https://github.com/zweifisch/sieve
a simple yet efficient cache
https://github.com/zweifisch/sieve
Last synced: 4 months ago
JSON representation
a simple yet efficient cache
- Host: GitHub
- URL: https://github.com/zweifisch/sieve
- Owner: zweifisch
- License: mit
- Created: 2024-02-15T12:11:28.000Z (over 1 year ago)
- Default Branch: main
- Last Pushed: 2024-02-19T12:43:30.000Z (about 1 year ago)
- Last Synced: 2025-01-08T11:52:23.589Z (4 months ago)
- Language: TypeScript
- Size: 862 KB
- Stars: 6
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# sieve
a simple yet efficient cache, [original introduction](https://cachemon.github.io/SIEVE-website/blog/2023/12/17/sieve-is-simpler-than-lru/)
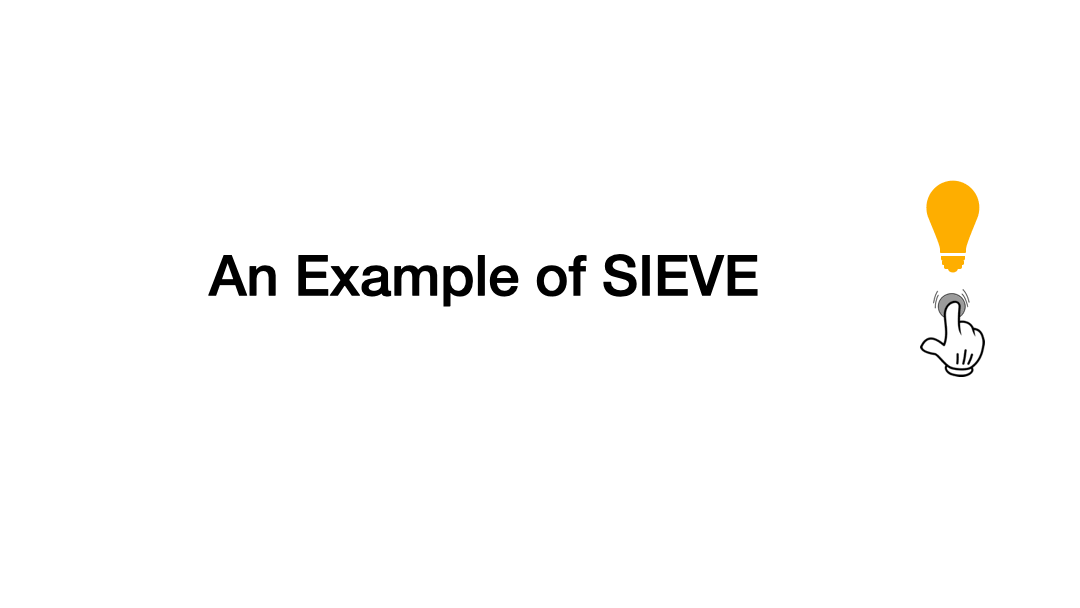
## Usage
for Node.js, install via npm: `npm install @zf/sieve`
```typescript
import { SieveCache, LRUCache, LFUCache } from '@zf/sieve'const cache = new SieveCache(3 /* capacity */)
cache.set('key', 'value')
cache.get('key')
```for Deno
```typescript
import { SieveCache, LRUCache, LFUCache } from "https://deno.land/x/sieve/mod.ts"
```## Benchmark
[Benchmark](/benchmark.ts) reading 1 million normally distributed items through a cache with a capacity of 100 compared with the [LRU](https://deno.land/x/[email protected]) package
showing SIEVE is more performant, while the cache hit/miss ratio is about the same:
It turned out that the LRU package's implementation is not very efficient, so I wrote my own [LRU](/lru.ts), and it is actually better than SIEVE:

Anyway, the cache hit/miss ratio is of much greater importance, and it is determined by the data distribution.
Added [LFU](/lfu.ts), which has the best hit rate for normally distributed data:

## Dev
```sh
deno test
``````sh
deno run --allow-all build_npm.ts 1.0.0
```