Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/tiagoprata/fastapi-frame-stream
Python package to easily stream individual frames (MJPEG) using FastAPI
https://github.com/tiagoprata/fastapi-frame-stream
fastapi mjpeg mjpeg-stream opencv python video-streaming
Last synced: about 1 month ago
JSON representation
Python package to easily stream individual frames (MJPEG) using FastAPI
- Host: GitHub
- URL: https://github.com/tiagoprata/fastapi-frame-stream
- Owner: TiagoPrata
- License: mit
- Created: 2022-06-24T18:31:23.000Z (over 2 years ago)
- Default Branch: main
- Last Pushed: 2022-07-07T22:16:09.000Z (over 2 years ago)
- Last Synced: 2024-10-15T17:06:06.281Z (about 1 month ago)
- Topics: fastapi, mjpeg, mjpeg-stream, opencv, python, video-streaming
- Language: Python
- Homepage: https://pypi.org/project/fastapi-frame-stream/
- Size: 7.98 MB
- Stars: 12
- Watchers: 3
- Forks: 1
- Open Issues: 2
-
Metadata Files:
- Readme: readme.md
- Changelog: changelog.md
- License: LICENSE
Awesome Lists containing this project
README
# fastapi-frame-stream
Package to easily stream individual frames (MJPEG) using FastAPI.
FastAPI server for publishing and viewing MJPEG streams.
Raw image files and images as base64 strings can be sent to a 'video stream' and then consumed by any client.
## Quick start
### Installing
```cmd
pip install fastapi-frame-stream
```#### Requirements
- [FastAPI](https://fastapi.tiangolo.com/)
- [uvicorn](https://www.uvicorn.org/)NOTE:
This package will also automatically install:- imutils
- opencv-python
- python-miltipart### How to use
#### Server
You can create a simple FastAPI server where it is possible to publish and get multiple streams.

full code
```python
from fastapi import FastAPI, File, UploadFile
import uvicorn
from pydantic import BaseModel
from fastapi_frame_stream import FrameStreamerapp = FastAPI()
fs = FrameStreamer()class InputImg(BaseModel):
img_base64str : str@app.post("/send_frame_from_string/{stream_id}")
async def send_frame_from_string(stream_id: str, d:InputImg):
await fs.send_frame(stream_id, d.img_base64str)@app.post("/send_frame_from_file/{stream_id}")
async def send_frame_from_file(stream_id: str, file: UploadFile = File(...)):
await fs.send_frame(stream_id, file)@app.get("/video_feed/{stream_id}")
async def video_feed(stream_id: str):
return fs.get_stream(stream_id)if __name__ == '__main__':
uvicorn.run(app, host="0.0.0.0", port=5000)
```#### Client
Any client can view a published image (MJPEG) stream using a simple ```
``` tag:

full code
```html
Testing fastapi-frame-stream
```
#### All together
It is possible to upload an image file directly...
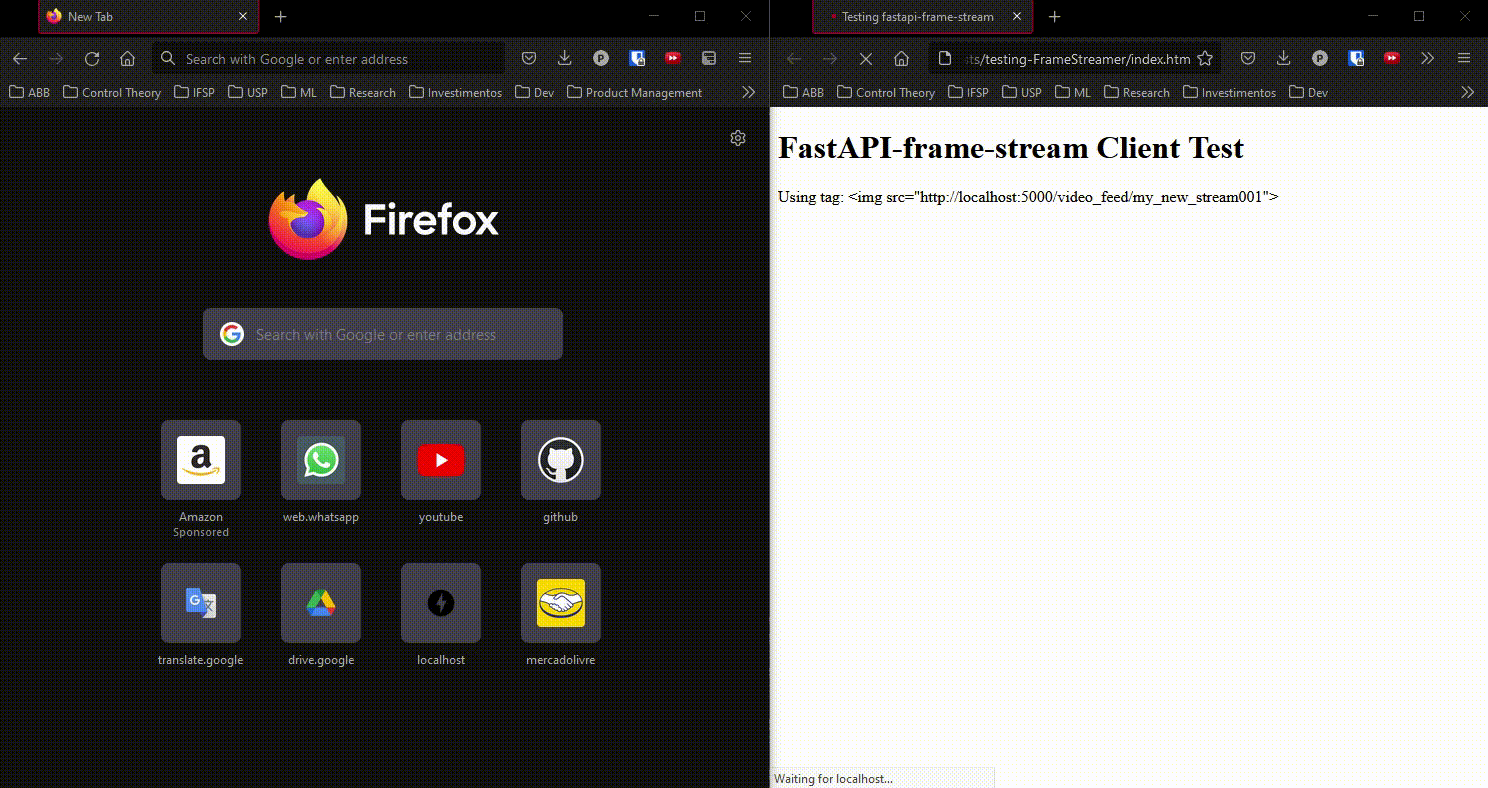
... or to use any kind of application to convert the frames to base64 and send it to the web server:
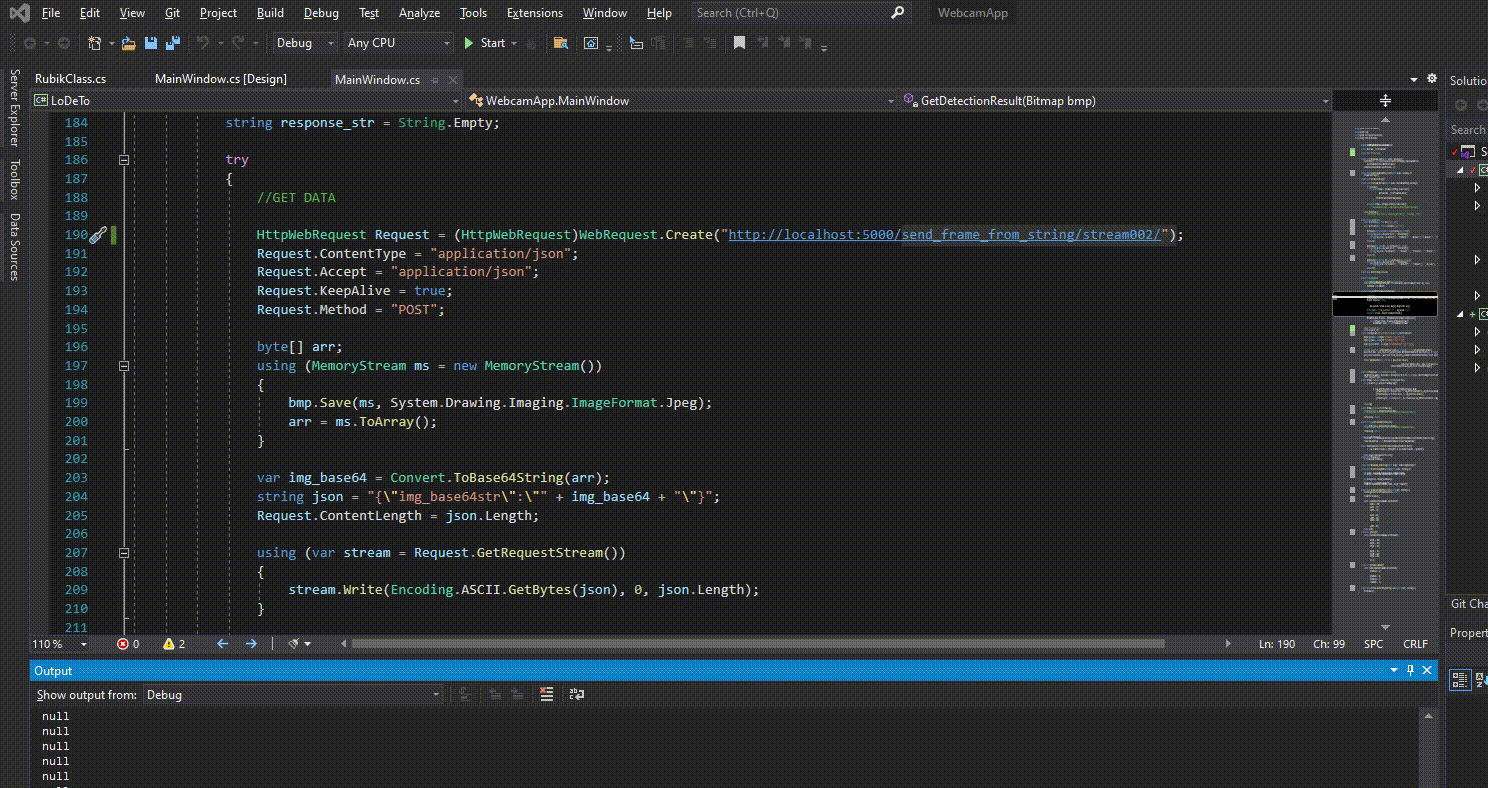
How it works
The frames sent throught the web server are stored in a temporary (in memory) SQLite DB...

... and the last frame of each stream is retrieved everytime a client wants to visualize the stream.
