Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/tristanhimmelman/HidingNavigationBar
Easily hide and show a view controller's navigation bar (and tab bar) as a user scrolls
https://github.com/tristanhimmelman/HidingNavigationBar
Last synced: 3 months ago
JSON representation
Easily hide and show a view controller's navigation bar (and tab bar) as a user scrolls
- Host: GitHub
- URL: https://github.com/tristanhimmelman/HidingNavigationBar
- Owner: tristanhimmelman
- License: mit
- Created: 2015-05-01T21:03:15.000Z (over 9 years ago)
- Default Branch: master
- Last Pushed: 2022-06-08T19:21:19.000Z (over 2 years ago)
- Last Synced: 2024-07-19T07:36:00.830Z (4 months ago)
- Language: Swift
- Homepage:
- Size: 2.7 MB
- Stars: 1,031
- Watchers: 31
- Forks: 127
- Open Issues: 32
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
- awesome-ios - HidingNavigationBar - Easily hide and show a view controller's navigation bar (and tab bar) as a user scrolls (UI / Navigation Bar)
- awesome-ios-star - HidingNavigationBar - Easily hide and show a view controller's navigation bar (and tab bar) as a user scrolls (UI / Navigation Bar)
README
HidingNavigationBar
==============
[](https://github.com/Carthage/Carthage)
[](https://github.com/tristanhimmelman/HidingNavigationBar)An easy to use library (written in Swift) that manages hiding and showing a navigation bar as a user scrolls.
- [Features](#features)
- [Usage](#usage)
- [Customization](#customization)
- [Installation](#installation)# Features
HidingNavigationBar supports hiding/showing of the following view elements:
- UINavigationBar
- UINavigationBar and an extension UIView
- UINavigationBar and a UIToolbar
- UINavigationBar and a UITabBar### UINavigationBar
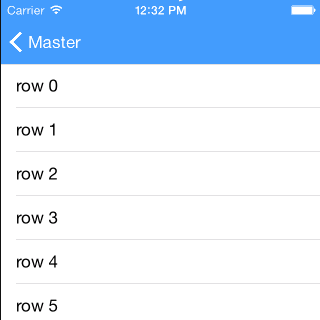
### UINavigationBar and an extension UIView
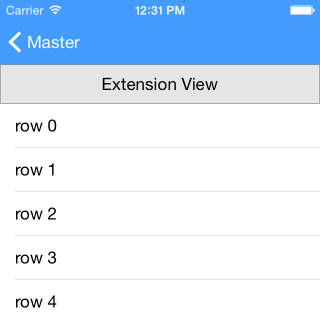
### UINavigationBar and a UIToolbar
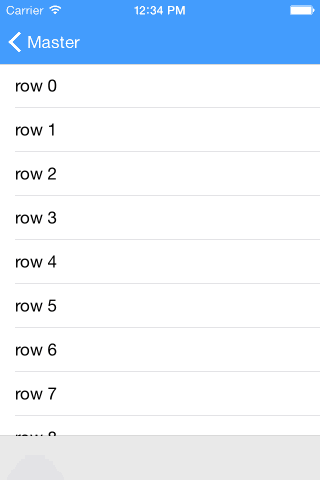
### A UINavigationBar and a UITabBar
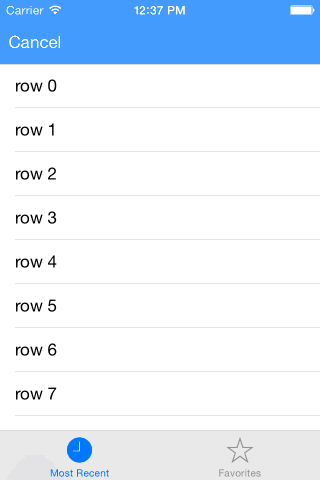# Usage
1. Import HidingNavigationBar
2. Include a member variable of type `HidingNavigationBarManager` in your `UIViewController` subclass.
3. Initialize the variable in `viewDidLoad` function, passing in the `UIViewController` instance and the `UIScrollView` instance that will control the hiding/showing of the navigation bar.
4. Relay the following `UIViewController` lifecycle functions to the `HidingNavigationBarManager` variable:
```swift
override func viewWillAppear(animated: Bool)
override func viewWillDisappear(animated: Bool)
override func viewDidLayoutSubviews() //Only necessary when adding the extension view
```
And finally relay the following `UIScrollViewDelegate` function:
```swift
func scrollViewShouldScrollToTop(scrollView: UIScrollView) -> Bool
```Below is an example of how your UIViewController subclass should look:
```swift
import HidingNavigationBarclass MyViewController: UIViewController, UITableViewDataSource, UITableViewDelegate {
var hidingNavBarManager: HidingNavigationBarManager?
@IBOutlet weak var tableView: UITableView!override func viewDidLoad() {
super.viewDidLoad()hidingNavBarManager = HidingNavigationBarManager(viewController: self, scrollView: tableView)
}override func viewWillAppear(animated: Bool) {
super.viewWillAppear(animated)hidingNavBarManager?.viewWillAppear(animated)
}override func viewDidLayoutSubviews() {
super.viewDidLayoutSubviews()hidingNavBarManager?.viewDidLayoutSubviews()
}override func viewWillDisappear(animated: Bool) {
super.viewWillDisappear(animated)hidingNavBarManager?.viewWillDisappear(animated)
}//// TableView datasoure and delegate
func scrollViewShouldScrollToTop(scrollView: UIScrollView) -> Bool {
hidingNavBarManager?.shouldScrollToTop()return true
}...
}
```Note: HidingNavigationBar only works with UINavigationBars that have translucent set to true.
# Customization
### Add an extension view to the UINavigationBar
```swift
let extensionView = // load your a UIView to use as an extension
hidingNavBarManager?.addExtensionView(extensionView)
```
### Hide and show a UITabBar or UIToolbar
```swift
if let tabBar = navigationController?.tabBarController?.tabBar {
hidingNavBarManager?.manageBottomBar(tabBar)
}
```### Hide/Show/Do Nothing when App is Foregrounded
```swift
hidingNavBarManager?.onForegroundAction = .Default //Do nothing, state of bars will remain the same as when backgrounded
hidingNavBarManager?.onForegroundAction = .Hide //Always hide on foreground
hidingNavBarManager?.onForegroundAction = .Show //Always show on foreground
```### Expansion Resistance
When the navigation bar is hidden, you can some 'resitance' which adds a delay before the navigation bar starts to expand when scrolling. The resistance value is the distance that the user needs to scroll before the navigation bar starts to expand.
```swift
hidingNavBarManager?.expansionResistance = 150
```### UIRefreshControl
If you are using a UIRefreshControl with your scroll view, it is important to let the `HidingNavigationBarManager` know about it:
```swift
hidingNavBarManager?.refreshControl = refreshControl
```# Installation
If your using [Carthage](https://github.com/Carthage/Carthage), add the following line to your Cartfile:
```
github "tristanhimmelman/HidingNavigationBar" ~> 2.0
```(for Swift 3, use `github "tristanhimmelman/HidingNavigationBar" ~> 1.0` instead)
If you are using [CocoaPods](https://cocoapods.org/), add the following line to your Podfile:
`pod 'HidingNavigationBar', '~> 2.0'`
(for Swift 3, use `pod 'HidingNavigationBar', '~> 1.0'` instead)
Otherwise, include the following files directly to your project:
- HidingNavigationBarManager.swift
- HidingViewController.swift