Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/skarab42/tssert
🔥 Micro TypeScript assertion (test) library.
https://github.com/skarab42/tssert
assert assertion test testing typescript
Last synced: about 1 month ago
JSON representation
🔥 Micro TypeScript assertion (test) library.
- Host: GitHub
- URL: https://github.com/skarab42/tssert
- Owner: skarab42
- License: mit
- Created: 2022-07-04T11:27:16.000Z (over 2 years ago)
- Default Branch: main
- Last Pushed: 2023-07-20T17:51:58.000Z (over 1 year ago)
- Last Synced: 2024-11-30T20:36:23.468Z (about 2 months ago)
- Topics: assert, assertion, test, testing, typescript
- Language: TypeScript
- Homepage: https://www.npmjs.com/package/@skarab/tssert
- Size: 98.6 KB
- Stars: 0
- Watchers: 2
- Forks: 0
- Open Issues: 1
-
Metadata Files:
- Readme: README.md
- Contributing: CONTRIBUTING.md
- Funding: .github/FUNDING.yml
- License: LICENSE
Awesome Lists containing this project
README
# @skarab/tssert
Micro TypeScript assertion (test) library.
> Add your assertions to your usual test suite and run `tsc --noEmit`, enjoy!
📌 Please note that this library does not perform any check at runtime, but only at compile time (or in your IDE).
# Why ?
An attempt to reduce the [verbosity](https://github.com/colinhacks/zod/blob/51e93e7dd30b161d55e2f17d0907ecdd2f526c60/src/__tests__/default.test.ts#L51-L56) of type testing.
# Install
```bash
pnpm add @skarab/tssert typescript
```# Usages
## With a full description of the error
```ts
import { expectType } from '@skarab/tssert';expectType().toAccept(true);
expectType().toAccept(false); // ts-error with description
```## Without error description, but stricter and more flexible
```ts
expectType('Hello').toExtend('42').toBe(true);
expectType().toExtend('42').toBe(true);
expectType('Hello').toExtend().toBe(true);
expectType().toExtend().toBe(true);expectType().toExtend().toBe(true); // ts-error
expectType().toExtend().toBe(true);
```> When you move the mouse over `toAccept`, `toExtend` and `toEqual` you can see the expected and received values.
>
> 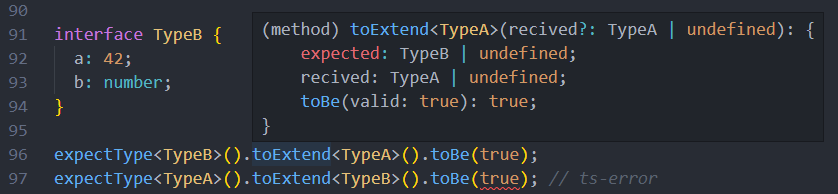# API
## toExtend()
```ts
interface TypeA {
a: 42;
b: string | number;
}interface TypeB {
a: 42;
b: number;
}expectType().toExtend().toBe(true);
expectType().toExtend().toBe(true); // ts-error
```## toEqual()
```ts
expectType().toEqual().toBe(true);
expectType().toEqual().toBe(true); // ts-error
expectType().toEqual().toBe(true); // ts-error
```## Types ...
```ts
import type { ExpectExtend, ExpectEqual } from '@skarab/tssert';type A = ExpectExtend; // true
type B = ExpectEqual; // false
```# TypeScript ~~god~~ strict mode
It is strongly recommended to activate the [strict](https://www.typescriptlang.org/tsconfig#strict) mode of TypeScript which will activate all checking behaviours that results in stronger guarantees of the program's correctness.
# Contributing 💜
See [CONTRIBUTING.md](https://github.com/skarab42/tssert/blob/main/CONTRIBUTING.md)