https://github.com/tapanila/sharpcaster
Chromecast C# SDK for .net standard 2.0
https://github.com/tapanila/sharpcaster
android-platform chromecast chromecast-audio chromecast-device chromecast-receiver chromecast-sender csharp csharp-library nuget nuget-package sdk sharpcaster uwp windows-phone xamarin
Last synced: 1 day ago
JSON representation
Chromecast C# SDK for .net standard 2.0
- Host: GitHub
- URL: https://github.com/tapanila/sharpcaster
- Owner: Tapanila
- License: mit
- Created: 2016-01-15T13:59:36.000Z (over 9 years ago)
- Default Branch: main
- Last Pushed: 2025-02-10T15:14:54.000Z (3 months ago)
- Last Synced: 2025-05-14T23:06:19.554Z (1 day ago)
- Topics: android-platform, chromecast, chromecast-audio, chromecast-device, chromecast-receiver, chromecast-sender, csharp, csharp-library, nuget, nuget-package, sdk, sharpcaster, uwp, windows-phone, xamarin
- Language: C#
- Homepage:
- Size: 1.05 MB
- Stars: 315
- Watchers: 23
- Forks: 50
- Open Issues: 6
-
Metadata Files:
- Readme: README.md
- License: LICENSE.txt
- Code of conduct: CODE_OF_CONDUCT.md
Awesome Lists containing this project
README

# SharpCaster### Currently Supported Platforms
* [.NET Standard 2.0](https://docs.microsoft.com/en-us/dotnet/standard/net-standard?tabs=net-standard-2-0)[](https://github.com/Tapanila/SharpCaster/actions/workflows/dotnet.yml)
SharpCaster is Chromecast C# SDK any platform support .net standard 2.0.
## The nuget package [](https://www.nuget.org/packages/SharpCaster/)
https://nuget.org/packages/SharpCaster/
PM> Install-Package SharpCaster
# Getting started
## Finding chromecast devices from network
```cs
IChromecastLocator locator = new MdnsChromecastLocator();
var source = new CancellationTokenSource(TimeSpan.FromMilliseconds(1500));
var chromecasts = await locator.FindReceiversAsync(source.Token);
```
## Connecting to chromecast device, launch application and load media
```cs
var chromecast = chromecasts.First();
var client = new ChromecastClient();
await client.ConnectChromecast(chromecast);
_ = await client.LaunchApplicationAsync("B3419EF5");var media = new Media
{
ContentUrl = "https://commondatastorage.googleapis.com/gtv-videos-bucket/CastVideos/mp4/DesigningForGoogleCast.mp4"
};
_ = await client.MediaChannel.LoadAsync(media);
```## SharpCaster Demo
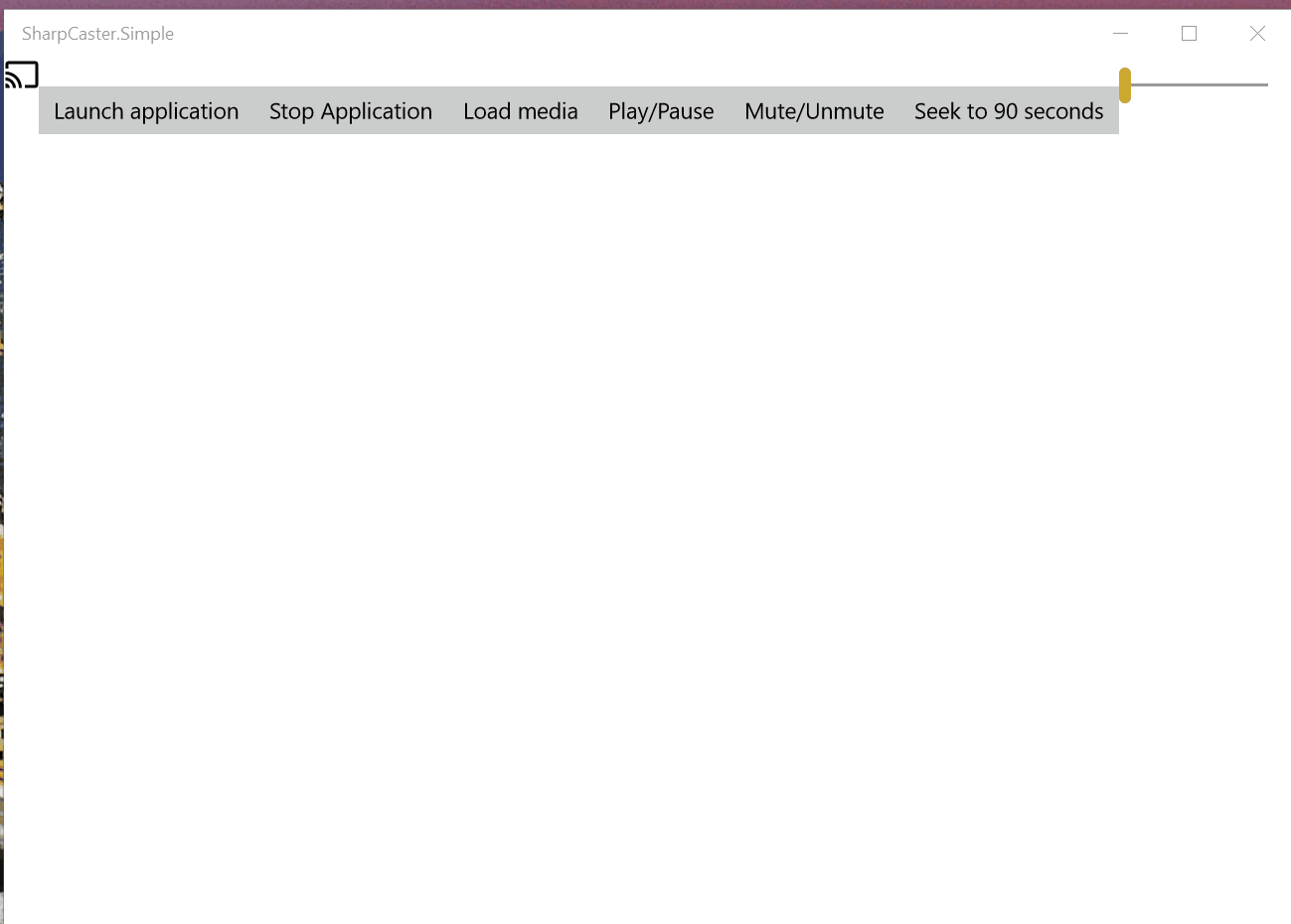
## Adding support for custom chromecast channels
* In Chrome, go to `chrome://net-export/`
* Select 'Include raw bytes (will include cookies and credentials)'
* Click 'Start Logging to Disk'
* Open a new tab, browse to your favorite application on the web that has Chromecast support and start casting.
* Go back to the tab that is capturing events and click on stop.
* Open https://netlog-viewer.appspot.com/ and select your event log file.
* Browse to https://netlog-viewer.appspot.com/#events&q=type:SOCKET, and find the socket that has familiar JSON data.
* Go through the results and collect the JSON that is exchanged.
* Now you can create a new class that inherits from ChromecastChannel and implement the logic to send and receive messages.