Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/yuyz0112/vue-type-check
a type checker for typescript written Vue components
https://github.com/yuyz0112/vue-type-check
types typescript vetur vue
Last synced: 20 days ago
JSON representation
a type checker for typescript written Vue components
- Host: GitHub
- URL: https://github.com/yuyz0112/vue-type-check
- Owner: Yuyz0112
- Created: 2019-10-02T07:05:37.000Z (about 5 years ago)
- Default Branch: master
- Last Pushed: 2023-01-04T11:55:27.000Z (almost 2 years ago)
- Last Synced: 2024-10-14T21:55:58.937Z (about 1 month ago)
- Topics: types, typescript, vetur, vue
- Language: TypeScript
- Homepage: http://www.myriptide.com/vue-type-check/
- Size: 569 KB
- Stars: 96
- Watchers: 5
- Forks: 19
- Open Issues: 20
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
![]()
# vue-type-check
vue-type-check is a type checker for typescript written Vue components.
It provides both a CLI wrapper and a programmatically API which is easy to integrate with your current workflow.
## Features
- type checking template code.
- type checking script code.## Usage
### CLI
```shell
Install: npm i -g vue-type-checkUsage: vue-type-check or vtc
Options:
--workspace path to your workspace, required
--srcDir path to the folder which contains your Vue components, will fallback to the workspace when not passed
--onlyTemplate whether to check the script code in a single file component
```#### Example
We are going to check a simple Vue component with two type errors:
```vue
{{ msg }}
import Vue from "vue";
export default Vue.extend({
name: "app",
data() {
return {
message: "Hello World!"
};
},
methods: {
printMessage() {
console.log(this.message.toFixed(1));
}
}
});```
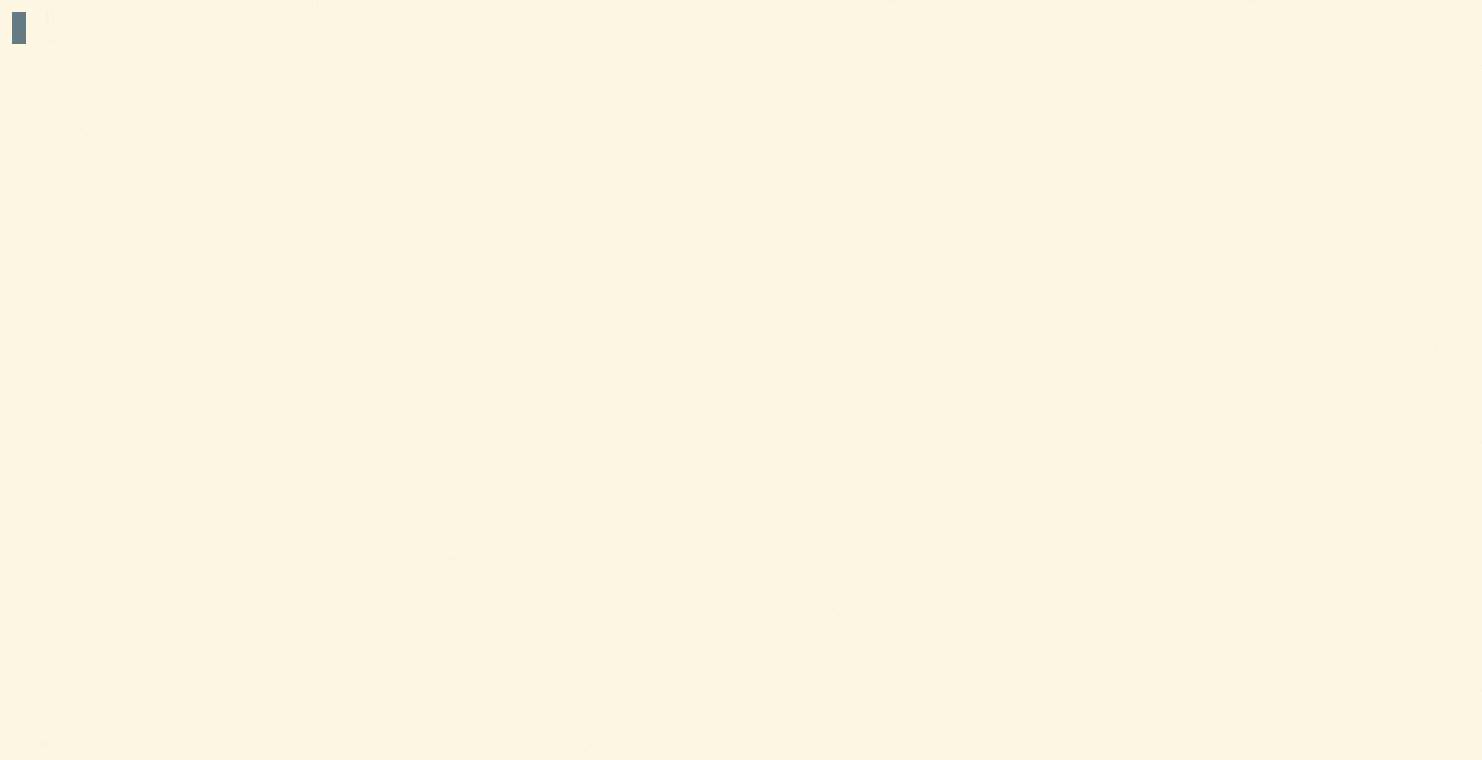
### Programmatical API
```js
const { check } = require("vue-type-check");(async () => {
await check({
workspace: PATH_TO_WORKSPACE,
srcDir: PATH_TO_SRC_DIR,
excludeDir: PATH_TO_EXCLUDE_DIR,
onlyTemplate: TRUE_OR_FALSE,
onlyTypeScript: TRUE_OR_FALSE
});
})();
```## How it works
Currently, the implementation is heavily based on vetur's awesome [interpolation feature](https://vuejs.github.io/vetur/guide/interpolation.html).
If you are interested in the design decisions and the attempts on other approaches, they can be found in [this post](http://www.myriptide.com/vue-type-check/).