Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/soywod/react-pin-field
📟 React component for entering PIN codes.
https://github.com/soywod/react-pin-field
code component field input pin react typescript
Last synced: 2 days ago
JSON representation
📟 React component for entering PIN codes.
- Host: GitHub
- URL: https://github.com/soywod/react-pin-field
- Owner: soywod
- License: mit
- Created: 2019-12-13T22:22:26.000Z (about 5 years ago)
- Default Branch: master
- Last Pushed: 2024-01-10T09:44:37.000Z (12 months ago)
- Last Synced: 2024-12-09T04:00:35.930Z (16 days ago)
- Topics: code, component, field, input, pin, react, typescript
- Language: TypeScript
- Homepage: https://soywod.github.io/react-pin-field/
- Size: 2.03 MB
- Stars: 412
- Watchers: 5
- Forks: 23
- Open Issues: 7
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Funding: .github/FUNDING.yml
- License: LICENSE
- Security: SECURITY.md
Awesome Lists containing this project
README
# 📟 React PIN Field [](https://github.com/soywod/react-pin-field/actions/workflows/test.yml) [](https://app.codecov.io/gh/soywod/react-pin-field) [](https://www.npmjs.com/package/react-pin-field)
React component for entering PIN codes.
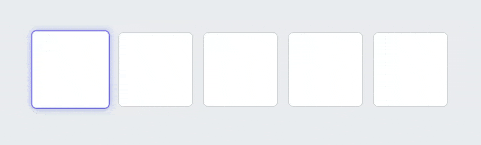
_Live demo at https://soywod.github.io/react-pin-field/._
## Installation
```bash
yarn add react-pin-field
# or
npm install react-pin-field
```## Usage
```typescript
import PinField from "react-pin-field";
```## Props
```typescript
type PinFieldProps = {
ref?: React.Ref;
className?: string;
length?: number;
validate?: string | string[] | RegExp | ((key: string) => boolean);
format?: (char: string) => string;
onResolveKey?: (key: string, ref?: HTMLInputElement) => any;
onRejectKey?: (key: string, ref?: HTMLInputElement) => any;
onChange?: (code: string) => void;
onComplete?: (code: string) => void;
style?: React.CSSProperties;
} & React.InputHTMLAttributes;const defaultProps = {
ref: {current: []},
className: "",
length: 5,
validate: /^[a-zA-Z0-9]$/,
format: key => key,
formatAriaLabel: (idx, length) => `pin code ${idx} of ${length}`,
onResolveKey: () => {},
onRejectKey: () => {},
onChange: () => {},
onComplete: () => {},
style: {},
};
```### Reference
Every input can be controlled thanks to the React reference:
```typescript
;// reset all inputs
ref.current.forEach(input => (input.value = ""));// focus the third input
ref.current[2].focus();
```### Style
The pin field can be styled either with `style` or `className`. This
last one allows you to use pseudo-classes like `:nth-of-type`,
`:focus`, `:hover`, `:valid`, `:invalid`…### Length
Length of the code (number of characters).
### Validate
Characters can be validated with a validator. A validator can take the
form of:- a String of allowed characters: `abcABC123`
- an Array of allowed characters: `["a", "b", "c", "1", "2", "3"]`
- a RegExp: `/^[a-zA-Z0-9]$/`
- a predicate: `(char: string) => boolean`### Format
Characters can be formatted with a formatter `(char: string) => string`.
### Format Aria Label(s)
This function is used to generate accessible labels for each input within the
``. By default it renders the string `pin code 1 of 6`,
`pin code 2 of 6`, etc., depending on the actual index of the input field
and the total length of the pin field.You can customize the aria-label string by passing your own function. This can
be useful for: i) site internationalisation (i18n); ii) simply describing
each input with different semantics than the ones provided by `react-pin-field`.### Events
- `onResolveKey`: when a key passes the validator
- `onRejectKey`: when a key is rejected by the validator
- `onChange`: when the code changes
- `onComplete`: when the code has been fully filled## Examples
See the [live demo](https://soywod.github.io/react-pin-field/).
## Development
```bash
git clone https://github.com/soywod/react-pin-field.git
cd react-pin-field
yarn install
```To start the development server:
```bash
yarn start
```To build the lib:
```bash
yarn build
```To build the demo:
```bash
yarn build:demo
```## Tests
### Unit tests
Unit tests are handled by [Jest](https://jestjs.io/) (`.test` files)
and [Enzyme](https://airbnb.io/enzyme/) (`.spec` files).```bash
yarn test:unit
```### End-to-end tests
End-to-end tests are handled by [Cypress](https://www.cypress.io)
(`.e2e` files).```bash
yarn start
yarn test:e2e
```## Sponsoring
[](https://github.com/sponsors/soywod)
[](https://www.paypal.com/paypalme/soywod)
[](https://ko-fi.com/soywod)
[](https://www.buymeacoffee.com/soywod)
[](https://liberapay.com/soywod)