Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/yushulx/flutter_ocr_sdk
Detect machine-readable zones (MRZ) in passports, travel documents, and ID cards
https://github.com/yushulx/flutter_ocr_sdk
dart flutter idcard mrz mrz-scanner ocr passport visa
Last synced: about 2 months ago
JSON representation
Detect machine-readable zones (MRZ) in passports, travel documents, and ID cards
- Host: GitHub
- URL: https://github.com/yushulx/flutter_ocr_sdk
- Owner: yushulx
- License: mit
- Created: 2021-07-21T08:33:51.000Z (over 3 years ago)
- Default Branch: main
- Last Pushed: 2024-09-25T06:54:29.000Z (3 months ago)
- Last Synced: 2024-10-09T08:08:37.341Z (2 months ago)
- Topics: dart, flutter, idcard, mrz, mrz-scanner, ocr, passport, visa
- Language: C++
- Homepage: https://pub.dev/packages/flutter_ocr_sdk
- Size: 49.4 MB
- Stars: 14
- Watchers: 3
- Forks: 6
- Open Issues: 5
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- License: LICENSE
Awesome Lists containing this project
README
# flutter_ocr_sdk
A wrapper for [Dynamsoft OCR SDK](https://www.dynamsoft.com/label-recognition/overview/) with MRZ detection model. It helps developers build Flutter applications to detect machine-readable zones (**MRZ**) in passports, travel documents, and ID cards.
## Try MRZ Detection Example
### Mobile: Android and iOS
```bash
cd example
flutter run
```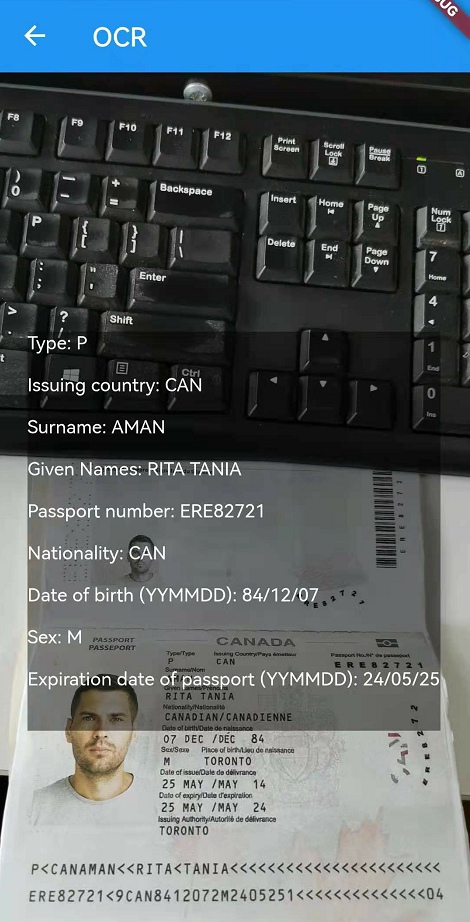
### Web
```bash
cd example
flutter run -d chrome
```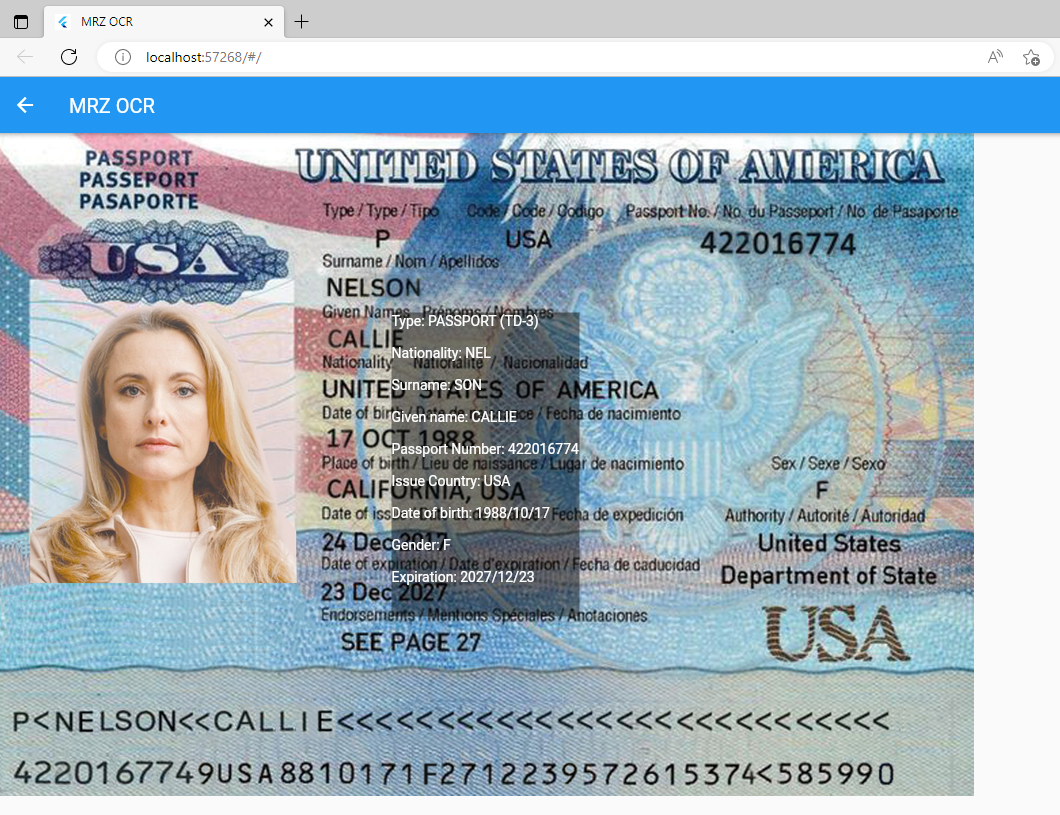
### Desktop: Windows and Linux
```bash
cd example
flutter run -d windows# flutter run -d linux
```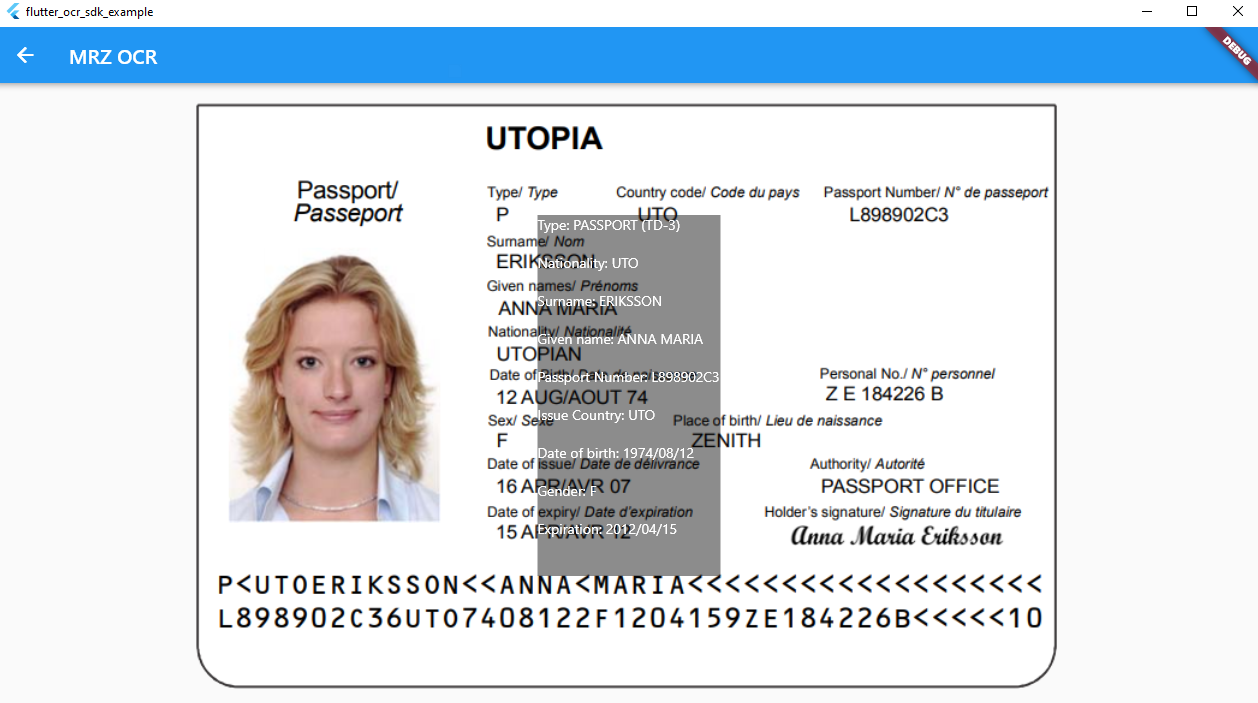
## Supported Platforms
- Android
- Web
- Windows
- Linux
- iOS## Installation
Add `flutter_ocr_sdk` as a dependency in your `pubspec.yaml` file.```yml
dependencies:
...
flutter_ocr_sdk:
```### One More Step for Web
Include the JavaScript library of Dynamsoft Label Recognizer in your `index.html` file:```html
```
## API Compatibility
| Methods | Android | iOS | Windows | Linux | macOS | Web|
| ----------- | ----------- | ----------- | ----------- |----------- |----------- |----------- |
| `Future init(String key)` | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: |:heavy_check_mark: | :heavy_check_mark: |
| `Future loadModel()` | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: |:heavy_check_mark: | :heavy_check_mark: |:heavy_check_mark: |
| `Future>?> recognizeByFile(String filename)` | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: |:heavy_check_mark: | :heavy_check_mark: |:heavy_check_mark: |
| `Future>?> recognizeByBuffer(Uint8List bytes, int width, int height, int stride, int format)` | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: | :heavy_check_mark: |:heavy_check_mark: | :heavy_check_mark: |## Usage
- Initialize the MRZ detector with a [valid license key](https://www.dynamsoft.com/customer/license/trialLicense/?product=dcv&package=cross-platform):```dart
FlutterOcrSdk _mrzDetector = FlutterOcrSdk();
int? ret = await _mrzDetector.init( "DLS2eyJoYW5kc2hha2VDb2RlIjoiMjAwMDAxLTE2NDk4Mjk3OTI2MzUiLCJvcmdhbml6YXRpb25JRCI6IjIwMDAwMSIsInNlc3Npb25QYXNzd29yZCI6IndTcGR6Vm05WDJrcEQ5YUoifQ==");
```
- Load the MRZ detection model:
```dart
await _mrzDetector.loadModel();
```
- Recognize MRZ from an image file:```dart
List>? results = await _mrzDetector.recognizeByFile(photo.path);
```
- Recognize MRZ from an image buffer:```dart
ui.Image image = await decodeImageFromList(fileBytes);ByteData? byteData =
await image.toByteData(format: ui.ImageByteFormat.rawRgba);List>? results = await _mrzDetector.recognizeByBuffer(
byteData.buffer.asUint8List(),
image.width,
image.height,
byteData.lengthInBytes ~/ image.height,
ImagePixelFormat.IPF_ARGB_8888.index);
```
- Parse MRZ information:```dart
String information = '';
if (results != null && results.isNotEmpty) {
for (List area in results) {
if (area.length == 2) {
information =
MRZ.parseTwoLines(area[0].text, area[1].text).toString();
} else if (area.length == 3) {
information = MRZ
.parseThreeLines(area[0].text, area[1].text, area[2].text)
.toString();
}
}
}
```