https://github.com/space-code/cqrs
The Command and Query Responsibility Segregation
https://github.com/space-code/cqrs
cqrs ios macos swift swift-package-manager tvos watchos
Last synced: 4 months ago
JSON representation
The Command and Query Responsibility Segregation
- Host: GitHub
- URL: https://github.com/space-code/cqrs
- Owner: space-code
- License: mit
- Created: 2023-01-24T06:50:17.000Z (over 2 years ago)
- Default Branch: dev
- Last Pushed: 2024-01-12T14:09:57.000Z (over 1 year ago)
- Last Synced: 2024-09-16T17:06:40.351Z (10 months ago)
- Topics: cqrs, ios, macos, swift, swift-package-manager, tvos, watchos
- Language: Swift
- Homepage:
- Size: 328 KB
- Stars: 2
- Watchers: 2
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- License: LICENSE
Awesome Lists containing this project
README
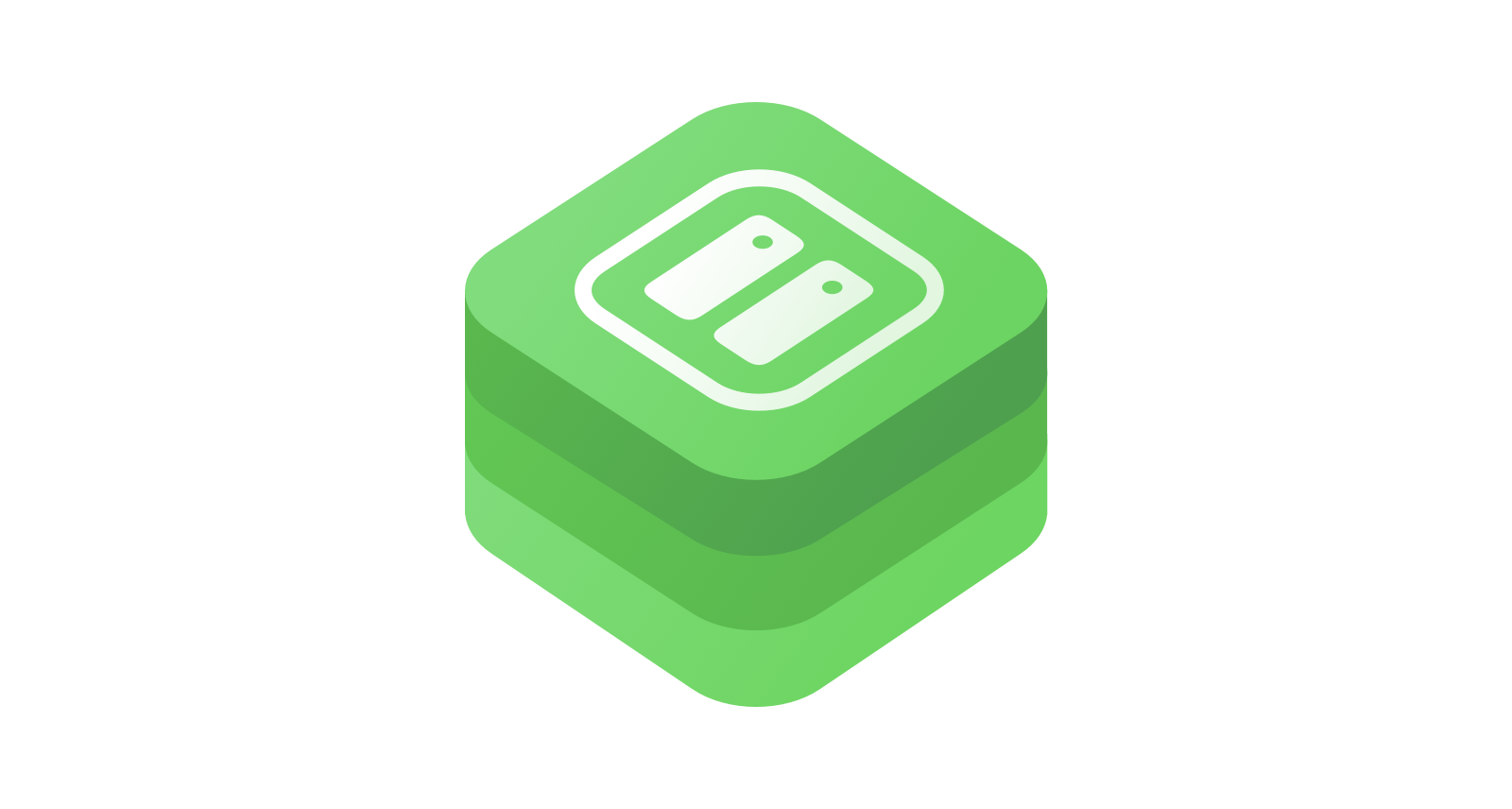
cqrs
## Description
`cqrs` is an implementation of the command and query responsibility segregation in Swift.- [Usage](#usage)
- [Requirements](#requirements)
- [Installation](#installation)
- [Communication](#communication)
- [Contributing](#contributing)
- [Author](#author)
- [License](#license)## Usage
1. `ICommand` and `IQuery` contain data for use by an appropriate handler.
Create an instance of a command that conforms to `ICommand` or a query that conforms to `IQuery`, respectively:
```swift
import CQRSfinal class ExampleCommand: ICommand {
// MARK: Properties
let value: Int
// MARK: Initialization
init(value: Int) {
self.value = value
}
}
``````swift
import CQRSfinal class ExampleQuery: IQueue {
typealias Result = Int// MARK: Properties
let value: Int
// MARK: Initialization
init(value: Int) {
self.value = value
}
}
```2. A command handler or a query handler contains the execution logic for a command or a query.
Create an instance of a command handler that conforms to `ICommandHandler` or a query handler that conforms to `IQueryHandler`, respectively:
```swift
import CQRSfinal class ExampleCommandHandler: ICommandHandler {
typealias Command = ExampleCommand// MARK: ICommandHandler
func execute(command: Command) throws {
// write the execution logic here
}
}
``````swift
import CQRSfinal class ExampleQueryHandler: IQueryHandler {
typealias Query = ExampleQuery// MARK: IQueryHandler
func execute(query: Query) throws -> Query.Result {
// write the execution logic here
}
}
```3. Register your handler implementation in the container:
```swift
import CQRSlet container = DependencyContainer()
container.register { ExampleCommandHandler() }
``````swift
import CQRSlet container = DependencyContainer()
container.register { ExampleQueryHandler() }
```4. Create an instance of a `CommandDispatcher` or a `QueryDispatcher` with the created container, like this:
```swift
import CQRSlet commandDispatcher = CommandDispatcher(container: container)
``````swift
import CQRSlet commandDispatcher = QueryDispatcher(container: container)
```5. Execute your command on `commandDispatcher` or `queryDispatcher`:
```swift
let command = ExampleCommand()do {
try commandDispatcher.execute(command: command)
} catch {
// Handle an error.
}
``````swift
let query = ExampleCommand()do {
let result = try queryDispatcher.execute(query: query)
} catch {
// Handle an error.
}
```## Requirements
- iOS 16.0+ / macOS 13+ / tvOS 16.0+ / watchOS 9.0+
- Xcode 14.0
- Swift 5.7## Installation
### Swift Package ManagerThe [Swift Package Manager](https://swift.org/package-manager/) is a tool for automating the distribution of Swift code and is integrated into the `swift` compiler. It is in early development, but `
cqrs` does support its use on supported platforms.Once you have your Swift package set up, adding `cqrs` as a dependency is as easy as adding it to the `dependencies` value of your `Package.swift`.
```swift
dependencies: [
.package(url: "https://github.com/space-code/cqrs.git", .upToNextMajor(from: "1.0.1"))
]
```## Communication
- If you **found a bug**, open an issue.
- If you **have a feature request**, open an issue.
- If you **want to contribute**, submit a pull request.## Contributing
Bootstrapping development environment```
make bootstrap
```Please feel free to help out with this project! If you see something that could be made better or want a new feature, open up an issue or send a Pull Request!
## Author
Nikita Vasilev, [email protected]## License
cqrs is available under the MIT license. See the LICENSE file for more info.