Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/sergiosanchezs/react-validation
This is a repository of an article explaining how to use reac-hook-form library with material-ui.
https://github.com/sergiosanchezs/react-validation
create-react-app javascript material-ui reac-hooks react react-hook-form
Last synced: 6 days ago
JSON representation
This is a repository of an article explaining how to use reac-hook-form library with material-ui.
- Host: GitHub
- URL: https://github.com/sergiosanchezs/react-validation
- Owner: sergiosanchezs
- Created: 2020-08-27T00:36:28.000Z (over 4 years ago)
- Default Branch: master
- Last Pushed: 2020-09-08T23:43:21.000Z (over 4 years ago)
- Last Synced: 2024-11-08T15:23:14.548Z (about 2 months ago)
- Topics: create-react-app, javascript, material-ui, reac-hooks, react, react-hook-form
- Language: JavaScript
- Homepage:
- Size: 408 KB
- Stars: 1
- Watchers: 2
- Forks: 2
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Ultimate Form Validation in React with the awesome React Hook Form and Material-UI Libraries!
#React
#ReactHookForm
#Material-UI
#React-Hooks
#JavaScript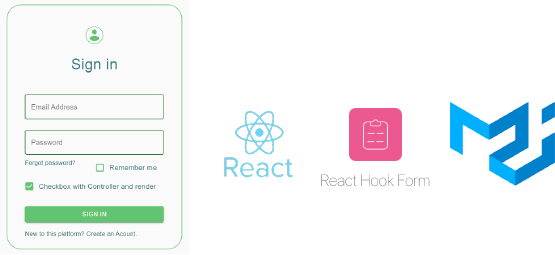
This article describes in a sneak peek what is the library [react-hook-form](#react-hook-form-install) and how to use it. First of all, you need to set up the environment by creating a new react application with the command create-react-app. If you don't know how to do it, please refer to my post [Setting up the react environment with the super tool create-react-app](https://dev.to/sergiosanchezs/setting-up-the-react-environment-with-the-super-tool-creat-react-app-5hja) before continuing in this tutorial.
## Content Table
1. [Installing libraries locally](#lib-local)
- [Installing React Hook Form Package](#react-hook-form-install)
- [Installing Material UI](#material-ui-install).
2. [Creating the Needing Files](#creating-files).
3. [Setting up the App.js](#setup-app).
4. [Starting the application](#start-app).
5. [Working on the design of SignInForm](#design-form).
- [styles.js File](#style-file).
- [SignInForm Component](#SignInForm-Component).
6. [Working with React-Hook-Form to Validate the data](#working-react-hook-form-validation).- [Email Field](#email-field).
- [Password Field](#password-field).
- [Checkbox](#checkbox).
- [Option 1 - Register](#check-op1).
- [Option 2 - Controller and render](#check-op2).7. [DevTools on React-Hook-Form](#devtools).
8. [References](#references).## **Installing libraries locally**
We need to install the npm modules necessary to build this project which means it will be saved as a dependency in our `package.json` file.
We are going to install _material-ui_ and _react-hook-form_ library locally.
#### Installing React Hook Form Package
To install the `react-hook-form` module we have to type the following command on the terminal located in the project directory:
```
yarn add [email protected]
```I've installed the version _6.5.3_.
To install the `material-ui` module to give some styling to our example form, we have to type the following command:
```
yarn add @material-ui/[email protected]
yarn add @material-ui/[email protected]
```This commands are for people working on a MAC or Linux Machine, If you are on a windows you can install an emulator to type the commands as you were in a Linux Machine. [Click here to download Hyper](https://hyper.is/)
```
mkdir components
touch components/SignInForm.js
touch styles.js
```In the App.js copy the following code or import and add the SignInForm component:
```javascript
import React from 'react';
import SignInForm from './components/SignInForm';const App = () => (
);export default App;
```You can start the application as you see in the tutorial in the introduction of this article with the following command:
```
yarn run start
```## Working on the design of SignInForm
In this file, we are gonna change some classes, colours and styles of the final design of our Sign In Form based on the `material-ui` library.
```javascript
import TextField from '@material-ui/core/TextField';
import { makeStyles, withStyles } from '@material-ui/core/styles';const mingColor = '#387780';
const dartmouthGreenColor = '#2D7638';
const emeraldGreenColor = '#62C370';export const CssTextField = withStyles({
root: {
'& label.Mui-focused': {
color: mingColor,
},
'& .MuiInput-underline:after': {
borderBottomColor: dartmouthGreenColor,
},
'&$checked': {
color: '#3D70B2',
},
'& .MuiOutlinedInput-root': {
'& fieldset': {
borderColor: dartmouthGreenColor,
},
'&:hover fieldset': {
borderColor: emeraldGreenColor,
},
'&.Mui-focused fieldset': {
borderColor: mingColor,
},
},
},
})(TextField);export const useStyles = makeStyles(theme => {
return {
paper: {
margin: theme.spacing(4, 0),
display: 'flex',
color: '#387780',
flexDirection: 'column',
alignItems: 'center',
border: `1px solid ${emeraldGreenColor}`,
borderRadius: '2rem',
padding: '1.5rem 2.5rem',
},
avatar: {
margin: theme.spacing(3),
backgroundColor: emeraldGreenColor,
fontSize: 50,
},
form: {
marginTop: theme.spacing(4),
width: '100%',
},
submit: {
margin: theme.spacing(3, 0, 2),
backgroundColor: emeraldGreenColor,
color: 'white',
padding: '50 50',
},
link: {
color: mingColor,
textDecoration: 'none !important',
},
checkBox: {
color: `${emeraldGreenColor} !important`,
},
error: {
color: 'red',
},
};
});
```This is the component with the styles defined in the previous file applied and using the `material-ui` library for final design.
```javascript
import React from 'react';
import { AccountCircle as AccountCircleIcon } from '@material-ui/icons';
import {
Avatar,
Grid,
Container,
CssBaseline,
FormControlLabel,
Button,
Link,
Checkbox,
Typography,
} from '@material-ui/core';
import { CssTextField, useStyles } from '../styles';const SignInForm = () => {
const classes = useStyles();const onSubmit = e => {
e.preventDefault();
console.log(e.target);
};return (
Sign in
onSubmit(e)}>
Forgot password?
}
/>
Sign In
{'New to this platform? Create an Acount.'}
);
};export default SignInForm;
```## Working with React-Hook-Form to Validate the data
Here we are going to explain some tweaks to be able to validate the data in the `SignInForm` component.
First, we need to import `useForm` hook and the `Controller` component from the library:
```javascript
import { useForm, Controller } from 'react-hook-form';
```After, initializing the styles in the `SignInForm` component we are going to add the `register` and `handleSubmit` functions, and `errors` and `control` objects:
```javascript
const { register, handleSubmit, errors, control } = useForm();
```However, If you want to configure the `useForm` hook more, you can add an object with the details that you want to specify:
```javascript
// ...
const { register, handleSubmit, control, errors } = useForm({
mode: 'onChange',
reValidateMode: 'onChange',
defaultValues: {
email: '',
password: '',
remember: false,
},
});
// ...
```We are gonna update our `onSubmit` function and integrate it with `handleSubmit` hook from `useFrom` inside the form tag:
```javascript
// ...
const onSubmit = data => alert(JSON.stringify(data));
// ...
// ...
```In order to add each input field to the form data, we just need to reference the `register` function in each component. For example, in an `input`, we add the property ref. However, we are using `material-ui`, so, to do the reference to the register function we use the property `inputRef` instead. See the example below:
```javascript
// ...// ...
```We can add an object to the register function to be able to set up different functionalities. For instance, in the email, we want the email to be `required` and personalize the `message` that the user will see if the email field is blank. Additionally, we want the email to follow a certain `pattern` that every email has, so we are going to use a regex expression to do this and set up a `message` if the email doesn´t follow the pattern established by this expression. We add an error property to the `CssTextField` that this changes the colour to red if there is an error message to show about this field.
```javascript
// ...
()[\]\\.,;:\s@\"]+(\.[^<>()[\]\\.,;:\s@\"]+)*)|(\".+\"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/,
message: 'You must provide a valid email address!',
},
})}
autoComplete='email'
error={!!errors.email}
className={classes.margin}
fullWidth
autoFocus
/>
// ...
```Now, we want that if occurs any of the errors aforementioned (required field and pattern) to show the message to the user. We use this code after the previous component:
```javascript
{
errors.email && {errors.email.message};
}
// ...
```In the password field we are going to set up as a required field and as the minimum length of 6 characters. The component with the register function and the error message display section will be like this:
```javascript
// ...
;
{
errors.password && (
{errors.password.message}
);
}
// ...
```For `Checkbox` component, there are two options. However, I prefer the second one which the defaultValue updates the initial state of the checkbox. This are the options:
This option is using the register function like in the previous components.
```javascript
// ...
}
/>
// ...
```##### Option 2 - Controller and render
In this option, we need to change the Checkbox to Controller inside the control property to be able to expose to register this field in the form data. In the `Controller` component we add the `control={control}` we put a `defaultValue` right in line, and add the render property to set up the onChange event and checked value.
After the previous grid container, we add another one to add the `checkTest` checkbox. You can see the component added into the code below:
```javascript
// ...
(
onChange(e.target.checked)}
checked={value}
/>
)}
/>
}
label='Checkbox with Controller and render'
/>
1. To install the devtools in the dev dependencies package in the project executing the following command:
```
yarn add @hookform/[email protected] -D
```The latest version at this time is `2.2.1`.
2. import the `DevTool` in the `SignInForm` Component:
```javascript
import { DevTool } from '@hookform/devtools';
```3. We have already the control object in the useForm declaration:
```javascript
const { register, handleSubmit, watch, control, errors } = useForm();
```4. After the Container component we add the DevTool:
```javascript
// ...
return (
// ...
```That's it. You will see the dev tools on the browser as soon as it renders again after you save the file.
1. [Get Started Guide of react-hook-form](https://react-hook-form.com/get-started)
2. [API of react-hook-form](https://react-hook-form.com/api/)
3. [Material UI Components](https://material-ui.com/components/)
4. [Example Project on Github](https://github.com/sergiosanchezs/react-validation)