Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/tryexceptpass/sofi
an OS agnostic UI module for Python
https://github.com/tryexceptpass/sofi
gui python widget
Last synced: 2 days ago
JSON representation
an OS agnostic UI module for Python
- Host: GitHub
- URL: https://github.com/tryexceptpass/sofi
- Owner: tryexceptpass
- License: mit
- Created: 2016-02-18T03:36:39.000Z (almost 9 years ago)
- Default Branch: master
- Last Pushed: 2021-06-11T17:45:13.000Z (over 3 years ago)
- Last Synced: 2024-12-14T10:06:47.201Z (9 days ago)
- Topics: gui, python, widget
- Language: Python
- Homepage:
- Size: 256 KB
- Stars: 387
- Watchers: 37
- Forks: 49
- Open Issues: 29
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
[](https://travis-ci.org/tryexceptpass/sofi) [](https://waffle.io/tryexceptpass/sofi) [](https://gitter.im/try-except-pass/sofi?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
[](http://sofi-gui-framework.readthedocs.io/en/latest/?badge=latest)
[](https://saythanks.io/to/tryexceptpass)## Overview
`sofi` is an OS agnostic UI module for Python.The main idea is to allow rapid, pythonic GUI development using standard web-based widgets from `Bootstrap` and
other common HTML5 libraries and package them in such a way that all event processing is done within python using
`websockets`.This was developed as an exercise in poking at the underlying technologies and to see what comes out of merging them together. For a better overview of why it came to be, feel free to take a look at this post: [A Python Ate My GUI - Part 3: Implementation](http://tryexceptpass.org/article/a-python-ate-my-gui-3)
We're in a **beta** stage for now, feedback appreciated.
## Usage
The `sofi.app.Sofi` object runs the main event thread with `.start()` and provides a number of functions to help manipulate the state of the user interface, which is actually a webpage opened in a browser. The `register` and `unregister` methods provide the mechanism for subscribing to events through callbacks, while the remaining functions (`append`, `replace`, `style`, etc.) wrap the commands intended to dynamically update the widgets (which are actual HTML elements).The commands map directly to `D3` or `jQuery` methods present in the `sofi.js` library that's loaded during initialization and is responsible for performing the requested operations.
Following basic practices from bootstrap, the widgets should be within a `Container`. The base page itself is represented with the `View` class, which wraps the necessary head, body and style tags that will contain the widgets.
Below is a quick idea of how to get things going, but check out `sample.py` for a more complicated hello world which instantiates a navbar item, adds a few links, creates some buttons, registers events and performs some timed updates.
```python
from sofi.app import Sofi
from sofi.ui import Container, Paragraph, Heading, Viewimport json
import asyncioasync def onload(event):
# Every page is built on top of a View object, which contains the and tags that are filled in by the other objects
v = View()# Make a bootstrap container in which to put all your widgets
c = Container()# Add a heading and paragraph to the container
c.addelement(Heading(2, "Dude!"))
c.addelement(Paragraph("Where's My Car?"))# Add the container to the view
v.addelement(c)# Tell the UI to load the HTML generated by the view
app.load(str(v))# Instantiate the application
app = Sofi()# Register the event handler that runs when the UI is ready to receive commands
app.register('init', onload)# Start the app (opens the default browser) and listen for events
app.start()
```## What do the widgets look like?
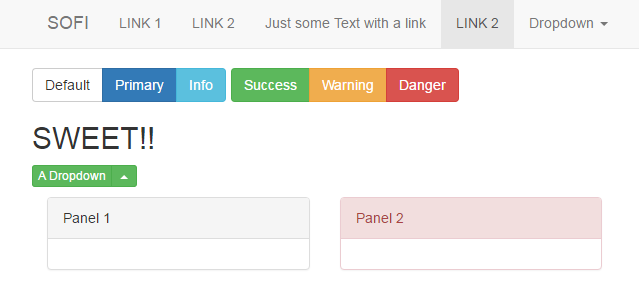
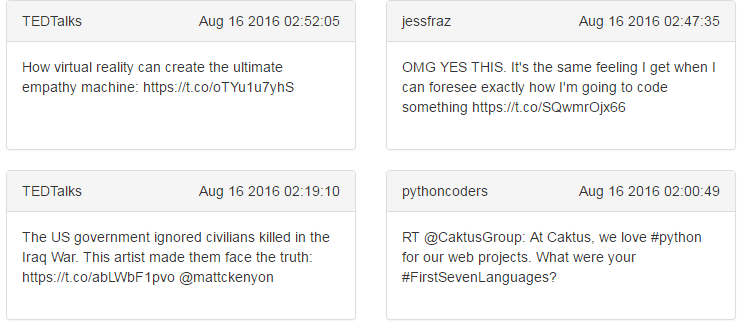