https://github.com/vutoan266/react-images-uploading
The simple images uploader applied Render Props pattern that allows you to fully control UI component and behaviors.
https://github.com/vutoan266/react-images-uploading
image image-upload images react react-images-upload react-images-uploading reactjs typescript upload uploader
Last synced: 24 days ago
JSON representation
The simple images uploader applied Render Props pattern that allows you to fully control UI component and behaviors.
- Host: GitHub
- URL: https://github.com/vutoan266/react-images-uploading
- Owner: vutoan266
- License: mit
- Created: 2019-12-20T10:06:45.000Z (over 5 years ago)
- Default Branch: master
- Last Pushed: 2023-03-05T11:23:49.000Z (about 2 years ago)
- Last Synced: 2024-05-17T12:48:23.882Z (12 months ago)
- Topics: image, image-upload, images, react, react-images-upload, react-images-uploading, reactjs, typescript, upload, uploader
- Language: TypeScript
- Homepage: https://github.com/vutoan266/react-images-uploading
- Size: 3.04 MB
- Stars: 316
- Watchers: 3
- Forks: 51
- Open Issues: 55
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# react-images-uploading
![]()
The simple images uploader applied `Render Props` pattern. (You can read more about this pattern [here](https://reactjs.org/docs/render-props.html)).
This approach allows you to fully control UI component and behaviours.
> See [the intro blog post](https://medium.com/@imvutoan/make-image-upload-in-react-easier-with-react-images-uploading-and-your-ui-983fed029ee2)
[](https://github.com/facebook/jest)  [](https://www.npmjs.com/package/react-images-uploading) [](https://www.npmjs.com/package/react-images-uploading) [](https://github.com/vutoan266/react-images-uploading/blob/master/LICENSE) [](https://github.com/vutoan266/react-images-uploading/pulls) [](https://packagequality.com/#?package=react-images-uploading) [](https://github.com/prettier/prettier)
[](#contributors-)
[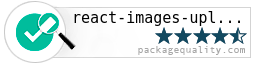](https://packagequality.com/#?package=react-images-uploading)
- [Installation](#installation)
- [Usage](#usage)
- [Props](#props)
- [Note](#note)
- [Exported options](#exported-options)
- [Contributors ✨](#contributors-)## Installation
**npm**
```bash
npm install --save react-images-uploading
```**yarn**
```bash
yarn add react-images-uploading
```## Usage
You can check out the basic demo here:
- Javascript: [https://codesandbox.io/s/react-images-uploading-demo-u0khz](https://codesandbox.io/s/react-images-uploading-demo-u0khz)
- Typescript: [https://codesandbox.io/s/react-images-uploading-demo-typescript-fr2zm](https://codesandbox.io/s/react-images-uploading-demo-typescript-fr2zm)
- Server Side rendering (NextJS): [https://codesandbox.io/s/react-images-uploading-ssr-j1qq2](https://codesandbox.io/s/react-images-uploading-ssr-j1qq2)**Basic**
```tsx
import React from 'react';
import ImageUploading from 'react-images-uploading';export function App() {
const [images, setImages] = React.useState([]);
const maxNumber = 69;const onChange = (imageList, addUpdateIndex) => {
// data for submit
console.log(imageList, addUpdateIndex);
setImages(imageList);
};return (
{({
imageList,
onImageUpload,
onImageRemoveAll,
onImageUpdate,
onImageRemove,
isDragging,
dragProps,
}) => (
// write your building UI
Click or Drop here
Remove all images
{imageList.map((image, index) => (
![]()
onImageUpdate(index)}>Update
onImageRemove(index)}>Remove
))}
)}
);
}
```**Validation**
```ts
...
{({ imageList, onImageUpload, onImageRemoveAll, errors }) => (
errors &&
{errors.maxNumber && Number of selected images exceed maxNumber}
{errors.acceptType && Your selected file type is not allow}
{errors.maxFileSize && Selected file size exceed maxFileSize}
{errors.resolution && Selected file is not match your desired resolution}
)}
...
```**Drag and Drop**
```tsx
...
{({ imageList, dragProps, isDragging }) => (
{isDragging ? "Drop here please" : "Upload space"}
{imageList.map((image, index) => (
![]()
))}
)}
...
```## Props
| parameter | type | options | default | description |
| ----------------- | ----------------------------------- | ----------------------------------------- | ------- | --------------------------------------------------------------------- |
| value | array | | [] | List of images |
| onChange | function | (imageList, addUpdateIndex) => void | | Called when add, update or delete action is called |
| dataURLKey | string | | dataURL | Customized field name that base64 of selected image is assigned to |
| multiple | boolean | | false | Set `true` for multiple chooses |
| maxNumber | number | | 1000 | Number of images user can select if mode = `multiple` |
| onError | function | (errors, files) => void | | Called when it has errors |
| acceptType | array | ['jpg', 'gif', 'png'] | [] | The file extension(s) to upload |
| maxFileSize | number | | | Max image size (Byte) and it is used in validation |
| resolutionType | string | 'absolute' \| 'less' \| 'more' \| 'ratio' | | Using for image validation with provided width & height |
| resolutionWidth | number | > 0 | | |
| resolutionHeight | number | > 0 | | |
| inputProps | React.HTMLProps\ | | | |
| allowNonImageType | boolean | | false | Using for uploading non-image type purpose (E.g. txt, pdf, heic, ...) |### Note
**resolutionType**
| value | description |
| :------- | :----------------------------------------------------------------------- |
| absolute | image's width === resolutionWidth && image's height === resolutionHeight |
| ratio | (resolutionWidth / resolutionHeight) === (image width / image height) |
| less | image's width < resolutionWidth && image's height < resolutionHeight |
| more | image's width > resolutionWidth && image's height > resolutionHeight |## Exported options
| parameter | type | description |
| :--------------- | :---------------------------------------- | :------------------------------------------------------------------ |
| imageList | array | List of images to render. |
| onImageUpload | function | Called when an element is clicks and triggers to open a file dialog |
| onImageRemoveAll | function | Called when removing all images |
| onImageUpdate | (updateIndex: number) => void | Called when updating an image at `updateIndex`. |
| onImageRemove | (deleteIndex: number \| number[]) => void | Called when removing one or list image. |
| errors | object | Exported object of validation |
| dragProps | object | Native element props for drag and drop feature |
| isDragging | boolean | "true" if an image is being dragged |## Contributors ✨
Thanks goes to these wonderful people ([emoji key](https://allcontributors.org/docs/en/emoji-key)):
vutoan266
💻 🤔 📖 🚧 ⚠️ 👀
David Nguyen
💻 🤔 📖 👀
DK
💻 🚇 🤔 👀 📖
Isaac Maseruka
💻
This project follows the [all-contributors](https://github.com/all-contributors/all-contributors) specification. Contributions of any kind welcome!