https://github.com/zepgram/module-rest
Provide a module to industrialize API REST request with dependency injection using Guzzle library
https://github.com/zepgram/module-rest
Last synced: 2 months ago
JSON representation
Provide a module to industrialize API REST request with dependency injection using Guzzle library
- Host: GitHub
- URL: https://github.com/zepgram/module-rest
- Owner: zepgram
- License: mit
- Created: 2021-11-04T23:33:11.000Z (over 3 years ago)
- Default Branch: master
- Last Pushed: 2024-11-24T21:33:01.000Z (8 months ago)
- Last Synced: 2025-05-07T00:08:49.856Z (2 months ago)
- Language: PHP
- Homepage:
- Size: 52.7 KB
- Stars: 12
- Watchers: 3
- Forks: 4
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
- awesome-magento2 - Rest Client - Technical Magento 2 module providing simple development pattern, configurations and optimizations to make REST API requests toward external services based on Guzzle Client. (Open Source Extensions / Development Utilities)
README
# Zepgram Rest
## Overview
Zepgram Rest is a technical module designed to streamline the development of REST API integrations in Magento 2 projects.
Utilizing the Guzzle HTTP client for dependency injection, this module offers a robust set of features aimed at reducing
boilerplate code, improving performance, and enhancing debugging capabilities. By centralizing REST API interactions and
leveraging Magento's built-in systems, Zepgram Rest simplifies the implementation process for developers.## Features
Zepgram Rest provides several key features to aid Magento developers in creating and managing RESTful services:
- Avoid Code Duplication: Minimize repetitive code with a straightforward setup in di.xml. Implement your REST API integrations with just one class creation, streamlining the development process.
- Centralized Configuration: Manage all your REST web services configurations in one place, ensuring consistency and ease of maintenance.
- Built-in Registry and Cache: Take advantage of Magento's native cache mechanisms and dedicated registry to boost your API's performance and security. This feature helps in efficiently managing data retrieval and storage, reducing the load on your server.
- Generic Logger: Debugging is made effortless with an inclusive logging system. Enable the debug mode to log detailed information about your API calls, including parameters, requests, and responses, facilitating easier troubleshooting.
- Data Serialization: Declare whether your requests and results should be JSON serialized or not. This flexibility prevents the need for multiple serializer implementations, accommodating various API requirements with ease.## Installation
```
composer require zepgram/module-rest
bin/magento module:enable Zepgram_Rest
bin/magento setup:upgrade
```## Guideline with ApiPool
1. Create a RequestAdapter class for your service extending abstract class `Zepgram\Rest\Model\RequestAdapter`,
this class represent your service contract adapter:
- **public const SERVICE_ENDPOINT**: define the service endpoint
- **dispatch(DataObject $rawData)**: initialize data that you will adapt to request the web service
- **getBody()**: implement body request
- **getHeaders()**: implement headers
- **getUri()**: implement uri endpoint (used to handle dynamic values)
- **getCacheKey()**: implement cache key for your specific request (you must define a unique key)
1. Create a system.xml, and a config.xml with a dedicated **configName**:
- **section**: `rest_api`
- **group_id**: `$configName`
- **fields**:
- `base_uri`
- `timeout`
- `is_debug`
- `cache_ttl`
1. Declare your service in di.xml by implementing `Zepgram\Rest\Service\ApiProvider` as VirtualClass, you can configure
it by following the [ApiProviderConfig](#configuration)
1. Declare your RequestAdapter and ApiProvider in `Zepgram\Rest\Service\ApiPoolInterface`:
- Add a new item in `apiProviders[]`:
- The **key** is your custom RequestAdapter full namespace
- The **value** is your ApiProvider as a VirtualClass
1. Inject ApiPoolInterface in the class that will consume your API and use `$this->apiPool->execute(RequestAdapter::class, $rawData)` where:
- **RequestAdapter::class** represents the request adapter declared in `apiProviders[]`
- **$rawData** is an array of dynamic data that will be dispatch in `dispatch()` method## Basic guideline implementation
Instead of declaring your class in `Zepgram\Rest\Service\ApiPoolInterface` you can also directly inject
your ApiProvider in a dedicated class:
```xml
Zepgram\Sales\Rest\FoxtrotOrderRequestAdapter
foxtrot
CustomApiProvider
```
```php
orderRepository->get($orderId);
// send request
$result = $this->apiProvider->execute(['order' => $order]);
return $result;
}
}
```## Configuration
### Store config
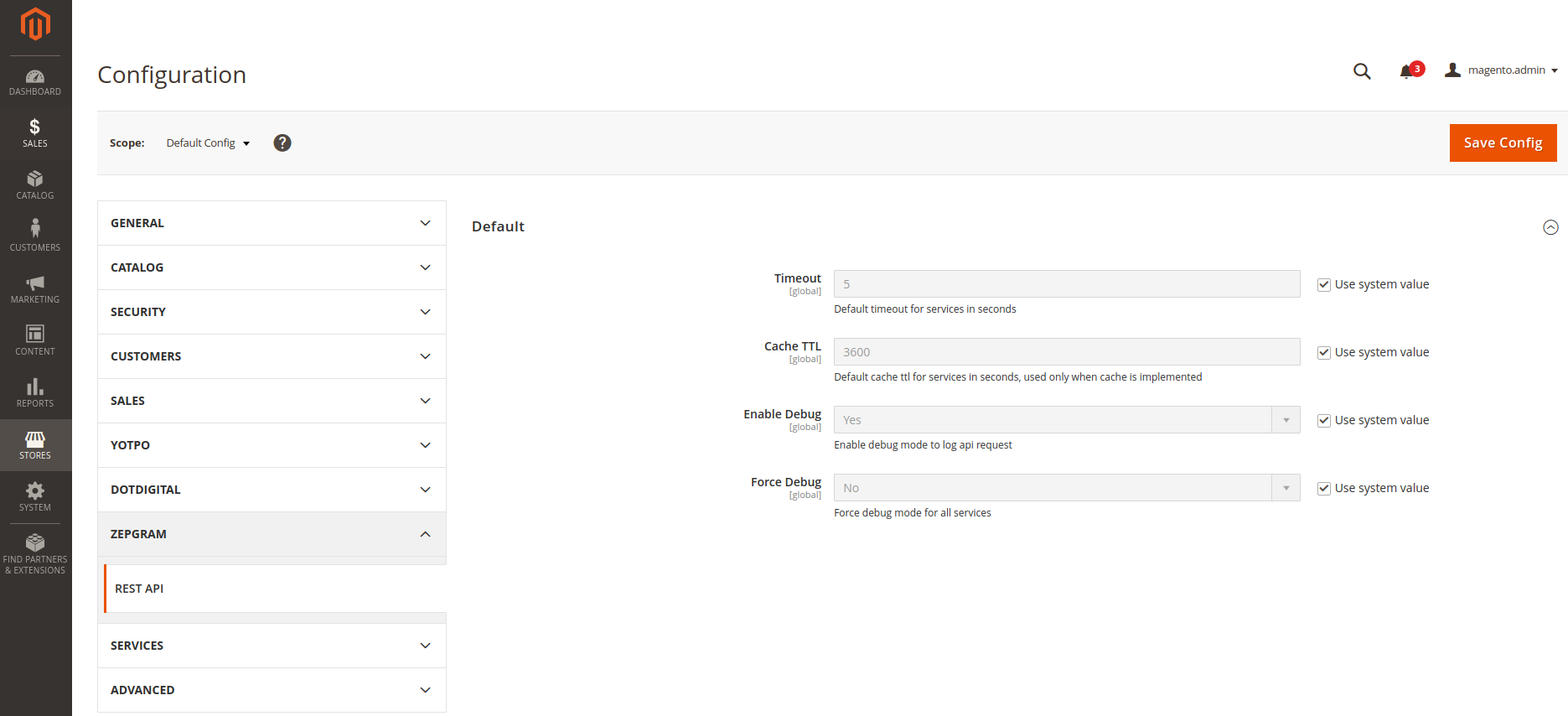
If you do not declare specific configuration, the request will fall back on default configuration.
To override the default config, you must follow this system config pattern: `rest_api/%configName%/base_uri`### XML config
You can configure your service with `Zepgram\Rest\Service\ApiProvider` by creating a
VirtualClass and customize its injections for your needs by following the below configuration:| Variable name | Type | Default value | Is Optional | Description |
|:--------------:|:-------:|:--------------:|:-----------:|:------------------------------------------------:|
| configName | string | default | no | Value to retrieve group id from system config |
| requestAdapter | object | RequestAdapter | no | Adapter class to build and customize the request |
| validator | object | null | yes | Validate the service contract |
| method | string | GET | yes | Request method |
| isJsonRequest | boolean | true | yes | Parse request array to json |
| isJsonResponse | boolean | true | yes | Parse response string to array |
| isVerify | boolean | true | yes | Enable SSL certificate verification |## Implementation
Here is a simple implementation example with a service called **Foxtrot** using the order object as rawData:
**FoxtrotOrderRequestAdapter.php**
```php
order = $rawData->getOrder();
}/**
* {@inheritDoc}
*/
public function getBody(): array
{
return [
'orderId' => $this->order->getEntityId(),
'customer' => $this->order->getCustomerEmail(),
'orderTotal' => $this->order->getGrandTotal(),
'version' => '1.0',
];
}/**
* {@inheritDoc}
*/
public function getCacheKey(): ?string
{
return $this->order->getEntityId();
}
}
```**system.xml**
```xml
Foxtrot
Base URI
Timeout
Cache TTL
Enable Debug
Magento\Config\Model\Config\Source\Yesno
```
**config.xml**
```xml
https://foxtrot.service.io
30
7200
1
```
**di.xml**
```xml
Zepgram\Sales\Rest\FoxtrotOrderRequestAdapter
foxtrot
FoxtrotOrderApiProvider
```
**OrderDataExample.php**
```php
orderRepository->get($orderId);
// send request
$result = $this->apiPool->execute(FoxtrotOrderRequestAdapter::class, ['order' => $order]);
// handle result
$order->setFoxtrotData($result);
$this->orderRepository->save($order);
} catch (InternalException $e) {
$context['context_error'] = 'Magento request is wrong, foxtrot order service could not handle it'
// service rejected request for business reason: do something (log, throw, errorMessage..)
throw MyAwesomeBusinessException(__('Bad request error'), $e);
} catch (ExternalException $e) {
$context['context_error'] = 'We could not reach foxtrot order service'
// service is unavailable due to technical reason: do something (log, throw, errorMessage..)
$this->logger->error($e, $context);
throw MyAwesomeTechnicalException(__('Foxtrot server error'), $e);
}
}
}
```
## Log & Sensitive DataThis module includes a built-in Monolog Processor: [SensitiveDataProcessor.php](Logger/SensitiveDataProcessor.php)
Depending on your `MAGE_MODE` environment variable, data will be automatically obfuscated if log key contains those keys:
- `password`
- `username`
- `user`
- `token`
- `key`
- `secret`
- `hash`
- `hmac`
- `sha`
- `sign`
- `authorization`
- `jwt`
- `access`
- `auth`
- `sso`
- `passphrase`
- `ssh`
- `pin`
- `cvv`
- `ccv`
- `cvc`
- `card`This class can be customized with `di.xml` by:
- Activating the processor with parameter `$isEnabled`, otherwise it will only be enabled if `MAGE_MODE === 'production'`
- Adding custom keys by using `$sensitiveKeys` parameter
- Overriding the default keys by using `$overrideSensitiveKeys` parameter
- Modify the placeholder for sensitive data by setting `$redactionPlaceholder`## Issues & Improvements
If you encountered an issue during installation or with usage, please report it on this github repository.
If you have good ideas to improve this module, feel free to contribute.