Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/sandysanthosh/springboot
Spring Boot Programs
https://github.com/sandysanthosh/springboot
mongodb postman restful-api spring springboot thymleaf
Last synced: about 2 months ago
JSON representation
Spring Boot Programs
- Host: GitHub
- URL: https://github.com/sandysanthosh/springboot
- Owner: sandysanthosh
- Created: 2020-04-05T16:06:27.000Z (over 4 years ago)
- Default Branch: master
- Last Pushed: 2022-11-24T06:41:36.000Z (about 2 years ago)
- Last Synced: 2023-03-04T17:47:25.717Z (almost 2 years ago)
- Topics: mongodb, postman, restful-api, spring, springboot, thymleaf
- Language: Java
- Homepage:
- Size: 5.17 MB
- Stars: 0
- Watchers: 1
- Forks: 0
- Open Issues: 1
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# SpringBoot
Spring Boot Programs
# SpringBoot
* Spring Boot Programs
* Spring Boot DATAJPA
* Spring Boot MONGODB
### Run Programs in POSTMAN: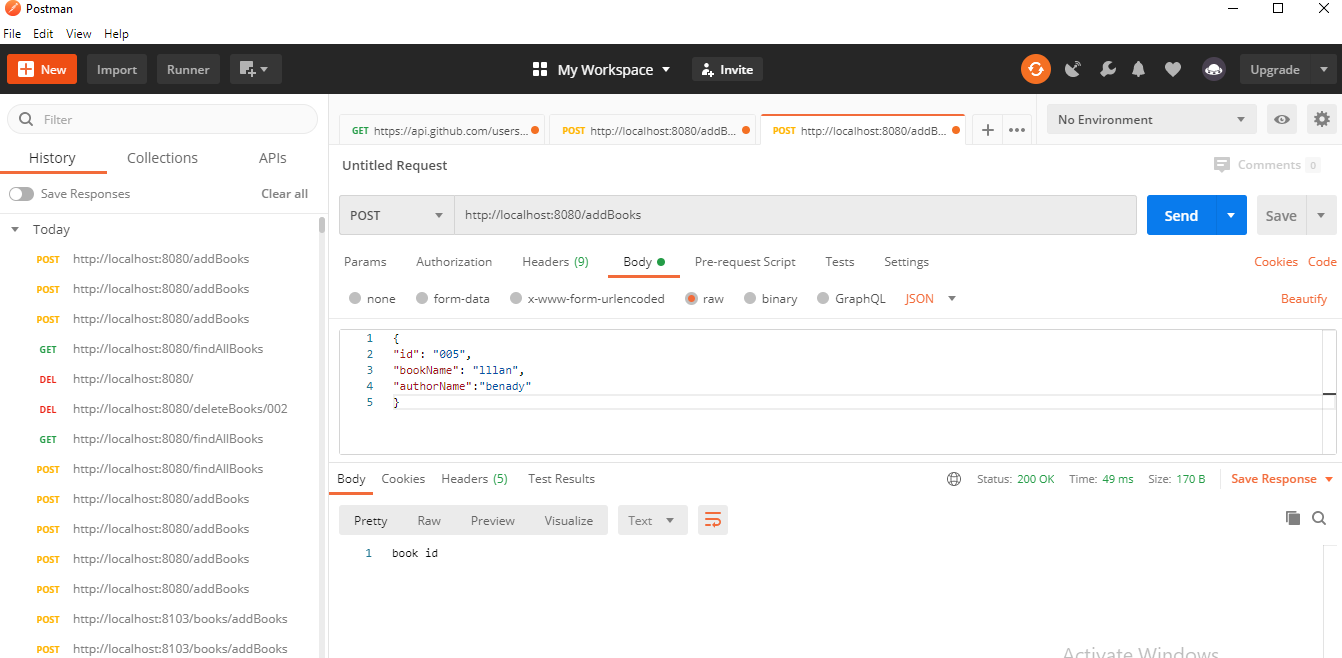
### RUN in MongoDB Compass:
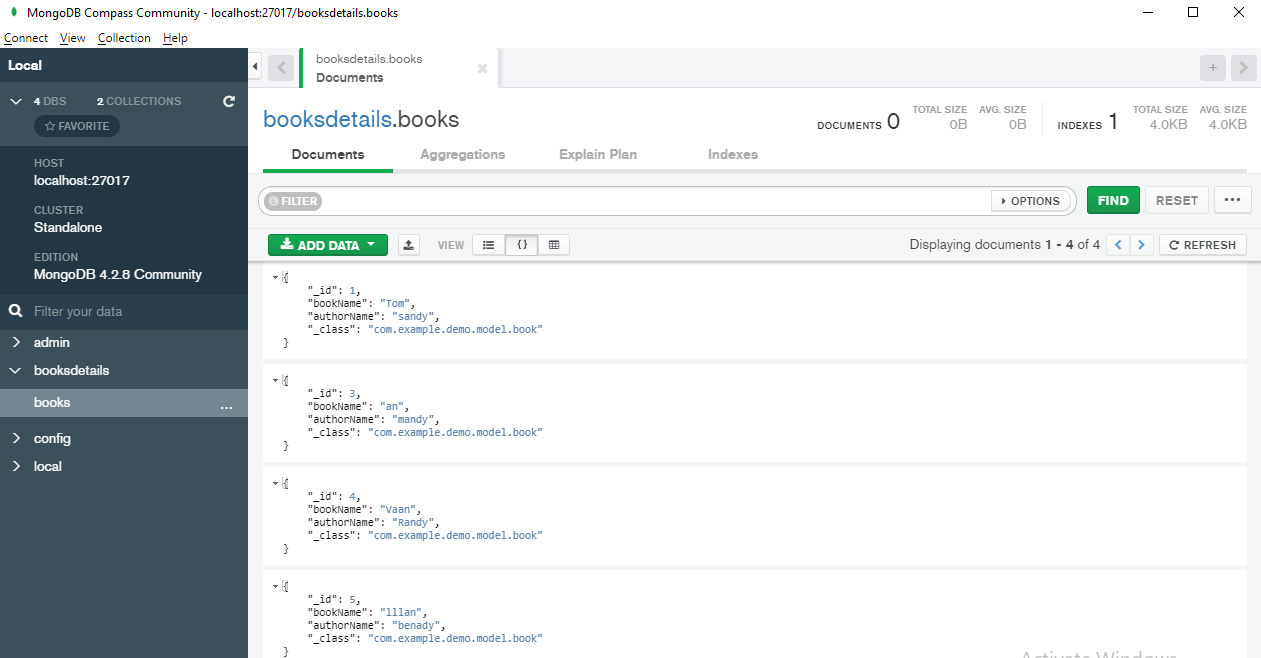
#### MOngoDB Tables:
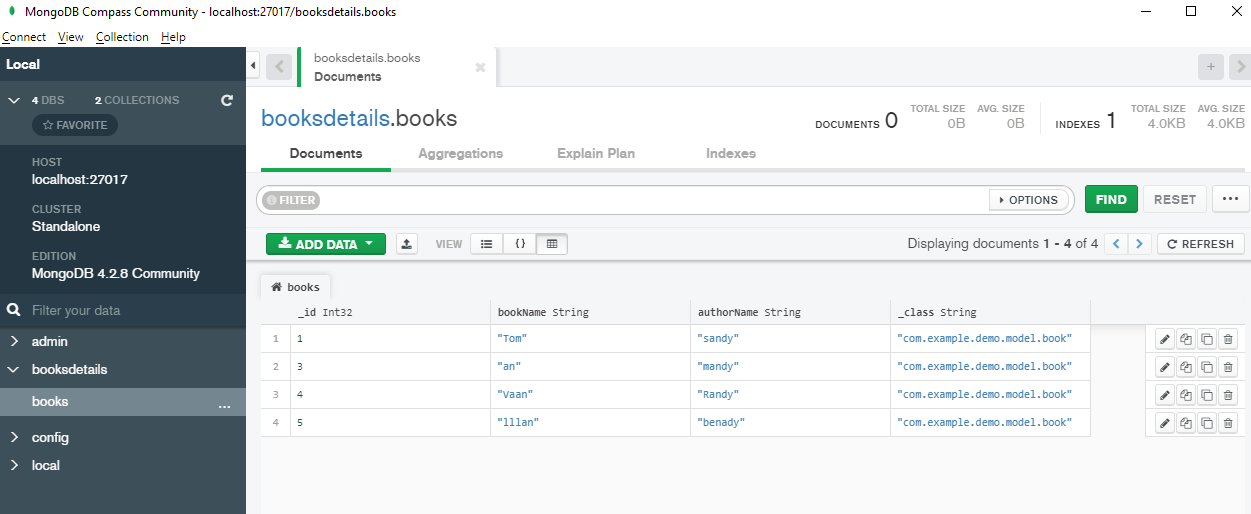
### Book.java:
```
package com.example.demo.model;
import org.springframework.data.annotation.Id;
import org.springframework.data.mongodb.core.mapping.Document;import lombok.Data;
@Data
@Document(collection="books")
public class book {
@Id
private int id;
private String bookName;
private String authorName;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getBookName() {
return bookName;
}
public void setBookName(String bookName) {
this.bookName = bookName;
}
public String getAuthorName() {
return authorName;
}
public void setAuthorName(String authorName) {
this.authorName = authorName;
}
}
```#### BookRepository.Java:
```
package com.example.demo.repository;
import org.springframework.data.mongodb.repository.MongoRepository;
import org.springframework.stereotype.Repository;import com.example.demo.model.book;
@Repository
public interface BookRepository extends MongoRepository{}
```
#### BookController.java:
```
package com.example.demo.Controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;import com.example.demo.model.book;
import com.example.demo.repository.BookRepository;@RestController
public class BookController {
@Autowired
private BookRepository BookRepository;
@GetMapping("/findAllBooks")
public List getAllBooks()
{
return BookRepository.findAll();
}
@PostMapping("/addBooks")
public String addAllBooks(@RequestBody book book)
{
BookRepository.save(book);
return "book id";
}
@DeleteMapping("/deleteBooks/{id}")
public String deleteBooks(@PathVariable int id)
{
BookRepository.deleteById(id);
return "book id";
}
@GetMapping("/findBook/{id}")
public String getAllBooksById(@PathVariable int id)
{
BookRepository.findById(id);
return "book id";
}
}```
#### Application.properties:
```
spring.data.mongodb.database=booksdetails
spring.data.mongodb.port=27017
spring.data.mongodb.host=localhost```