Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/shibayan/Sharprompt
Interactive command-line based application framework for C#
https://github.com/shibayan/Sharprompt
cli command-line csharp dotnet interactive prompt terminal
Last synced: 2 months ago
JSON representation
Interactive command-line based application framework for C#
- Host: GitHub
- URL: https://github.com/shibayan/Sharprompt
- Owner: shibayan
- License: mit
- Created: 2019-07-29T17:38:55.000Z (over 5 years ago)
- Default Branch: master
- Last Pushed: 2024-04-29T02:13:07.000Z (9 months ago)
- Last Synced: 2024-05-02T05:07:44.166Z (8 months ago)
- Topics: cli, command-line, csharp, dotnet, interactive, prompt, terminal
- Language: C#
- Homepage:
- Size: 332 KB
- Stars: 711
- Watchers: 15
- Forks: 46
- Open Issues: 15
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
- License: LICENSE
- Code of conduct: CODE_OF_CONDUCT.md
Awesome Lists containing this project
- awesome-reference-tools - Sharprompt
README
# Sharprompt
[](https://github.com/shibayan/Sharprompt/actions/workflows/build.yml)
[](https://www.nuget.org/packages/Sharprompt/)
[](https://www.nuget.org/packages/Sharprompt/)
[](https://github.com/shibayan/Sharprompt/blob/master/LICENSE)Interactive command-line based application framework for C#
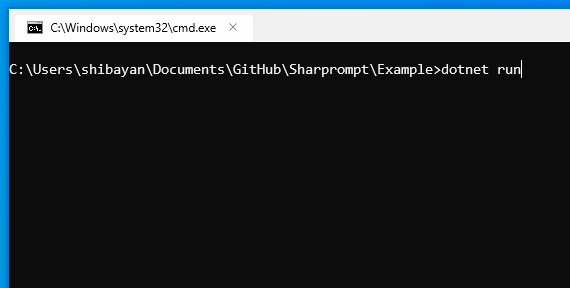
## Features
- Multi-platform support
- Supports the popular prompts (`Input` / `Password` / `Select` / etc)
- Supports model-based prompts
- Validation of input value
- Automatic generation of data source using Enum type
- Customizable symbols and color schema
- Unicode support (Multi-byte characters and Emoji😀🎉)## Installation
```
Install-Package Sharprompt
``````
dotnet add package Sharprompt
``````csharp
// Simple input
var name = Prompt.Input("What's your name?");
Console.WriteLine($"Hello, {name}!");// Password input
var secret = Prompt.Password("Type new password", validators: new[] { Validators.Required(), Validators.MinLength(8) });
Console.WriteLine("Password OK");// Confirmation
var answer = Prompt.Confirm("Are you ready?", defaultValue: true);
Console.WriteLine($"Your answer is {answer}");
```## Examples
The project in the folder `Sharprompt.Example` contains all the samples. Please check it.
```
dotnet run --project Sharprompt.Example
```## Prompt types
### Input
Takes a generic type parameter and performs type conversion as appropriate.
```csharp
var name = Prompt.Input("What's your name?");
Console.WriteLine($"Hello, {name}!");var number = Prompt.Input("Enter any number");
Console.WriteLine($"Input = {number}");
```
### Confirm
```csharp
var answer = Prompt.Confirm("Are you ready?");
Console.WriteLine($"Your answer is {answer}");
```
### Password
```csharp
var secret = Prompt.Password("Type new password");
Console.WriteLine("Password OK");
```
### Select
```csharp
var city = Prompt.Select("Select your city", new[] { "Seattle", "London", "Tokyo" });
Console.WriteLine($"Hello, {city}!");
```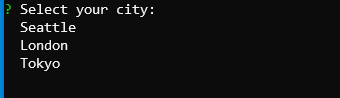
### MultiSelect (Checkbox)
```csharp
var cities = Prompt.MultiSelect("Which cities would you like to visit?", new[] { "Seattle", "London", "Tokyo", "New York", "Singapore", "Shanghai" }, pageSize: 3);
Console.WriteLine($"You picked {string.Join(", ", cities)}");
```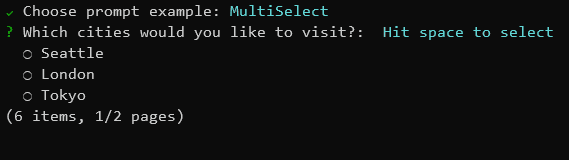
### List
```csharp
var value = Prompt.List("Please add item(s)");
Console.WriteLine($"You picked {string.Join(", ", value)}");
```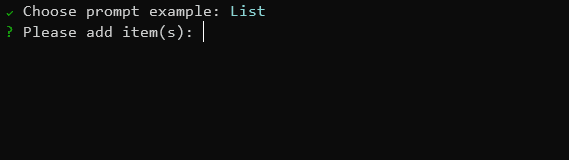
### Bind (Model-based prompts)
```csharp
// Input model definition
public class MyFormModel
{
[Display(Name = "What's your name?")]
[Required]
public string Name { get; set; }[Display(Name = "Type new password")]
[DataType(DataType.Password)]
[Required]
[MinLength(8)]
public string Password { get; set; }[Display(Name = "Select your city")]
[Required]
[InlineItems("Seattle", "London", "Tokyo")]
public string City { get; set; }[Display(Name = "Are you ready?")]
public bool? Ready { get; set; }
}var result = Prompt.Bind();
```## Configuration
### Symbols
```csharp
Prompt.Symbols.Prompt = new Symbol("🤔", "?");
Prompt.Symbols.Done = new Symbol("😎", "V");
Prompt.Symbols.Error = new Symbol("😱", ">>");var name = Prompt.Input("What's your name?");
Console.WriteLine($"Hello, {name}!");
```### Color schema
```csharp
Prompt.ColorSchema.Answer = ConsoleColor.DarkRed;
Prompt.ColorSchema.Select = ConsoleColor.DarkCyan;var name = Prompt.Input("What's your name?");
Console.WriteLine($"Hello, {name}!");
```### Cancellation support
```csharp
// Throw an exception when canceling with Ctrl-C
Prompt.ThrowExceptionOnCancel = true;try
{
var name = Prompt.Input("What's your name?");
Console.WriteLine($"Hello, {name}!");
}
catch (PromptCanceledException ex)
{
Console.WriteLine("Prompt canceled");
}
```## Features
### Enum type support
```csharp
public enum MyEnum
{
[Display(Name = "First value")]
First,
[Display(Name = "Second value")]
Second,
[Display(Name = "Third value")]
Third
}var value = Prompt.Select("Select enum value");
Console.WriteLine($"You selected {value}");
```### Unicode support
```csharp
// Prefer UTF-8 as the output encoding
Console.OutputEncoding = Encoding.UTF8;var name = Prompt.Input("What's your name?");
Console.WriteLine($"Hello, {name}!");
```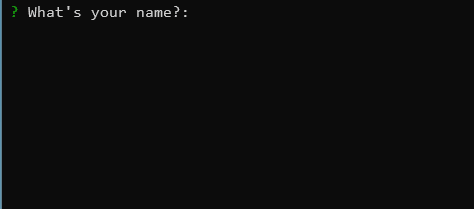
### Fluent interface support
```csharp
using Sharprompt.Fluent;// Use fluent interface
var city = Prompt.Select(o => o.WithMessage("Select your city")
.WithItems(new[] { "Seattle", "London", "Tokyo" })
.WithDefaultValue("Seattle"));
```## Supported platforms
- Windows
- Command Prompt
- PowerShell
- Windows Terminal
- Linux (Ubuntu, etc)
- Windows Terminal (WSL 2)
- macOS
- Terminal.app## License
This project is licensed under the [MIT License](https://github.com/shibayan/Sharprompt/blob/master/LICENSE)