https://github.com/sjwhitworth/golearn
Machine Learning for Go
https://github.com/sjwhitworth/golearn
Last synced: 4 days ago
JSON representation
Machine Learning for Go
- Host: GitHub
- URL: https://github.com/sjwhitworth/golearn
- Owner: sjwhitworth
- License: mit
- Created: 2013-12-26T13:06:14.000Z (over 11 years ago)
- Default Branch: master
- Last Pushed: 2024-01-15T13:55:44.000Z (about 1 year ago)
- Last Synced: 2025-04-02T00:09:21.351Z (11 days ago)
- Language: Go
- Homepage:
- Size: 26.4 MB
- Stars: 9,363
- Watchers: 430
- Forks: 1,190
- Open Issues: 87
-
Metadata Files:
- Readme: README.md
- License: LICENSE.md
Awesome Lists containing this project
- awesome-go - GoLearn - General Machine Learning library for Go. (Machine Learning / Search and Analytic Databases)
- awesome-golang-ai - golearn
- zero-alloc-awesome-go - GoLearn - General Machine Learning library for Go. (Machine Learning / Search and Analytic Databases)
- go-awesome - GoLearn - an out-of-the-box machine learning library (Open source library / Machine Learning)
- awesome-go - GoLearn - General Machine Learning library for Go. Stars:`9.4K`. (Machine Learning / Search and Analytic Databases)
- awesome-list - GoLearn - Machine Learning for Go. (Machine Learning Framework / General Purpose Framework)
- awesome-go - golearn - Machine Learning for Go - ★ 6080 (Machine Learning)
- awesome-ai-ml-dl - GoLearn - Machine Learning library for Go
- awesome-go-extra - golearn - 12-26T13:06:14Z|2022-08-04T21:27:27Z| (Machine Learning / Advanced Console UIs)
- awesome-go-zh - GoLearn
- StarryDivineSky - sjwhitworth/golearn - learn的Fit/Predict接口,支持多种评估方法和数据处理工具,方便用户进行模型选择和性能评估。该项目正在积极开发中,欢迎用户反馈和参与。 (其他_机器学习与深度学习)
README
GoLearn
=======
[](https://godoc.org/github.com/sjwhitworth/golearn)
[](https://travis-ci.org/sjwhitworth/golearn)
[](https://codecov.io/gh/sjwhitworth/golearn)[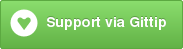](https://www.gittip.com/sjwhitworth/)
GoLearn is a 'batteries included' machine learning library for Go. **Simplicity**, paired with customisability, is the goal.
We are in active development, and would love comments from users out in the wild. Drop us a line on Twitter.twitter: [@golearn_ml](http://www.twitter.com/golearn_ml)
Install
=======See [here](https://github.com/sjwhitworth/golearn/wiki/Installation) for installation instructions.
Getting Started
=======Data are loaded in as Instances. You can then perform matrix like operations on them, and pass them to estimators.
GoLearn implements the scikit-learn interface of Fit/Predict, so you can easily swap out estimators for trial and error.
GoLearn also includes helper functions for data, like cross validation, and train and test splitting.```go
package mainimport (
"fmt""github.com/sjwhitworth/golearn/base"
"github.com/sjwhitworth/golearn/evaluation"
"github.com/sjwhitworth/golearn/knn"
)func main() {
// Load in a dataset, with headers. Header attributes will be stored.
// Think of instances as a Data Frame structure in R or Pandas.
// You can also create instances from scratch.
rawData, err := base.ParseCSVToInstances("datasets/iris.csv", true)
if err != nil {
panic(err)
}// Print a pleasant summary of your data.
fmt.Println(rawData)//Initialises a new KNN classifier
cls := knn.NewKnnClassifier("euclidean", "linear", 2)//Do a training-test split
trainData, testData := base.InstancesTrainTestSplit(rawData, 0.50)
cls.Fit(trainData)//Calculates the Euclidean distance and returns the most popular label
predictions, err := cls.Predict(testData)
if err != nil {
panic(err)
}// Prints precision/recall metrics
confusionMat, err := evaluation.GetConfusionMatrix(testData, predictions)
if err != nil {
panic(fmt.Sprintf("Unable to get confusion matrix: %s", err.Error()))
}
fmt.Println(evaluation.GetSummary(confusionMat))
}
``````
Iris-virginica 28 2 56 0.9333 0.9333 0.9333
Iris-setosa 29 0 59 1.0000 1.0000 1.0000
Iris-versicolor 27 2 57 0.9310 0.9310 0.9310
Overall accuracy: 0.9545
```Examples
========GoLearn comes with practical examples. Dive in and see what is going on.
```bash
cd $GOPATH/src/github.com/sjwhitworth/golearn/examples/knnclassifier
go run knnclassifier_iris.go
```
```bash
cd $GOPATH/src/github.com/sjwhitworth/golearn/examples/instances
go run instances.go
```
```bash
cd $GOPATH/src/github.com/sjwhitworth/golearn/examples/trees
go run trees.go
```Docs
====* [English](https://github.com/sjwhitworth/golearn/wiki)
* [中文文档(简体)](doc/zh_CN/Home.md)
* [中文文档(繁体)](doc/zh_TW/Home.md)Join the team
=============Please send me a mail at [email protected]