https://github.com/servicestack/servicestack.swift
Swift support used in ServiceStack
https://github.com/servicestack/servicestack.swift
generic service-client servicestack servicestack-reference servicestack-xcode-plugin swift typed
Last synced: about 2 months ago
JSON representation
Swift support used in ServiceStack
- Host: GitHub
- URL: https://github.com/servicestack/servicestack.swift
- Owner: ServiceStack
- License: other
- Created: 2015-01-17T22:30:29.000Z (over 10 years ago)
- Default Branch: master
- Last Pushed: 2024-11-11T03:21:31.000Z (8 months ago)
- Last Synced: 2024-11-11T04:25:40.153Z (8 months ago)
- Topics: generic, service-client, servicestack, servicestack-reference, servicestack-xcode-plugin, swift, typed
- Language: Swift
- Homepage:
- Size: 11.7 MB
- Stars: 9
- Watchers: 8
- Forks: 9
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: license.txt
Awesome Lists containing this project
README
Follow [@ServiceStack](https://twitter.com/servicestack) or join the [Google+ Community](https://plus.google.com/communities/112445368900682590445)
for updates, or [StackOverflow](http://stackoverflow.com/questions/ask) or the [Customer Forums](https://forums.servicestack.net/) for support.# ServiceStack.Swift
See [Swift Add ServiceStack Reference](http://docs.servicestack.net/swift-add-servicestack-reference) for an overview of the Swift Support in ServiceStack.
ServiceStack's **Add ServiceStack Reference** feature lets iOS/macOS developers easily generate an native
typed Swift API for your ServiceStack Services using the `x` dotnet command-line tool.## Simple command-line utils for ServiceStack
The [x dotnet tool](https://docs.servicestack.net/dotnet-tool) provides a simple command-line UX to easily Add and Update Swift ServiceStack References.
Prerequisites: Install [.NET Core](https://dotnet.microsoft.com/download).
$ dotnet tool install --global x
This will make the `x` dotnet tool available in your `$PATH` which can now be used from within a **Terminal window** at your Xcode project folder.
To use the latest `JsonServiceClient` you'll need to add a reference to ServiceStack Swift library using your preferred package manager:
### Xcode
From Xcode 12 the Swift Package Manager is built into Xcode.
Go to **File** > **Swift Packages** > **Add Package Dependency**:
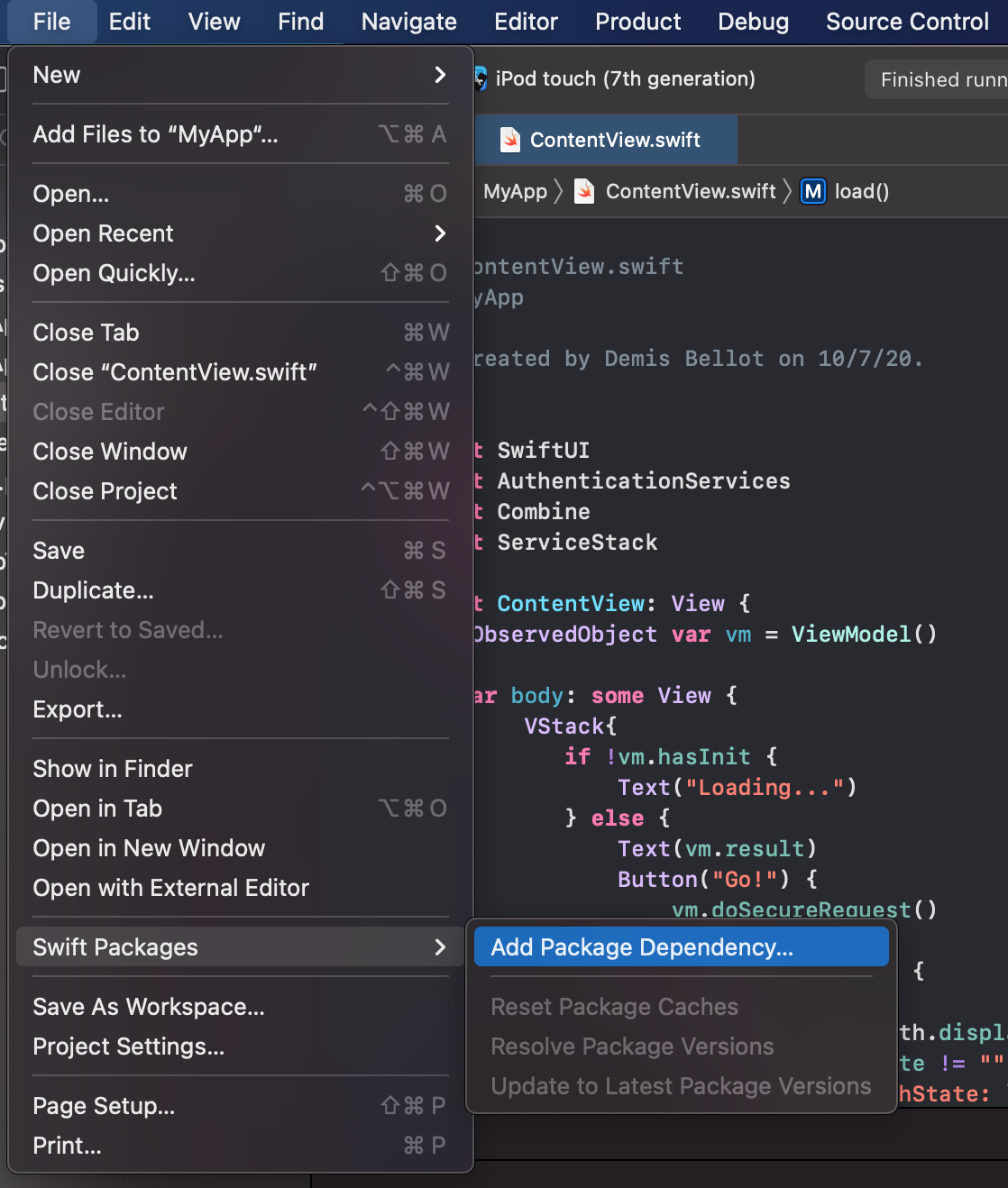
Add a reference to the ServiceStack.Swift GitHub repo:
https://github.com/ServiceStack/ServiceStack.Swift
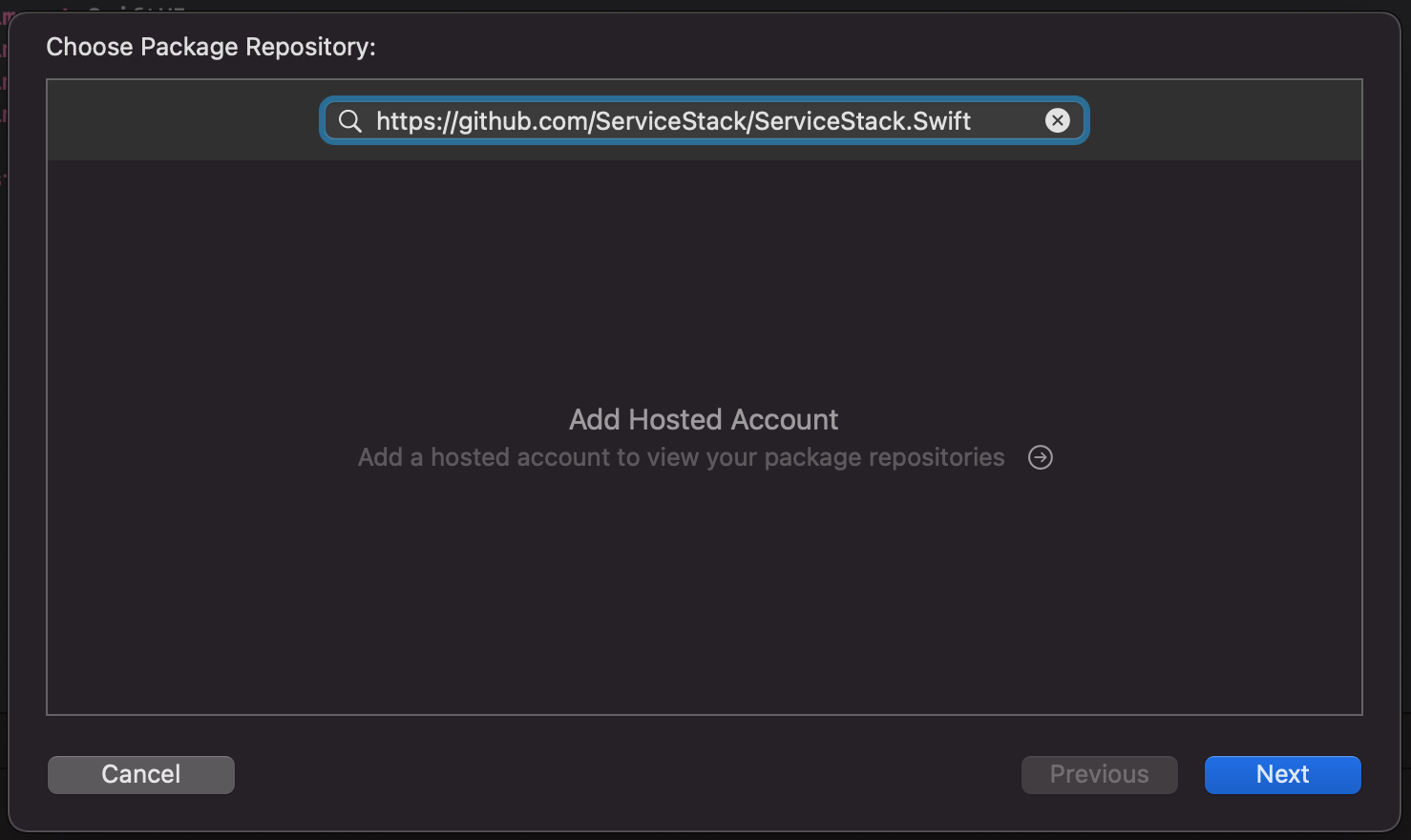
After adding the dependency both [ServiceStack.Swift](https://github.com/ServiceStack/ServiceStack.Swift) and its
[PromiseKit](https://github.com/mxcl/PromiseKit) dependency will be added to your project: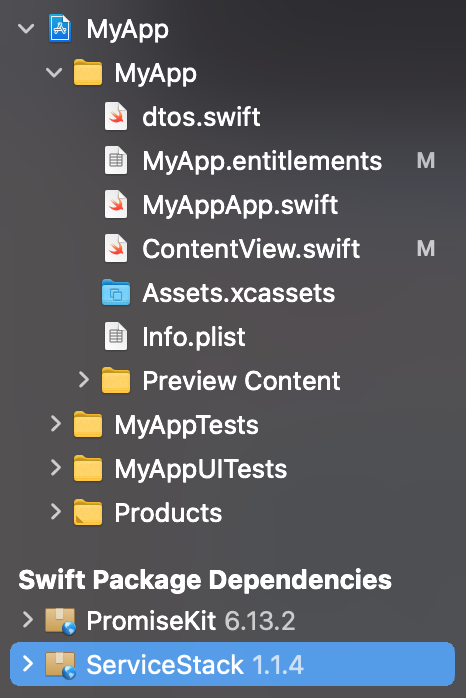
#### SwiftPM
```swift
dependencies: [
.package(name: "ServiceStack",
url: "https://github.com/ServiceStack/ServiceStack.Swift.git",
Version(6,0,0).. 6.0.2'
```#### Carthage
```ruby
github "ServiceStack/ServiceStack.Swift" ~> 6.0.2
```## API
```swift
public protocol ServiceClient {
func get(_ request: T) throws -> T.Return where T: Codable
func get(_ request: T) throws -> Void where T: Codable
func get(_ request: T, query: [String: String]) throws -> T.Return where T: Codable
func get(_ relativeUrl: String) throws -> T
func getAsync(_ request: T) async throws -> T.Return where T: Codable
func getAsync(_ request: T) async throws -> Void where T: Codable
func getAsync(_ request: T, query: [String: String]) async throws -> T.Return where T: Codable
func getAsync(_ relativeUrl: String) async throws -> Tfunc post(_ request: T) throws -> T.Return where T: Codable
func post(_ request: T) throws -> Void where T: Codable
func post(_ relativeUrl: String, request: Request?) throws -> Response
func postAsync(_ request: T) async throws -> T.Return where T: Codable
func postAsync(_ request: T) async throws -> Void where T: Codable
func postAsync(_ relativeUrl: String, request: Request?) async throws -> Responsefunc put(_ request: T) throws -> T.Return where T: Codable
func put(_ request: T) throws -> Void where T: Codable
func put(_ relativeUrl: String, request: Request?) throws -> Response
func putAsync(_ request: T) async throws -> T.Return where T: Codable
func putAsync(_ request: T) async throws -> Void where T: Codable
func putAsync(_ relativeUrl: String, request: Request?) async throws -> Responsefunc delete(_ request: T) throws -> T.Return where T: Codable
func delete(_ request: T) throws -> Void where T: Codable
func delete(_ request: T, query: [String: String]) throws -> T.Return where T: Codable
func delete(_ relativeUrl: String) throws -> T
func deleteAsync(_ request: T) async throws -> T.Return where T: Codable
func deleteAsync(_ request: T) async throws -> Void where T: Codable
func deleteAsync(_ request: T, query: [String: String]) async throws -> T.Return where T: Codable
func deleteAsync(_ relativeUrl: String) async throws -> Tfunc patch(_ request: T) throws -> T.Return where T: Codable
func patch(_ request: T) throws -> Void where T: Codable
func patch(_ relativeUrl: String, request: Request?) throws -> Response
func patchAsync(_ request: T) async throws -> T.Return where T: Codable
func patchAsync(_ request: T) async throws -> Void where T: Codable
func patchAsync(_ relativeUrl: String, request: Request?) async throws -> Responsefunc send(_ request: T) throws -> T.Return where T: Codable
func send(_ request: T) throws -> Void where T: Codable
func send(intoResponse: T, request: URLRequest) throws -> T
func sendAsync(intoResponse: T, request: URLRequest) async throws -> Tfunc postFileWithRequest(request:T, file:UploadFile) throws -> T.Return
func postFileWithRequestAsync(request:T, file:UploadFile) async throws -> T.Return
func postFileWithRequest(_ relativeUrl: String, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) throws -> T.Return
func postFileWithRequestAsync(_ relativeUrl: String, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) async throws -> T.Return
func postFileWithRequest(url:URL, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) throws -> T.Return
func postFileWithRequestAsync(url:URL, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) async throws -> T.Return
func postFilesWithRequest(request:T, files:[UploadFile]) throws -> T.Return
func postFilesWithRequestAsync(request:T, files:[UploadFile]) async throws -> T.Return
func postFilesWithRequest(url:URL, request:T, files:[UploadFile]) throws -> T.Return
func postFilesWithRequestAsync(url:URL, request:T, files:[UploadFile]) async throws -> T.Return
func putFileWithRequest(request:T, file:UploadFile) throws -> T.Return
func putFileWithRequestAsync(request:T, file:UploadFile) async throws -> T.Return
func putFileWithRequest(_ relativeUrl: String, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) throws -> T.Return
func putFileWithRequestAsync(_ relativeUrl: String, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) async throws -> T.Return
func putFileWithRequest(url:URL, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) throws -> T.Return
func putFileWithRequestAsync(url:URL, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) async throws -> T.Return
func putFilesWithRequest(request:T, files:[UploadFile]) throws -> T.Return
func putFilesWithRequestAsync(request:T, files:[UploadFile]) async throws -> T.Return
func putFilesWithRequest(url:URL, request:T, files:[UploadFile]) throws -> T.Return
func putFilesWithRequestAsync(url:URL, request:T, files:[UploadFile]) async throws -> T.Return
func sendFileWithRequest(_ req:inout URLRequest, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) throws -> T.Return
func sendFileWithRequestAsync(_ req:inout URLRequest, request:T, fileName:String, data:Data, mimeType:String?, fieldName:String?) async throws -> T.Return
func sendFilesWithRequest(_ req:inout URLRequest, request:T, files:[UploadFile]) throws -> T.Return
func sendFilesWithRequestAsync(_ req:inout URLRequest, request:T, files:[UploadFile]) async throws -> T.Returnfunc getData(url: String) throws -> (Data, HTTPURLResponse)?
func getDataAsync(url: String) async throws -> (Data, HTTPURLResponse)?
func getData(request: URLRequest, retryIf:((HTTPURLResponse) -> Bool)?) throws -> (Data, HTTPURLResponse)?
func getDataAsync(request: URLRequest, retryIf:((HTTPURLResponse) async throws -> Bool)?) async throws -> (Data, HTTPURLResponse)?func getCookies() -> [String:String]
func getTokenCookie() -> String?
func getRefreshTokenCookie() -> String?
}
```### v6.0.5 Release
- Replaced PromiseKit with Swift Concurrency's async/await
- Added new `postFileWithRequest` and `postFilesWithRequest` sync and async APIs### v6.0.1 Release
Added new sync and async file upload with Request APIs for POST and PUT HTTP Requests:
### v6.0.0 Release
The latest **v6** Release is now dependency-free, where its PromiseKit async APIs have been replaced to use
[Swift's native Concurrency](https://docs.swift.org/swift-book/documentation/the-swift-programming-language/concurrency/) support.```swift
import ServiceStacklet client = JsonServiceClient(baseUrl:baseUrl)
```#### Async
```swift
let request = Hello()
request.name = "World"let response = try await client.postAsync(request)
print(response.result!)
```#### Sync
```swift
let request = Hello()
request.name = "World"let response = try client.post(request)
print(response.result!)
```### v5.0.0 Release
The latest **v5** support for ServiceStack.Swift has been rewritten to use **Swift 5** and DTOs generated using Swift's new `Codable`
available in ServiceStack from **v5.10.5+**.#### Previous Version
To use a `JsonServiceStack` with DTOs generated earlier ServiceStack versions you'll need to reference the older **1.x** client version instead:
```swift
dependencies: [
.package(name: "ServiceStack", url: "https://github.com/ServiceStack/ServiceStack.Swift.git",
Version(1,0,0)..