This is a text block! I have a
lot of inline formatting options!
Here's some text in
color
This is a link
```
Output
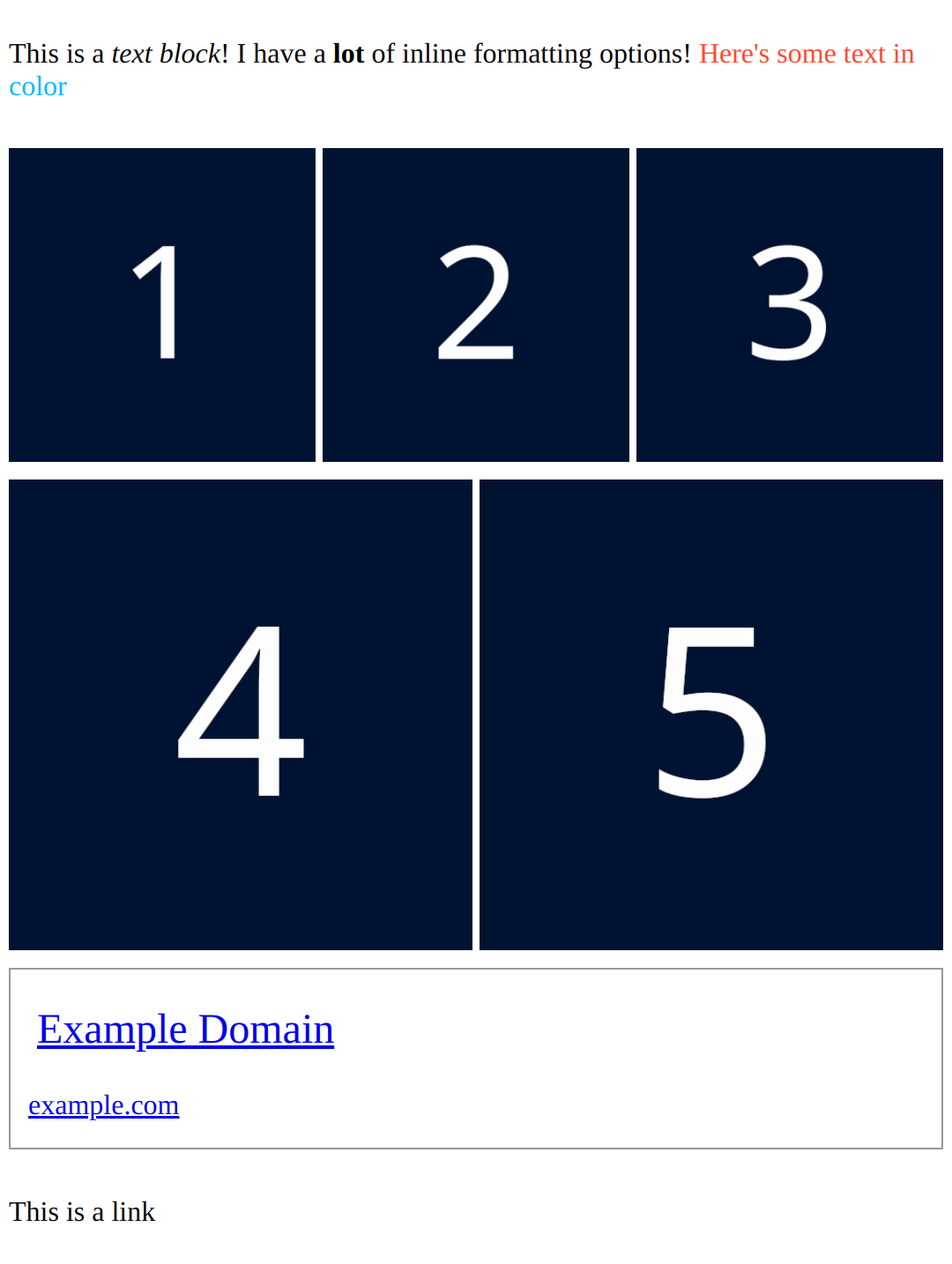
**Make sure to import the CSS from `npf_renderer.utils.BASIC_LAYOUT_CSS`!**
```python
with open("basic_layout.css", "w") as file:
file.write(npf_renderer.utils.BASIC_LAYOUT_CSS)
```
```html
```
`format_npf` will return placeholder HTML for any blocks it doesn't support.
Unsupported blocks
```html
This text block is supported but the next block is not!
```
In the event that it cannot format anything an empty div will be returned
---
## Installation
```bash
pip install npf-renderer
```
## Advanced
### URL Handling
You can pass in a custom URL handler function to `format_npf` to replace any links within the NPF tree.
```python
def url_handler(url):
url = urllib.parse.urlparse(url)
if url.hostname.endswith("example.com"):
return url._replace(netloc="other.example.com").geturl()
format_npf(contents, layouts, url_handler=url_handler)
```
### Polls
As polls require an additional request in order to fetch results, `npf-renderer` by default can only render a very basic poll without any votes attached.
You can however provide the data necessary for `npf-renderer` to populate polls by passing in a callback function to `format_npf` that takes a `poll_id` argument.
Be sure to have the blog name and the post ID at hand too, as Tumblr's API requires all three to fetch poll results.
The data returned should be in the form:
```jsonc
{
"results": {
// answer_id => vote_count
"9a025e86-2f02-452e-99d3-b4c0fd9afd48": 123,
"fad65faf-06d3-4a24-85a9-47c096ab07e3": 321
},
"timestamp": 1706642448
}
```
Example:
```python
def create_callback(blog_name, post_id)
def poll_callback(poll_id):
initial_results = request_poll_results(blog_name, post_id, poll_id)
return initial_results["response"]
return poll_callback
npf_renderer.format_npf(content, layout, poll_result_callback=create_callback(blog_name, post_id))
```
## Features
(True as of master branch)
- [x] Text blocks
- [x] Image blocks
- [x] Link Blocks
- [x] Audio Blocks
- [x] Video Blocks
- [x] Polls Blocks
- [x] Layouts
- [x] Attributions