
- Watchers: 2
- Forks: 2
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- License: LICENSE
Awesome Lists containing this project
README
# Arche
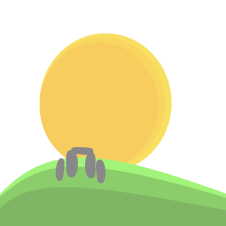
> Arche (/ˈɑːrki/; Ancient Greek: ἀρχή) is a Greek word with primary senses "beginning", "origin" or "source of action" (ἐξ' ἀρχῆς: from the beginning, οr ἐξ' ἀρχῆς λόγος: the original argument), and later "first principle" or "element". ([wikipedia](https://en.wikipedia.org/wiki/Arche))
[](https://codecov.io/gh/richytong/arche)HTML as JavaScript.
```javascript [playground]
const ReactElement = Arche(React)
// supply the React libraryconst { Div, H1, P } = ReactElement
// some common building blocks are provided on ReactElement
// as property functions.const myElement = Div([
H1('I am a heading'),
P('heyo'),
P('lorem ipsum'),
])render(myElement)
//
//I am a heading
//heyo
//lorem ipsum
//
```Create dynamic components with props:
```javascript [playground]
const ReactElement = Arche(React)
const { Div, H1, P, Button, Img } = ReactElementconst UserCard = ReactElement(({
firstName, lastName, age,
}) => Div([
H1(`${firstName} ${lastName}`),
Img({ src: 'https://via.placeholder.com/150x150', alt: 'placeholder' }),
P({ style: { color: 'lightgrey' } }, `age: ${age}`),
]))render(UserCard({ firstName: 'George', lastName: 'Henry', age: 32 }))
//
//George Henry
//![]()
//age: 32
//
```Complete interoperability with React hooks (converted from [this example](https://reactjs.org/docs/hooks-intro.html)):
```javascript [playground]
const ReactElement = Arche(React)
const { Div, P, Button } = ReactElement
const { useState } = Reactconst Example = ReactElement(() => {
const [count, setCount] = useState(0)return Div([
P(`You clicked ${count} times`),
Button({
onClick() {
setCount(count + 1)
},
}, 'Click me'),
])
})render(Example())
//
//You clicked {count} times
// Click me
//
```# Installation
with `npm`
```bash
npm i arche
```browser script, global `Arche`
```html```
browser module
```javascript
import Arche from 'https://unpkg.com/arche/es.js'
```# Syntax
```coffeescript [specscript]
Arche(React {
createElement: (type, props?, children?)=>ReactElement,
}, options? {
styled?: {
div: function,
p: funcion,
span: function,
// etc
},
styledMemoizationCap?: number, // defaults to 1000
}) -> ReactElement
```# Usage
Set Arche elements globally for a great developer experience.
```javascript
// global.jsconst ReactElement = Arche(React)
window.ReactElement = ReactElement
for (const elementName in ReactElement) {
window[elementName] = ReactElement[elementName]
}// Arche for now does not export every element
// create the ones you need like so
window.Aside = ReactElement('aside')
window.Svg = ReactElement('svg')
window.Path = ReactElement('path')
```## Using styled
Arche accepts a `styled` option from css-in-js libraries like [Styled Components](https://styled-components.com/) to enable a `css` prop on the base elements. This does not apply to composite components (those created with `ReactElement(props => {...})` syntax)```javascript
// global.js
const ReactElement = Arche(React, { styled })
```Elements can now specify a `css` prop to use css-in-js.
```javascript
// MyComponent.js
const MyComponent = ReactElement(props => {
return Div({
css: `
width: 500px;
background-color: pink;
`,
})
})
```## Using React Context
To use React Context with Arche, wrap `YourContext.Provider` with `ReactElement` and supply `value` as a prop, specifying children in the next argument.JSX example:
```javascript
function ArticleWrapper () {
const [theme, setTheme] = React.useState(themes[0])return (
)
}
```Translates to the following with Arche:
```javascript
const ArticleWrapper = ReactElement(() => {
const [theme, setTheme] = React.useState(themes[0])return ReactElement(ThemeContext.Provider)({
value: { theme, changeTheme: setTheme },
}, [ThemeSwitcher(), Article()])
})
```