https://github.com/slorber/combine-promises
Like Promise.all(array) but with an object instead of an array.
https://github.com/slorber/combine-promises
async await ecmascript functional-js functional-programming javascript promise promises
Last synced: about 1 month ago
JSON representation
Like Promise.all(array) but with an object instead of an array.
- Host: GitHub
- URL: https://github.com/slorber/combine-promises
- Owner: slorber
- License: mit
- Created: 2021-04-07T07:50:11.000Z (about 4 years ago)
- Default Branch: main
- Last Pushed: 2023-08-17T10:48:48.000Z (over 1 year ago)
- Last Synced: 2025-04-04T02:07:33.788Z (about 1 month ago)
- Topics: async, await, ecmascript, functional-js, functional-programming, javascript, promise, promises
- Language: TypeScript
- Homepage: https://sebastienlorber.com
- Size: 166 KB
- Stars: 205
- Watchers: 4
- Forks: 5
- Open Issues: 3
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# Combine-Promises
[](https://www.npmjs.com/package/combine-promises)
[](https://github.com/slorber/combine-promises/actions/workflows/main.yml)

Like `Promise.all([])` but for objects.
```ts
import combinePromises from 'combine-promises';const { user, company } = await combinePromises({
user: fetchUser(),
company: fetchCompany(),
});
```Why:
- Insensitive to destructuring order
- Simpler async functional codeFeatures:
- TypeScript support
- Lightweight
- Feature complete
- Well-tested
- ESM / CJS---
# Sponsor
**[ThisWeekInReact.com](https://thisweekinreact.com)**: the best newsletter to stay up-to-date with the React ecosystem:
[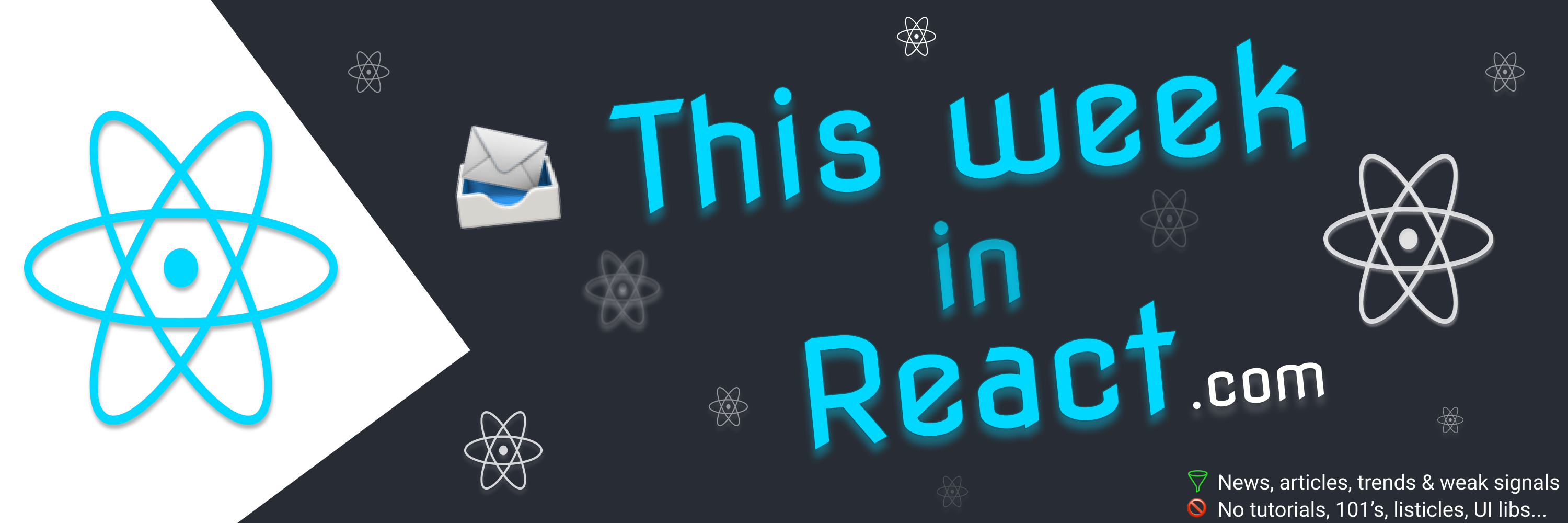](https://thisweekinreact.com)
---
## Install
```
npm install combine-promises
// OR
yarn add combine-promises
```## TypeScript support
Good, native and strict TypeScript support:
- Return type correctly inferred from the input object
- All object values should be async
- Only accept objects (reject arrays, null, undefined...)```ts
const result: { user: User; company: Company } = await combinePromises({
user: fetchUser(),
company: fetchCompany(),
});
```## Insensitive to destructuring order
A common error with `Promise.all` is to have a typo in the destructuring order.
```js
// Bad: destructuring order reversed
const [company, user] = await Promise.all([fetchUser(), fetchCompany()]);
```This becomes more dangerous as size of the promises array grows.
With `combinePromises`, you are using explicit names instead of array indices, which makes the code more robust and not sensitive to destructuring order:
```js
// Good: we don't care about the order anymore
const { company, user } = await combinePromises({
user: fetchUser(),
company: fetchCompany(),
});
```## Simpler async functional code
Suppose you have an object representing a friendship like `{user1: "userId-1", user2: "userId-2"}`, and you want to transform it to `{user1: User, user2: User}`.
You can easily do that:
```js
import combinePromises from 'combine-promises';
import { mapValues } from 'lodash'; // can be replaced by vanilla ES if you preferconst friendsIds = { user1: 'userId-1', user2: 'userId-2' };
const friends = await combinePromises(mapValues(friendsIds, fetchUserById));
```Without this library: good luck to keep your code simple.
## Inspirations
Name inspired by [combineReducers](https://redux.js.org/api/combinereducers) from Redux.