Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/uraimo/SG90Servo.swift
Swift library for the SG90 Servo Motor, adaptable for other servos (9g ES08A, SM-S4303R, S3003, etc...).
https://github.com/uraimo/SG90Servo.swift
9g raspberrypi servo-motor sg90 swift
Last synced: 3 months ago
JSON representation
Swift library for the SG90 Servo Motor, adaptable for other servos (9g ES08A, SM-S4303R, S3003, etc...).
- Host: GitHub
- URL: https://github.com/uraimo/SG90Servo.swift
- Owner: uraimo
- License: other
- Created: 2017-03-08T21:19:33.000Z (over 7 years ago)
- Default Branch: master
- Last Pushed: 2019-09-04T07:24:58.000Z (almost 5 years ago)
- Last Synced: 2024-03-20T18:20:48.574Z (3 months ago)
- Topics: 9g, raspberrypi, servo-motor, sg90, swift
- Language: Swift
- Homepage:
- Size: 51.8 KB
- Stars: 11
- Watchers: 4
- Forks: 3
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Lists
- awesome-embedded-swift - SG90Servo.swift - Swift library for the SG90 Servo Motor, adaptable for other servos (9g ES08A, SM-S4303R, S3003, etc...). (Modules / Networking, IoT, Bus Protocols, …)
README
# SG90Servo.swift
*A Swift library for the SG90 servo motor, that can be adapted to work with other servos (9g ES08A, SM-S4303R, S3003, ...).*
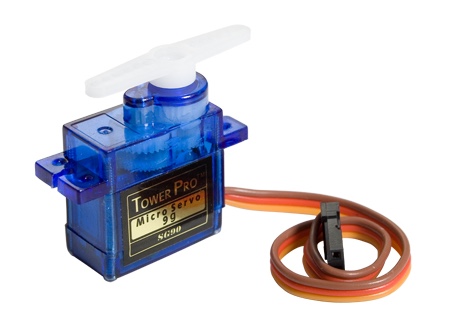
# Summary
This simple library can control an SG90 servo motor with a PWM, that has a 20ms period and duty cycle that represent these positions:
* 1ms = 5%: _-90°, left position_
* 1.5ms = 7.5%: _-90°, middle position_
* 2ms = 10%: _+90°, right position_Your servo could have slightly different settings (1-2% difference), play around with the values in the `Position` enum (expressed in percentage) to find the right duty cycles for your servo.
## Supported Boards
Every board supported by [SwiftyGPIO](https://github.com/uraimo/SwiftyGPIO) with the PWM feature, right now only RaspberryPis. And you'll need Swift 3.x, check out the SwiftyGPIO readme for more info on where to find it.
The example below uses a RaspberryPi 2 board but you can easily modify the example to use one the the other supported boards, a full working demo projects for the RaspberryPi2 is available in the `Examples` directory.
## Usage
The first thing we need to do is to obtain an instance of `PWMOutput` from SwiftyGPIO and use it to initialize the `SG90Servo` object:
```swift
import SwiftyGPIO
import SG90Servolet pwms = SwiftyGPIO.hardwarePWMs(for:.RaspberryPi2)!
let pwm = (pwms[0]?[.P18])!let s1 = SG90Servo(pwm)
s1.enable()
s1.move(to: .left)
sleep(1)
s1.move(to: .middle)
sleep(1)
s1.move(to: .right)
sleep(1)s1.disable()
```
## Installation
Please refer to the [SwiftyGPIO](https://github.com/uraimo/SwiftyGPIO) readme for Swift installation instructions.
Once your board runs Swift, if your version support the Swift Package Manager, you can simply add this library as a dependency of your project and compile with `swift build`:
```
// swift-tools-version:4.0
import PackageDescriptionlet package = Package(
name: "TestServo",
dependencies: [
.package(url: "https://github.com/uraimo/SwiftyGPIO.git", from: "1.0.0"),
.package(url: "https://github.com/uraimo/SG90Servo.swift.git",from: "3.0.0")
],
targets: [
.target(name: "TestServo", dependencies: ["SwiftyGPIO","SG90Servo"]),
]
)
```The directory `Examples` contains sample projects that uses SPM, compile it and run the sample with `sudo ./.build/debug/TestServo`.
As everything interacting with GPIOs, if you are not already root or member of a specific gpio group, you will need to run that binary with `sudo ./main`.