https://github.com/yushulx/python-mrz-scanner-sdk
Python MRZ scanner SDK built with Dynamsoft Label Recognizer
https://github.com/yushulx/python-mrz-scanner-sdk
cpython idcard machine-readable-zone mrz ocr passport python python-extension python3 visa wheel
Last synced: 3 months ago
JSON representation
Python MRZ scanner SDK built with Dynamsoft Label Recognizer
- Host: GitHub
- URL: https://github.com/yushulx/python-mrz-scanner-sdk
- Owner: yushulx
- License: mit
- Created: 2022-08-05T09:01:07.000Z (almost 3 years ago)
- Default Branch: main
- Last Pushed: 2024-10-17T08:21:21.000Z (8 months ago)
- Last Synced: 2025-02-26T01:16:08.669Z (4 months ago)
- Topics: cpython, idcard, machine-readable-zone, mrz, ocr, passport, python, python-extension, python3, visa, wheel
- Language: C++
- Homepage: https://pypi.org/project/mrz-scanner-sdk/
- Size: 90.3 MB
- Stars: 6
- Watchers: 3
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# Python MRZ Scanner SDK
This project provides a Python-C++ binding for the [Dynamsoft Label Recognizer v2.x](https://www.dynamsoft.com/label-recognition/overview/), allowing developers to build **MRZ (Machine Readable Zone)** scanner applications on both **Windows** and **Linux** platforms using Python.> Note: This project is an unofficial, community-maintained Python wrapper for the Dynamsoft Label Recognizer SDK. For those seeking the most reliable and fully-supported solution, Dynamsoft offers an official Python package. Visit the [Dynamsoft Capture Vision Bundle](https://pypi.org/project/dynamsoft-capture-vision-bundle/) page on PyPI for more details.
## About Dynamsoft Capture Vision Bundle
- Activate the SDK with a [30-day FREE trial license](https://www.dynamsoft.com/customer/license/trialLicense/?product=dcv&package=cross-platform).
- Install the SDK via `pip install dynamsoft-capture-vision-bundle`.### Comparison Table
| Feature | Unofficial Wrapper (Community) | Official Dynamsoft Capture Vision SDK |
| --- | --- | --- |
| Support | Community-driven, best effort | Official support from Dynamsoft |
| Documentation | README only | [Comprehensive Online Documentation](https://www.dynamsoft.com/capture-vision/docs/server/programming/python/?lang=python) |
| API Coverage | Limited | Full API coverage |
|Feature Updates| May lag behind the official SDK | First to receive new features |
| Compatibility | Limited testing across environments| Thoroughly tested across all supported environments|
| OS Support | Windows, Linux | Windows, Linux, **macOS** |## Supported Python Versions
* Python 3.x## Installation
Install the required dependencies:
```bash
pip install mrz opencv-python
```## Command-line Usage
- Scan MRZ from an image file:
```bash
scanmrz -l
```
- Scan MRZ from a webcam:
```bash
scanmrz -u 1 -l
```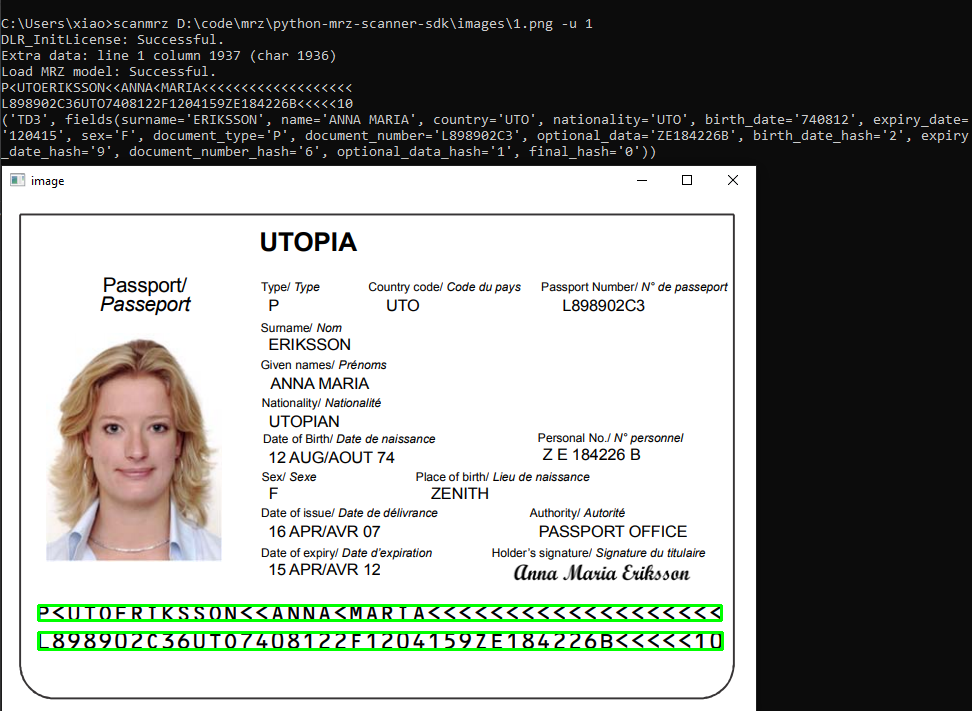
## Quick Start
```python
import mrzscanner
from mrz.checker.td1 import TD1CodeChecker
from mrz.checker.td2 import TD2CodeChecker
from mrz.checker.td3 import TD3CodeChecker
from mrz.checker.mrva import MRVACodeChecker
from mrz.checker.mrvb import MRVBCodeCheckerdef check(lines):
try:
td1_check = TD1CodeChecker(lines)
if bool(td1_check):
return "TD1", td1_check.fields()
except Exception as err:
pass
try:
td2_check = TD2CodeChecker(lines)
if bool(td2_check):
return "TD2", td2_check.fields()
except Exception as err:
pass
try:
td3_check = TD3CodeChecker(lines)
if bool(td3_check):
return "TD3", td3_check.fields()
except Exception as err:
pass
try:
mrva_check = MRVACodeChecker(lines)
if bool(mrva_check):
return "MRVA", mrva_check.fields()
except Exception as err:
pass
try:
mrvb_check = MRVBCodeChecker(lines)
if bool(mrvb_check):
return "MRVB", mrvb_check.fields()
except Exception as err:
pass
return 'No valid MRZ information found'# set license
mrzscanner.initLicense("LICENSE-KEY")# initialize mrz scanner
scanner = mrzscanner.createInstance()# load MRZ model
scanner.loadModel(mrzscanner.load_settings())print('')
# decodeFile()
s = ""
results = scanner.decodeFile("images/1.png")
for result in results:
print(result.text)
s += result.text + '\n'
print('')
print(check(s[:-1]))
print('')
```## API Reference
- `mrzscanner.initLicense('YOUR-LICENSE-KEY')`: Initialize the SDK with your license key.
```python
mrzscanner.initLicense("LICENSE-KEY")
```- `mrzscanner.createInstance()`: Create an instance of the MRZ scanner.
```python
scanner = mrzscanner.createInstance()
```
- `scanner.loadModel()`: Load the MRZ model configuration.
```python
scanner.loadModel(mrzscanner.load_settings())
```
- `decodeFile()`: Recognize MRZ from an image file.```python
results = scanner.decodeFile()
for result in results:
print(result.text)
```
- `decodeMat()`: Recognize MRZ from an OpenCV Mat.
```python
import cv2
image = cv2.imread()
results = scanner.decodeMat(image)
for result in results:
print(result.text)
```
- `addAsyncListener(callback function)`: Register a callback function to receive MRZ recognition results asynchronously.
- `decodeMatAsync()`: Recognize MRZ from OpenCV Mat asynchronously.
```python
def callback(results):
s = ""
for result in results:
print(result.text)
s += result.text + '\n'
print('')
print(check(s[:-1]))
import cv2
image = cv2.imread()
scanner.addAsyncListener(callback)
for i in range (2):
scanner.decodeMatAsync(image)
sleep(1)
```## How to Build the Python MRZ Scanner Extension
- Create a source distribution:
```bash
python setup.py sdist
```- setuptools:
```bash
python setup.py build
python setup.py develop
```
- Build wheel:
```bash
pip wheel . --verbose
# Or
python setup.py bdist_wheel
```