Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/tscircuit/jscad-fiber
Create 3d CAD models with React using jscad
https://github.com/tscircuit/jscad-fiber
3d cad hacktoberfest react
Last synced: 1 day ago
JSON representation
Create 3d CAD models with React using jscad
- Host: GitHub
- URL: https://github.com/tscircuit/jscad-fiber
- Owner: tscircuit
- License: mit
- Created: 2024-07-04T19:22:56.000Z (7 months ago)
- Default Branch: main
- Last Pushed: 2025-01-23T04:11:54.000Z (16 days ago)
- Last Synced: 2025-01-30T12:09:01.194Z (8 days ago)
- Topics: 3d, cad, hacktoberfest, react
- Language: TypeScript
- Homepage:
- Size: 1.79 MB
- Stars: 26
- Watchers: 2
- Forks: 13
- Open Issues: 18
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# jscad-fiber
[View examples](https://tscircuit.github.io/jscad-fiber/) · [jscad](https://github.com/jscad/OpenJSCAD.org) · [tscircuit](https://github.com/tscircuit/tscircuit) · [jscad-electronics](https://github.com/tscircuit/jscad-electronics)
This package allows you to create 3D CAD objects with React and JSCAD.
## Table of Contents
- [Installation](#installation)
- [Usage](#usage)
- [Common Props](#common-props)
- [Components](#components)
- [Basic Shapes](#basic-shapes)
- [Boolean Operations](#boolean-operations)
- [Transformations](#transformations)
- [Extrusions](#extrusions)
- [Example Wrapper](#example-wrapper)
- [Why?](#why)
- [Contributing](#contributing)
## Installation
```bash
npm install jscad-fiber @jscad/modeling three @react-three/fiber
```## Usage
Create JSCAD components with React:
```tsx
import { JsCadView } from "jscad-fiber"
import { Cube, Sphere, Subtract } from "jscad-fiber"export default () => (
)
```## Common Props
All components support these common props:
| Prop | Type | Description |
|------|------|-------------|
| `color` | `string \| [number,number,number]` | CSS color string or RGB array |
| `center` | `[number,number,number] \| {x,y,z}` | Position in 3D space |
| `offset` | `[number,number,number] \| {x,y,z}` | Alternative to center |## Components
### Basic Shapes
#### Cube
| Prop | Type | Description |
|------|------|-------------|
| `size` | `number \| [number,number,number]` | Single number for all dimensions or [width, height, depth] |```tsx
// Single number for all dimensions
// Array for different dimensions
```#### Sphere
| Prop | Type | Description |
|------|------|-------------|
| `radius` | `number` | Sphere radius |
| `segments` | `number` | Optional detail level (default: 32) |```tsx
```
#### Cylinder
| Prop | Type | Description |
|------|------|-------------|
| `radius` | `number` | Cylinder radius |
| `height` | `number` | Cylinder height |```tsx
```
#### Torus
| Prop | Type | Description |
|------|------|-------------|
| `innerRadius` | `number` | Inner ring radius |
| `outerRadius` | `number` | Outer ring radius |
| `innerSegments` | `number` | Optional inner detail level (default: 32) |
| `outerSegments` | `number` | Optional outer detail level (default: 32) |```tsx
```
### Boolean Operations
#### Subtract
| Prop | Type | Description |
|------|------|-------------|
| `children` | `React.ReactNode[]` | First child is base shape, others are subtracted |```tsx
{/* Base shape */}
{/* Subtracted from base */}```
#### Union
| Prop | Type | Description |
|------|------|-------------|
| `children` | `React.ReactNode[]` | Shapes to combine |```tsx
```
#### Hull
| Prop | Type | Description |
|------|------|-------------|
| `children` | `React.ReactNode[]` | Shapes to create hull from |```tsx
```
### Transformations
#### Translate
| Prop | Type | Description |
|------|------|-------------|
| `offset` | `[number,number,number]` | Translation in [x,y,z] |```tsx
```
#### Rotate
| Prop | Type | Description |
|------|------|-------------|
| `angles` | `[number,number,number]` | Rotation angles in radians [x,y,z] |```tsx
```
### Extrusions
#### ExtrudeLinear
| Prop | Type | Description |
|------|------|-------------|
| `height` | `number` | Extrusion height |
| `twistAngle` | `number` | Optional twist during extrusion |```tsx
```
#### ExtrudeRotate
| Prop | Type | Description |
|------|------|-------------|
| `angle` | `number` | Rotation angle in radians |
| `segments` | `number` | Optional number of segments |```tsx
```
## Component Props Reference
| Component | Props | Description |
|-----------|-------|-------------|
| Cube | `size: number \| [number,number,number]` | Size in each dimension |
| Sphere | `radius: number`, `segments?: number` | Radius and detail level |
| Cylinder | `radius: number`, `height: number` | Basic cylinder dimensions |
| Torus | `innerRadius: number`, `outerRadius: number`, `segments?: number` | Ring dimensions |
| ExtrudeLinear | `height: number`, `twistAngle?: number` | Linear extrusion with optional twist |
| ExtrudeRotate | `angle: number`, `segments?: number` | Rotational sweep |
| Polygon | `points: [number,number][]` | 2D points array |
| Translate | `offset: [number,number,number]` | 3D translation |
| Rotate | `angles: [number,number,number]` | Rotation angles in radians |
| Hull | `children: React.ReactNode` | Convex hull of children |
| Union | `children: React.ReactNode` | Boolean union of children |
| Subtract | `children: React.ReactNode` | Boolean subtraction |## Why?
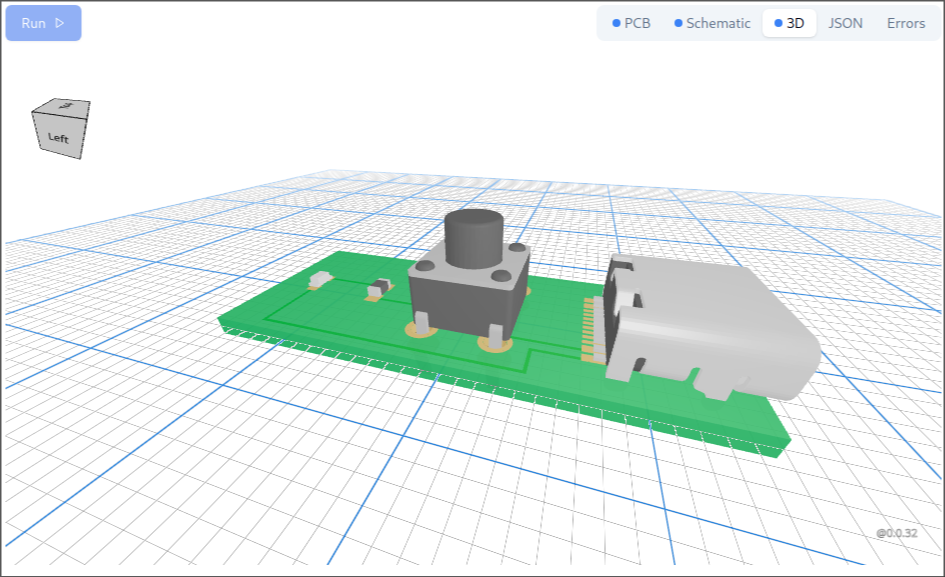JSCAD allows you to create detailed 3D objects using boolean operations. This
is how modern CAD tools create precise 3D models. jscad-fiber is used to
create the 3D electronics library for [tscircuit](https://github.com/tscircuit/tscircuit) called
[jscad-electronics](https://github.com/tscircuit/jscad-electronics)
## Example WrapperThe library includes an `ExampleWrapper` component that provides a convenient way to display both the rendered 3D object and its source code:

```tsx
import { ExampleWrapper } from "jscad-fiber"export default () => (
)
```This wrapper:
- Shows the rendered 3D object
- Provides a "Show Code" button that reveals the source code
- Automatically syntax highlights the code
- Makes examples more interactive and educational## Contributing
See the [`examples` directory](./examples) for more usage examples.
Pull requests welcome! Please check existing issues or create a new one to discuss major changes.