Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/will123195/venti
⚛️ Global State for React
https://github.com/will123195/venti
javascript react state
Last synced: 2 months ago
JSON representation
⚛️ Global State for React
- Host: GitHub
- URL: https://github.com/will123195/venti
- Owner: will123195
- Created: 2019-11-15T01:26:06.000Z (about 5 years ago)
- Default Branch: master
- Last Pushed: 2020-09-21T22:45:55.000Z (over 4 years ago)
- Last Synced: 2024-09-01T15:19:58.416Z (4 months ago)
- Topics: javascript, react, state
- Language: JavaScript
- Homepage:
- Size: 1.17 MB
- Stars: 17
- Watchers: 3
- Forks: 1
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
[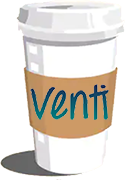](https://github.com/will123195/venti)
**Global State for React**
[](https://travis-ci.org/will123195/venti)
## Install
```
npm i venti
```## Quick Start
#### Get global state
```jsx
import React from 'react'
import { useVenti } from 'venti'export default function Book({ id }) {
const state = useVenti()
const { author, title } = state.get(`books.${id}`, {})
const year = state.get(`books.${id}.year`)
return"{title}" by {author} ({year})
}
```#### Set global state
```js
import { state } from 'venti'state.set('books.1', {
author: 'John Steinbeck',
title: 'Cannery Row',
year: 1945
})
```## API
### `useVenti( [state] )`
- `state` {State} (optional) defaults to singleton state if not provided
- Returns `state` that has been instrumented to update the component when applicable
- See [StateEventer](https://github.com/will123195/state-eventer) for more info### `state.get( path, [defaultValue] )`
- `path` {Array|string} The path to get
- `defaultValue` {*} (optional) The value returned for undefined resolved values
- Returns the resolved value### `state.set( path, value )`
- `path` {Array|string} The path of the property to set
- `value` {*} The value to set### `state.unset( path )`
- `path` {Array|string} The path of the property to unset### `state.update( path, transformFn, [defaultValue] )`
- `path` {Array|string} The path of the property to set
- `transformFn` {Function} transforms the current value to a new value
- `defaultValue` {*} (optional) the default value to pass into the transform function if the existing value at the given path is undefined
```js
state.update('counter', n => n + 1, 0)
```## Advanced Usage
If you don't want to use Venti's singleton state, you can do this:
```jsx
import React from 'react'
import { State, useVenti } from 'venti'const globalState = new State()
const useGlobalState = () => useVenti(globalState)export default function Book({ id }) {
const state = useGlobalState()
const { title, year } = state.get(`books.${id}`)
return{title} ({year})
}
```## Performance Benchmarks
### Color Matrix Benchmark
- Venti: https://will123195.github.io/venti-performance/build/
- Redux: https://will123195.github.io/redux-performance/build/## Examples
- [React State Museum - Packing List App](https://codesandbox.io/s/github/GantMan/ReactStateMuseum/tree/master/React/venti)
## Tests
```
npm test
```## License
MIT