Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/smstuebe/xamarin-fingerprint
Xamarin and MvvMCross plugin for authenticate a user via fingerprint sensor
https://github.com/smstuebe/xamarin-fingerprint
xamarin xamarin-plugin
Last synced: 4 days ago
JSON representation
Xamarin and MvvMCross plugin for authenticate a user via fingerprint sensor
- Host: GitHub
- URL: https://github.com/smstuebe/xamarin-fingerprint
- Owner: smstuebe
- License: ms-pl
- Created: 2015-11-23T19:12:26.000Z (about 9 years ago)
- Default Branch: master
- Last Pushed: 2022-11-02T09:58:50.000Z (about 2 years ago)
- Last Synced: 2024-12-21T05:03:52.835Z (11 days ago)
- Topics: xamarin, xamarin-plugin
- Language: C#
- Homepage:
- Size: 6.85 MB
- Stars: 495
- Watchers: 31
- Forks: 118
- Open Issues: 65
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
- awesome-xamarin - Fingerprint ★165 - Xamarin and MvvMCross plugin for accessing the fingerprint sensor. (XPlat APIs)
README
#
Biometric / Fingerprint plugin for Xamarin
Xamarin and MvvMCross plugin for accessing the fingerprint, Face ID or other biometric sensors.
| Type | Stable | Pre release |
| --------- | ------------------------------------------------------------------------------------------------------------------------------------------------------------------------- | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| vanilla | [](https://www.nuget.org/packages/Plugin.Fingerprint/) | [](https://www.nuget.org/packages/Plugin.Fingerprint/) |
| MvvmCross | [](https://www.nuget.org/packages/MvvmCross.Plugins.Fingerprint/) | [](https://www.nuget.org/packages/MvvmCross.Plugins.Fingerprint/) |[Changelog](doc/changelog.md)
## Support
If you like the quality and code you can support me
- [](https://www.paypal.me/smstuebe)
Thanks!
The plugin supports the listed platforms.
| Platform | Version |
| --------------- | ------- |
| Xamarin.Android | 6.0 |
| Xamarin.iOS | 8.0 |
| Xamarin.Mac | 10.12 |
| Windows UWP | 10 |## Setup
### iOS
Add `NSFaceIDUsageDescription` to your Info.plist to describe the reason your app uses Face ID. (see [Documentation](https://developer.apple.com/library/content/documentation/General/Reference/InfoPlistKeyReference/Articles/CocoaKeys.html#//apple_ref/doc/uid/TP40009251-SW75)). Otherwise the App will crash when you start a Face ID authentication on iOS 11.3+.
```xml
NSFaceIDUsageDescription
Need your face to unlock secrets!
```### Android
**Set Target SDK version**
The target SDK version has to be >= 6.0. I recomment to use always the latest stable SDK version, if possible. You can set the target SDK version in your Android project properties.
**Install Android X Migration**
Since version 2, this plugin uses Android X. You have to install Xamarin.AndroidX.Migration in your Android project.
**Request the permission in AndroidManifest.xml**
```xml
```
**Set the resolver of the current Activity**
Skip this, if you use the MvvMCross Plugin or don't use the dialog.
We need the current activity to display the dialog. You can use the [Current Activity Plugin](https://github.com/jamesmontemagno/CurrentActivityPlugin) from James Montemagno or implement your own functionality to retrieve the current activity. See Sample App for details.
```csharp
CrossFingerprint.SetCurrentActivityResolver(() => CrossCurrentActivity.Current.Activity);
```## Usage
### Example
#### vanilla
```csharp
var request = new AuthenticationRequestConfiguration ("Prove you have fingers!", "Because without it you can't have access");
var result = await CrossFingerprint.Current.AuthenticateAsync(request);
if (result.Authenticated)
{
// do secret stuff :)
}
else
{
// not allowed to do secret stuff :(
}
```#### using MvvMCross
```csharp
var fpService = Mvx.Resolve(); // or use dependency injection and inject IFingerprintvar request = new AuthenticationRequestConfiguration ("Prove you have mvx fingers!", "Because without it you can't have access");
var result = await fpService.AuthenticateAsync(request);
if (result.Authenticated)
{
// do secret stuff :)
}
else
{
// not allowed to do secret stuff :(
}
```#### mocking in unit tests
```C#
//Create mock with LigthMock (http://www.lightinject.net/)
var mockFingerprintContext = new MockContext();
var mockFingerprint = new CrossFingerprintMock(mockFingerprintContext);mockFingerprintContext.Current = mockFingerprint;
```### Detailed Tutorial by @jfversluis
Youtube: Secure Your Xamarin App with Fingerprint or Face Recognition (click thumbnail)
[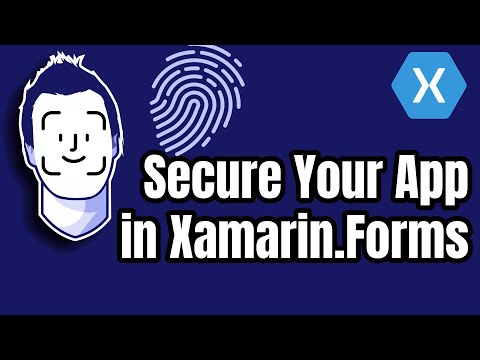](https://www.youtube.com/watch?v=k-eg3gcSMSU)
### API
The API is defined by the `IFingerprint` interface:
```csharp
///
/// Checks the availability of fingerprint authentication.
/// Checks are performed in this order:
/// 1. API supports accessing the fingerprint sensor
/// 2. Permission for accessint the fingerprint sensor granted
/// 3. Device has sensor
/// 4. Fingerprint has been enrolled
/// will be returned if the check failed
/// with some other platform specific reason.
///
///
/// En-/Disables the use of the PIN / Passwort as fallback.
/// Supported Platforms: iOS, Mac
/// Default: false
///
Task GetAvailabilityAsync(bool allowAlternativeAuthentication = false);///
/// Checks if returns .
///
///
/// En-/Disables the use of the PIN / Passwort as fallback.
/// Supported Platforms: iOS, Mac
/// Default: false
///
/// true if Available, else false
Task IsAvailableAsync(bool allowAlternativeAuthentication = false);///
/// Requests the authentication.
///
/// Reason for the fingerprint authentication request. Displayed to the user.
/// Token used to cancel the operation.
/// Authentication result
Task AuthenticateAsync(string reason, CancellationToken cancellationToken = default(CancellationToken));///
/// Requests the authentication.
///
/// Configuration of the dialog that is displayed to the user.
/// Token used to cancel the operation.
/// Authentication result
Task AuthenticateAsync(AuthenticationRequestConfiguration authRequestConfig, CancellationToken cancellationToken = default(CancellationToken));
```The returned `FingerprintAuthenticationResult` contains information about the authentication.
```csharp
///
/// Indicatates whether the authentication was successful or not.
///
public bool Authenticated { get { return Status == FingerprintAuthenticationResultStatus.Succeeded; } }///
/// Detailed information of the authentication.
///
public FingerprintAuthenticationResultStatus Status { get; set; }///
/// Reason for the unsucessful authentication.
///
public string ErrorMessage { get; set; }
```### iOS
#### Limitations
You can't create a custom dialog. The standard iOS Dialog will be shown.
##### iOS 9+ only
- cancelable programmatically with passed CancellationToken
- custom fallback button title##### iOS 10+ only
- custom cancel button title
### UWP
#### Limitations
You can't use the alternative authentication method.
## Testing on Simulators
### iOS

With the Hardware menu you can
- Toggle the enrollment status
- Trigger valid (⌘ ⌥ M) and invalid (⌘ ⌥ N) fingerprint sensor events### Android
- start the emulator (Android >= 6.0)
- open the settings app
- go to Security > Fingerprint, then follow the enrollment instructions
- when it asks for touch
- open command prompt
- `telnet 127.0.0.1 ` (`adb devices` prints "emulator-<emulator-id>")
- `finger touch 1`
- `finger touch 1`Sending fingerprint sensor events for testing the plugin can be done with the telnet commands, too.
**Note for Windows users:**
You have to enable telnet: Programs and Features > Add Windows Feature > Telnet Client## Nice to know
### Android code shrinker (Proguard & r8)
If you use the plugin with Link all, Release Mode and ProGuard/r8 enabled, you may have to do the following:
1. Create a `proguard.cfg` file in your android project and add the following:
```
-dontwarn com.samsung.**
-keep class com.samsung.** {*;}
```2. Include it to your project
3. Properties > Build Action > ProguardConfiguration## Contribution
+
![]()