Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/wang-bin/fvp
Flutter video player plugin for all desktop+mobile platforms. download prebuilt examples from github actions. https://pub.dev/packages/fvp
https://github.com/wang-bin/fvp
d3d11 desktop dolby-vision flutter hdr metal player video
Last synced: 1 day ago
JSON representation
Flutter video player plugin for all desktop+mobile platforms. download prebuilt examples from github actions. https://pub.dev/packages/fvp
- Host: GitHub
- URL: https://github.com/wang-bin/fvp
- Owner: wang-bin
- License: bsd-3-clause
- Created: 2022-09-24T01:34:12.000Z (over 2 years ago)
- Default Branch: master
- Last Pushed: 2024-12-23T04:09:33.000Z (15 days ago)
- Last Synced: 2024-12-29T22:08:01.437Z (9 days ago)
- Topics: d3d11, desktop, dolby-vision, flutter, hdr, metal, player, video
- Language: Dart
- Homepage:
- Size: 558 KB
- Stars: 211
- Watchers: 5
- Forks: 33
- Open Issues: 33
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Funding: .github/FUNDING.yml
- License: LICENSE
Awesome Lists containing this project
README
# FVP
A plugin for official [Flutter Video Player](https://pub.dev/packages/video_player) to support all desktop and mobile platforms, with hardware accelerated decoding and optimal rendering. Based on [libmdk](https://github.com/wang-bin/mdk-sdk). You can also create your own players other than official `video_player` with [backend player api](#backend-player-api)
Prebuilt example can be download from artifacts of [github actions](https://github.com/wang-bin/fvp/actions).
[More examples are here](https://github.com/wang-bin/mdk-examples/tree/master/flutter)
project is create with `flutter create -t plugin --platforms=linux,macos,windows,android,ios -i objc -a java fvp`
## Features
- All platforms: Windows x64(including win7) and arm64, Linux x64 and arm64, macOS, iOS, Android(requires flutter > 3.19 because of minSdk 21).
- You can choose official implementation or this plugin's
- Optimal render api: d3d11 for windows, metal for macOS/iOS, OpenGL for Linux and Android(Impeller support)
- Hardware decoders are enabled by default
- Dolby Vision support on all platforms
- Minimal code change for existing [Video Player](https://pub.dev/packages/video_player) apps
- Support most formats via FFmpeg demuxer and software decoders if not supported by gpu. You can use your own ffmpeg 4.0~7.0(or master branch) by removing bundled ffmpeg dynamic library.
- High performance. Lower cpu, gpu and memory load than libmpv based players.
- Support audio without video
- Small footprint. Only about 10MB size increase per cpu architecture(platform dependent).## How to Use
- Add [fvp](https://pub.dev/packages/fvp) in your pubspec.yaml dependencies: `flutter pub add fvp`. Since flutter 3.27, fvp must be a direct dependency in your app's pubspec.yaml.
- **(Optional)** Add 2 lines in your video_player examples. Without this step, this plugin will be used for video_player unsupported platforms(windows, linux), official implementation will be used otherwise.```dart
import 'package:fvp/fvp.dart' as fvp;fvp.registerWith(); // in main() or anywhere before creating a player. use fvp for all platforms.
```You can also select the platforms to enable fvp implementation
```dart
registerWith(options: {'platforms': ['windows', 'macos', 'linux']}); // only these platforms will use this plugin implementation
```To select [other decoders](https://github.com/wang-bin/mdk-sdk/wiki/Decoders), pass options like this
```dart
fvp.registerWith(options: {
'video.decoders': ['D3D11', 'NVDEC', 'FFmpeg']
//'lowLatency': 1, // optional for network streams
}); // windows
```[The document](https://pub.dev/documentation/fvp/latest/fvp/registerWith.html) lists all options for `registerWith()`
### Error Handling
Errors are usually produced when loading a media.
```dart
_controller.addListener(() {
if (_controller.value.hasError && !_controller.value.isCompleted) {
...
```### Backend Player API
```dart
import 'package:fvp/mdk.dart';
```The plugin implements [VideoPlayerPlatform](https://pub.dev/packages/video_player_platform_interface) via [a thin wrapper](https://github.com/wang-bin/fvp/blob/master/lib/video_player_mdk.dart) on [player.dart](https://github.com/wang-bin/fvp/blob/master/lib/src/player.dart).
Now we also expose this backend player api so you can create your own players easily, and gain more features than official [video_player](https://pub.dev/packages/video_player), for example, play from a given position, loop in a range, decoder selection, media information detail etc. You can also reuse the Player instance without unconditionally create and dispose, changing the `Player.media` is enough.
[This is an example](https://github.com/wang-bin/mdk-examples/blob/master/flutter/simple/lib/multi_textures.dart)### VideoPlayerController Extensions
With this extension, we can leverage mature `video_player` code without rewriting a new one via backend player api, but gain more features, for example `snapshot()`, `record()`, `fastSeekTo()`, `setExternalSubtitle()`.
```dart
import 'package:fvp/fvp.dart' as fvp;fvp.registerWith(); // in main() or anywhere before creating a player. use fvp for all platforms.
// somewhere after controller is initialized
_controller.record('rtmp://127.0.0.1/live/test');
```# Upgrade Dependencies Manually
Upgrading binary dependencies can bring new features and backend bug fixes. For macOS and iOS, in your project dir, run
```bash
pod cache clean mdk
find . -name Podfile.lock -delete
rm -rf {mac,i}os/Pods
```For other platforms, set environment var `FVP_DEPS_LATEST=1` and rebuilt, will upgrade to the latest sdk. If fvp is installed from pub.dev, run `flutter pub cache clean` is another option.
# Design
- Playback control api in dart via ffi
- Manage video renderers in platform specific manners. Receive player ptr via `MethodChannel` to construct player instance and set a renderer target.
- Callbacks and events in C++ are notified by ReceivePort
- Function with a one time callback is async and returns a future# Enable Subtitles
libass is required, and it's added to your app automatically for windows, macOS and android(remove ass.dll, libass.dylib and libass.so from mdk-sdk if you don't need it). For iOS, [download](https://sourceforge.net/projects/mdk-sdk/files/deps/dep.7z/download) and add `ass.framework` to your xcode project. For linux, system libass can be used, you may have to install manually via system package manager.
If required subtitle font is not found in the system(e.g. android), you can add [assets/subfont.ttf](https://github.com/mpv-android/mpv-android/raw/master/app/src/main/assets/subfont.ttf) in pubspec.yaml as the fallback.
# DO NOT use flutter-snap
Flutter can be installed by snap, but it will add some [enviroment vars(`CPLUS_INCLUDE_PATH` and `LIBRARY_PATH`) which may break C++ compiler](https://github.com/canonical/flutter-snap/blob/main/env.sh#L15-L18). It's not recommended to use snap, althrough building for linux is [fixed](https://github.com/wang-bin/fvp/commit/567c68270ba16b95b1198ae58850707ae4ad7b22), but it's not possible for android.# Screenshots
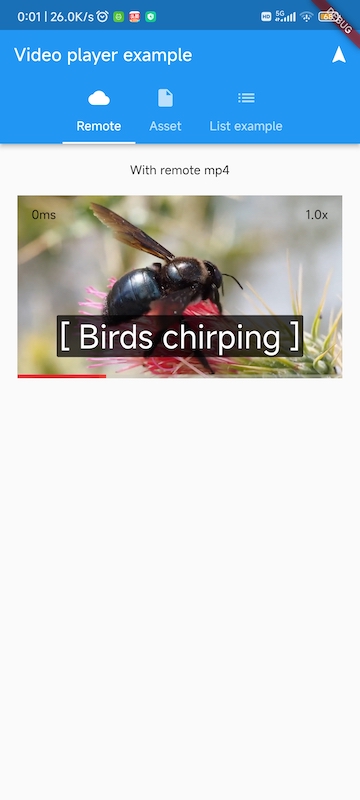
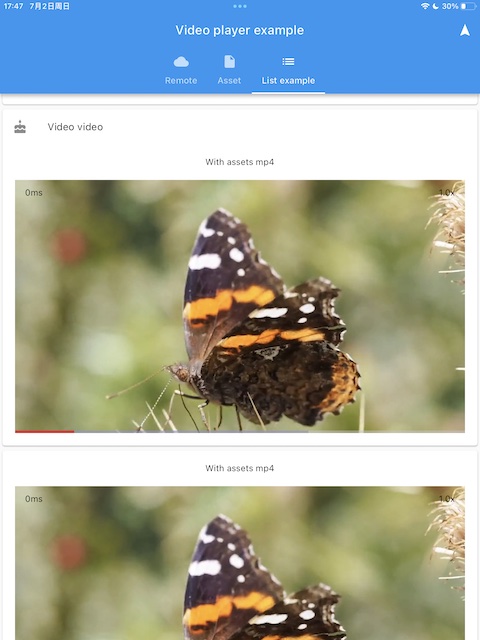
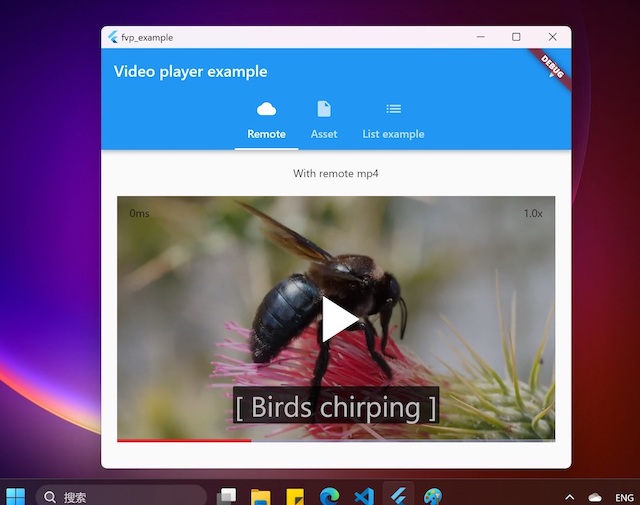
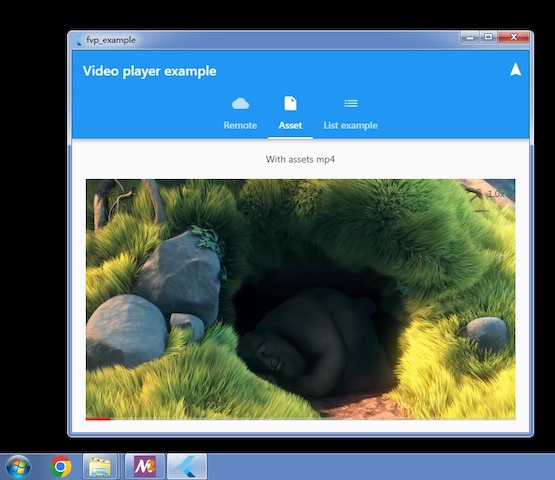
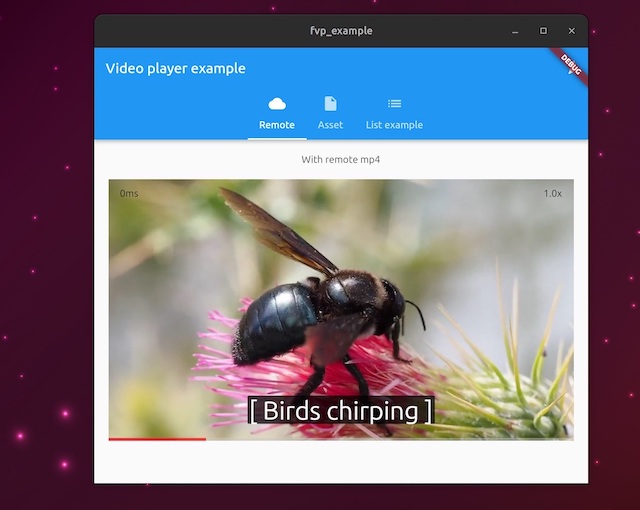
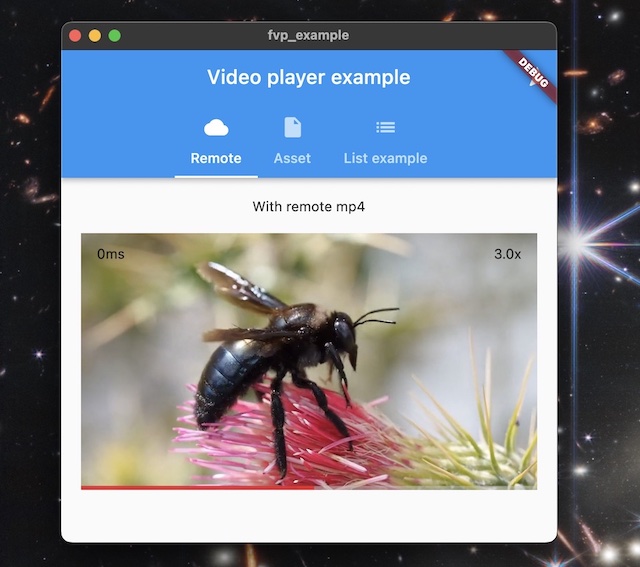