https://github.com/souvikinator/notion-to-md
Convert Notion pages, blocks, or entire lists into any format: Markdown, MDX, JSX, HTML, LaTeX, and more. A powerful Notion conversion engine that lets you write once in Notion and publish seamlessly anywhere, in any format. Compatible with your favorite CMS, workflow, static site generator, and beyond.
https://github.com/souvikinator/notion-to-md
exporter hacktoberfest markdown md nodejs notion notion-api notion-client notion-convert notion-database notion-export notion-exporter notion-markdown notion-to-anything notion-to-html notion-to-md notion2md
Last synced: about 1 month ago
JSON representation
Convert Notion pages, blocks, or entire lists into any format: Markdown, MDX, JSX, HTML, LaTeX, and more. A powerful Notion conversion engine that lets you write once in Notion and publish seamlessly anywhere, in any format. Compatible with your favorite CMS, workflow, static site generator, and beyond.
- Host: GitHub
- URL: https://github.com/souvikinator/notion-to-md
- Owner: souvikinator
- License: mit
- Created: 2021-12-04T13:11:08.000Z (over 3 years ago)
- Default Branch: master
- Last Pushed: 2025-05-12T17:41:10.000Z (about 1 month ago)
- Last Synced: 2025-05-12T18:41:48.226Z (about 1 month ago)
- Topics: exporter, hacktoberfest, markdown, md, nodejs, notion, notion-api, notion-client, notion-convert, notion-database, notion-export, notion-exporter, notion-markdown, notion-to-anything, notion-to-html, notion-to-md, notion2md
- Language: TypeScript
- Homepage: https://notionconvert.com/docs/v4
- Size: 1.84 MB
- Stars: 1,328
- Watchers: 7
- Forks: 105
- Open Issues: 20
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
- License: LICENSE
Awesome Lists containing this project
- jimsghstars - souvikinator/notion-to-md - Convert notion pages, block and list of blocks to markdown (supports nesting and custom parsing) (TypeScript)
README
![]()
Notion-to-MD
(Notion to Markdown)
Notion-to-MD is a Node.js package that allows you to convert Notion pages to Markdown format.
Convert notion pages, blocks and list of blocks to markdown (supports nesting) using notion-sdk-js
![]()
![]()
![]()
## 🗒️ Recent posts
- Apr 23 - [Mastering Media Handling in notion-to-md v4 - Download, Upload, and Direct Strategies](https://notionconvert.com/blog/mastering-media-handling-in-notion-to-md-v4/)
- Mar 12 - [How to Convert Notion Properties to Frontmatter with notion-to-md v4](https://notionconvert.com/blog/how-to-convert-notion-properties-to-frontmatter/)
- Mar 12 - [How to Handle Documents in Notion Using notion-to-md v4](https://notionconvert.com/blog/how-to-handle-documents-in-notion-using-notion-to-md-v4/)
- Mar 11 - [How to Convert Notion Comments to Markdown Footnotes with notion-to-md v4](https://notionconvert.com/blog/how-to-use-notion-comments-as-footnotes-in-markdown/)## Looking for Support in Other Languages?
> If you've created a specific client, please open an issue to have it added here.- [notion-to-md-py](https://github.com/SwordAndTea/notion-to-md-py) by [@SwordAndTea](https://github.com/SwordAndTea)
## Install
```Bash
npm install notion-to-md
```## Usage
> ⚠️ **Note:** Before getting started, create [an integration and find the token](https://www.notion.so/my-integrations).
> Details on methods can be found in [API section](https://github.com/souvikinator/notion-to-md#api)> ⚠️ **Note:** Starting from v2.7.0, `toMarkdownString` no longer automatically saves child pages.
> Now it provides an object containing the markdown content of child pages.## converting markdown objects to markdown string
This is how the notion page looks for this example:
```javascript
const { Client } = require("@notionhq/client");
const { NotionToMarkdown } = require("notion-to-md");
const fs = require('fs');
// or
// import {NotionToMarkdown} from "notion-to-md";const notion = new Client({
auth: "your integration token",
});// passing notion client to the option
const n2m = new NotionToMarkdown({ notionClient: notion });(async () => {
const mdblocks = await n2m.pageToMarkdown("target_page_id");
const mdString = n2m.toMarkdownString(mdblocks);
console.log(mdString.parent);
})();
```## Separate child page content
**parent page content:**
**child page content:**
`NotionToMarkdown` takes second argument, `config`
```javascript
const { Client } = require("@notionhq/client");
const { NotionToMarkdown } = require("notion-to-md");
const fs = require('fs');
// or
// import {NotionToMarkdown} from "notion-to-md";const notion = new Client({
auth: "your integration token",
});// passing notion client to the option
const n2m = new NotionToMarkdown({
notionClient: notion,
config:{
separateChildPage:true, // default: false
}
});(async () => {
const mdblocks = await n2m.pageToMarkdown("target_page_id");
const mdString = n2m.toMarkdownString(mdblocks);
console.log(mdString);
})();
```**Output:**
`toMarkdownString` returns an object with target page content corresponding to `parent` property and if any child page exists then it's included in the same object.
User gets to save the content separately.
## Disable child page parsing
```javascript
...const n2m = new NotionToMarkdown({
notionClient: notion,
config:{
parseChildPages:false, // default: parseChildPages
}
});...
```## converting page to markdown object
**Example notion page:**
```js
const { Client } = require("@notionhq/client");
const { NotionToMarkdown } = require("notion-to-md");const notion = new Client({
auth: "your integration token",
});// passing notion client to the option
const n2m = new NotionToMarkdown({ notionClient: notion });(async () => {
// notice second argument, totalPage.
const x = await n2m.pageToMarkdown("target_page_id", 2);
console.log(x);
})();
```**Output:**
```json
[
{
"parent": "# heading 1",
"children": []
},
{
"parent": "- bullet 1",
"children": [
{
"parent": "- bullet 1.1",
"children": []
},
{
"parent": "- bullet 1.2",
"children": []
}
]
},
{
"parent": "- bullet 2",
"children": []
},
{
"parent": "- [ ] check box 1",
"children": [
{
"parent": "- [x] check box 1.2",
"children": []
},
{
"parent": "- [ ] check box 1.3",
"children": []
}
]
},
{
"parent": "- [ ] checkbox 2",
"children": []
}
]
```## converting list of blocks to markdown object
```js
const { Client } = require("@notionhq/client");
const { NotionToMarkdown } = require("notion-to-md");const notion = new Client({
auth: "your integration token",
});// passing notion client to the option
const n2m = new NotionToMarkdown({ notionClient: notion });(async () => {
// get all blocks in the page
const { results } = await notion.blocks.children.list({
block_id,
});//convert to markdown
const x = await n2m.blocksToMarkdown(results);
console.log(x);
})();
```**Output**: same as before
## Converting a single block to markdown string
- only takes a single notion block and returns corresponding markdown string
- nesting is ignored
- depends on @notionhq/client```js
const { NotionToMarkdown } = require("notion-to-md");// passing notion client to the option
const n2m = new NotionToMarkdown({ notionClient: notion });const result = n2m.blockToMarkdown(block);
console.log(result);
```**result**:
```
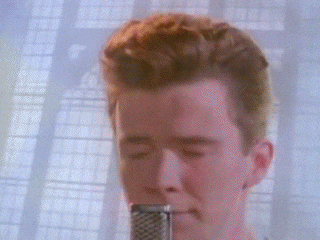
```## Custom Transformers
You can define your own custom transformer for a notion type, to parse and return your own string.
`setCustomTransformer(type, func)` will overload the parsing for the giving type.```ts
const { NotionToMarkdown } = require("notion-to-md");
const n2m = new NotionToMarkdown({ notionClient: notion });
n2m.setCustomTransformer("embed", async (block) => {
const { embed } = block as any;
if (!embed?.url) return "";
return `
${await n2m.blockToMarkdown(embed?.caption)}
`;
});
const result = n2m.blockToMarkdown(block);
// Result will now parse the `embed` type with your custom function.
```**Note** Be aware that `setCustomTransformer` will take only the last function for the given type. You can't set two different transforms for the same type.
You can also use the default parsing by returning `false` in your custom transformer.
```ts
// ...
n2m.setCustomTransformer("embed", async (block) => {
const { embed } = block as any;
if (embed?.url?.includes("myspecialurl.com")) {
return `...`; // some special rendering
}
return false; // use default behavior
});
const result = n2m.blockToMarkdown(block);
// Result will now only use custom parser if the embed url matches a specific url
```## Contribution
Pull requests are welcome. For major changes, please open an issue first to discuss what you would like to change.
Please make sure to update tests as appropriate.## Contributers
## License
[MIT](https://choosealicense.com/licenses/mit/)